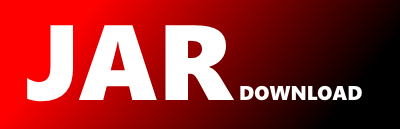
org.refcodes.web.HttpServerResponse Maven / Gradle / Ivy
package org.refcodes.web;
import java.util.HashMap;
import java.util.Map;
import org.refcodes.exception.MarshalException;
import org.refcodes.mixin.Dumpable;
import org.refcodes.textual.VerboseTextBuilder;
import org.refcodes.web.HttpBodyAccessor.HttpBodyProvider;
import org.refcodes.web.HttpStatusCodeAccessor.HttpStatusCodeBuilder;
import org.refcodes.web.HttpStatusCodeAccessor.HttpStatusCodeProperty;
/**
* Defines a {@link HttpServerResponse} as produced by the server.
*/
public class HttpServerResponse extends AbstractHttpResponse implements HttpStatusCodeProperty, HttpStatusCodeBuilder, HttpBodyProvider, Dumpable {
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
protected MediaTypeFactoryLookup _mediaTypeFactoryLookup;
private Object _response = null;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Instantiates a new http server response impl.
*
* @param aMediaTypeFactoryLookup the media type factory lookup
*/
public HttpServerResponse( MediaTypeFactoryLookup aMediaTypeFactoryLookup ) {
_mediaTypeFactoryLookup = aMediaTypeFactoryLookup;
}
/**
* Instantiates a new http server response impl.
*
* @param aHttpStatusCode the http status code
* @param aMediaTypeFactoryLookup the media type factory lookup
*/
public HttpServerResponse( HttpStatusCode aHttpStatusCode, MediaTypeFactoryLookup aMediaTypeFactoryLookup ) {
super( aHttpStatusCode );
_mediaTypeFactoryLookup = aMediaTypeFactoryLookup;
}
/**
* Instantiates a new http server response impl.
*
* @param aResponseHeaderFields the response Header-Fields
* @param aMediaTypeFactoryLookup the media type factory lookup
*/
public HttpServerResponse( ResponseHeaderFields aResponseHeaderFields, MediaTypeFactoryLookup aMediaTypeFactoryLookup ) {
super( aResponseHeaderFields );
_mediaTypeFactoryLookup = aMediaTypeFactoryLookup;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public HttpServerResponse withHttpStatusCode( HttpStatusCode aStatusCode ) {
setHttpStatusCode( aStatusCode );
return this;
}
/**
* Sets the response for the response property.
*
* @param the generic type
* @param aResponse The response to be stored by the response property.
*
* @return The builder for applying multiple build operations.
*/
public HttpServerResponse withResponse( RES aResponse ) {
setResponse( aResponse );
return this;
}
/**
* Retrieves the response from the response property.
*
* @param the generic type
*
* @return The response stored by the response property.
*/
@SuppressWarnings("unchecked")
public RES getResponse() {
return (RES) _response;
}
/**
* Sets the response for the response property. The
* {@link HttpServerResponse#setResponse(Object)} supports the
* {@link HttpBodyMap} to marshal an {@link HttpBodyMap} into an HTTP
* Request-Body.
*
* @param the generic type
* @param aResponse The response to be stored by the response property.
*/
public void setResponse( RES aResponse ) {
_response = aResponse;
}
/**
* {@inheritDoc}
*/
@Override
public void setHttpStatusCode( HttpStatusCode aStatusCode ) {
_httpStatusCode = aStatusCode;
}
/**
* {@inheritDoc}
*/
@Override
public String toHttpBody() throws BadResponseException {
if ( _response != null ) {
MediaTypeFactory theFactory = null;
final ContentType theContentType = getHeaderFields().getContentType();
String theCharset = null;
if ( theContentType == null ) {
throw new BadResponseException( "No (known) Media-Type provided in response's Header-Field <" + HeaderField.CONTENT_TYPE.getName() + ">: " + new VerboseTextBuilder().withElements( getHeaderFields().get( HeaderField.CONTENT_TYPE ) ) );
}
theFactory = _mediaTypeFactoryLookup.toMediaTypeFactory( theContentType.getMediaType() );
theCharset = theContentType.getCharsetParametrer();
if ( theFactory == null ) {
throw new BadResponseException( "No Media-Type factory found (added) for Media-Type <" + theContentType + "> (raw requested Media-Type is <" + getHeaderFields().get( HeaderField.CONTENT_TYPE ) + ">)" );
}
Map theProperties = null;
if ( theCharset != null ) {
theProperties = new HashMap<>();
theProperties.put( MediaTypeParameter.CHARSET.getName(), theCharset );
}
try {
// HttpBodyMap support |-->
Object theResponse = _response;
if ( _response instanceof HttpBodyMap ) {
theResponse = ( (HttpBodyMap) _response ).toDataStructure();
}
// HttpBodyMap support <--|
return theFactory.toMarshaled( theResponse, theProperties );
}
catch ( MarshalException e ) {
throw new BadResponseException( "Unable to marshal response <" + getResponse() + ">.", e );
}
}
return null;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return getClass().getName() + " [_httpStatusCode=" + _httpStatusCode + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy