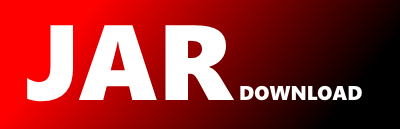
org.refcodes.web.HttpInterceptable Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-web Show documentation
Show all versions of refcodes-web Show documentation
This artifact provides web (HTTP) related definitions and types being used
by REFCODES.ORG web related functionality and artifacts.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany and licensed
// under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/LICENSE-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.web;
/**
* The {@link HttpInterceptable} provides base functionality for working with
* {@link HttpInterceptor} instances using the {@link PreHttpInterceptable} as
* well as the {@link PostHttpInterceptable} definitions.
*
* @param The type of {@link HttpInterceptor} to be managed.
*/
public interface HttpInterceptable> extends PreHttpInterceptable, PostHttpInterceptable {
/**
* Tests whether the given {@link HttpInterceptor} instance has been added.
*
* @param aInterceptor The {@link HttpInterceptor} instance for which to
* test if it has been added.
*
* @return True in case {@link #hasPreHttpInterceptor(PreHttpInterceptor)}
* as well {@link #hasPostHttpInterceptor(PostHttpInterceptor)}
* return true,
*/
default boolean hasHttpInterceptor( I aInterceptor ) {
return hasPreHttpInterceptor( aInterceptor ) && hasPostHttpInterceptor( aInterceptor );
}
/**
* Adds the given {@link HttpInterceptor} instance. The
* {@link HttpInterceptor} instance itself acts as the handle which is used
* when removing the given {@link HttpInterceptor} instance later.
*
* @param aInterceptor The {@link HttpInterceptor} instance which is to be
* added.
*
* @return True in case {@link #addPreHttpInterceptor(PreHttpInterceptor)}
* or {@link #addPostHttpInterceptor(PostHttpInterceptor)} return
* true.
*/
default boolean addHttpInterceptor( I aInterceptor ) {
return addPreHttpInterceptor( aInterceptor ) | addPostHttpInterceptor( aInterceptor );
}
/**
* Removes the {@link HttpInterceptor} instance. In case the
* {@link HttpInterceptor} instance has not been added before, then false is
* returned.
*
* @param aInterceptor The {@link HttpInterceptor} instance which is to be
* removed.
*
* @return True in case
* {@link #removePreHttpInterceptor(PreHttpInterceptor)} or
* {@link #removePostHttpInterceptor(PostHttpInterceptor)} return
* true.
*/
default boolean removeHttpInterceptor( I aInterceptor ) {
return removePreHttpInterceptor( aInterceptor ) | removePostHttpInterceptor( aInterceptor );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy