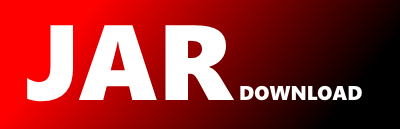
org.refcodes.web.HttpServerContextBuilder Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of refcodes-web Show documentation
Show all versions of refcodes-web Show documentation
This artifact provides web (HTTP) related definitions and types being used
by REFCODES.ORG web related functionality and artifacts.
The newest version!
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.web;
import org.refcodes.data.Scheme;
import org.refcodes.io.MaxConnectionsAccessor.MaxConnectionsBuilder;
import org.refcodes.io.MaxConnectionsAccessor.MaxConnectionsProperty;
import org.refcodes.mixin.PortAccessor.PortBuilder;
import org.refcodes.mixin.PortAccessor.PortProperty;
import org.refcodes.security.KeyStoreDescriptor;
import org.refcodes.security.KeyStoreDescriptorAccessor.KeyStoreDescriptorBuilder;
import org.refcodes.security.KeyStoreDescriptorAccessor.KeyStoreDescriptorProperty;
import org.refcodes.web.SchemeAccessor.SchemeBuilder;
import org.refcodes.web.SchemeAccessor.SchemeProperty;
/**
* The {@link HttpServerContextBuilder} implements the {@link HttpServerContext}
* interface extending it with builder functionality..
*/
public class HttpServerContextBuilder implements HttpServerContext, SchemeProperty, SchemeBuilder, KeyStoreDescriptorProperty, KeyStoreDescriptorBuilder, PortProperty, PortBuilder, MaxConnectionsProperty, MaxConnectionsBuilder {
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
public static final Scheme DEFAULT_SCHEME = Scheme.HTTP;
public static final int DEFAULT_PORT = 8080;
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private KeyStoreDescriptor _keyStoreDescriptor = null;
private int _maxConnections = -1;
private int _port = DEFAULT_PORT;
private Scheme _scheme = DEFAULT_SCHEME;
private String _protocol = null;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Constructs a {@link HttpClientContext} with a default scheme
* {@link Scheme#HTTP} for port 8080.
*/
public HttpServerContextBuilder() {}
/**
* Constructs a {@link HttpClientContext} with a default scheme.
*
* @param aPort The TCP port to be used.
*/
public HttpServerContextBuilder( int aPort ) {
_port = aPort;
}
/**
* Constructs a {@link HttpClientContext} with a default scheme.
*
* @param aScheme The {@link Scheme} (HTTP or HTTPS) to be used.
* @param aPort The TCP port to be used.
*/
public HttpServerContextBuilder( Scheme aScheme, int aPort ) {
setScheme( aScheme );
_port = aPort;
}
/**
* Constructs a {@link HttpClientContext} with a default scheme.
*
* @param aProtocol The protocol such as the {@link TransportLayerProtocol}
* @param aPort The TCP port to be used.
*/
public HttpServerContextBuilder( String aProtocol, int aPort ) {
setProtocol( aProtocol );
_port = aPort;
}
/**
* Constructs a {@link HttpClientContext} with the given data.
*
* @param aScheme The {@link Scheme} (HTTP or HTTPS) to be used.
* @param aPort The TCP port to be used.
* @param aKeyStoreDescriptor The descriptor describing the keystore to be
* used.
* @param aMaxConnections The maximum allowed open connections.
*/
public HttpServerContextBuilder( Scheme aScheme, int aPort, KeyStoreDescriptor aKeyStoreDescriptor, int aMaxConnections ) {
setScheme( aScheme );
_port = aPort;
_keyStoreDescriptor = aKeyStoreDescriptor;
_maxConnections = aMaxConnections;
}
/**
* Constructs a {@link HttpClientContext} with the given data.
*
* @param aProtocol The protocol such as the {@link TransportLayerProtocol}
* elements.
* @param aPort The TCP port to be used.
* @param aKeyStoreDescriptor The descriptor describing the keystore to be
* used.
* @param aMaxConnections The maximum allowed open connections.
*/
public HttpServerContextBuilder( String aProtocol, int aPort, KeyStoreDescriptor aKeyStoreDescriptor, int aMaxConnections ) {
setProtocol( aProtocol );
_port = aPort;
_keyStoreDescriptor = aKeyStoreDescriptor;
_maxConnections = aMaxConnections;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public KeyStoreDescriptor getKeyStoreDescriptor() {
return _keyStoreDescriptor;
}
/**
* {@inheritDoc}
*/
@Override
public int getMaxConnections() {
return _maxConnections;
}
/**
* {@inheritDoc}
*/
@Override
public int getPort() {
return _port;
}
/**
* {@inheritDoc}
*/
@Override
public Scheme getScheme() {
return _scheme;
}
/**
* {@inheritDoc}
*/
@Override
public void setKeyStoreDescriptor( KeyStoreDescriptor aKeyStoreDescriptor ) {
_keyStoreDescriptor = aKeyStoreDescriptor;
}
/**
* {@inheritDoc}
*/
@Override
public void setMaxConnections( int aMaxConnections ) {
_maxConnections = aMaxConnections;
}
/**
* {@inheritDoc}
*/
@Override
public void setPort( int aPort ) {
_port = aPort;
}
/**
* {@inheritDoc}
*/
@Override
public void setScheme( Scheme aScheme ) {
_scheme = aScheme;
_protocol = null;
}
/**
* {@inheritDoc}
*/
@Override
public String toProtocol() {
return _scheme != null ? _scheme.toProtocol() : _protocol;
}
/**
* {@inheritDoc}
*/
@Override
public void setProtocol( String aProtocol ) {
final Scheme theScheme = Scheme.fromProtocol( aProtocol );
if ( theScheme != null ) {
_scheme = theScheme;
_protocol = null;
}
else {
_protocol = aProtocol;
_scheme = null;
}
}
/**
* {@inheritDoc}
*/
@Override
public HttpServerContextBuilder withKeyStoreDescriptor( KeyStoreDescriptor aKeyStoreDescriptor ) {
setKeyStoreDescriptor( aKeyStoreDescriptor );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public HttpServerContextBuilder withMaxConnections( int aMaxConnections ) {
setMaxConnections( aMaxConnections );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public HttpServerContextBuilder withPort( int aPort ) {
setPort( aPort );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public HttpServerContextBuilder withProtocol( String aProtocol ) {
setProtocol( aProtocol );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public HttpServerContextBuilder withScheme( Scheme aScheme ) {
setScheme( aScheme );
return this;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy