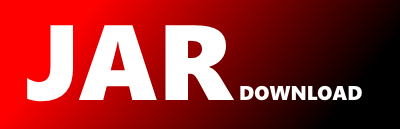
org.refcodes.web.OauthField Maven / Gradle / Ivy
/////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// =============================================================================
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// =============================================================================
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// together with the GPL linking exception applied; as being applied by the GNU
// Classpath ("http://www.gnu.org/software/classpath/license.html")
// =============================================================================
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// =============================================================================
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.web;
import org.refcodes.data.Delimiter;
import org.refcodes.mixin.NameAccessor;
import org.refcodes.mixin.PathAccessor;
/**
* The Enum OauthField.
*/
public enum OauthField implements PathAccessor, HeaderFieldAccessor, NameAccessor {
/**
* OAuth scopes let you specify exactly how your application needs to access
* a user's resource. Scope is specified on either the authorization or
* token endpoints using the parameter scope. Scope is expressed as a set of
* case-sensitive and space-delimited strings. The authorization server may
* override the scope request, in this case it must include scope in its
* response to inform a client of their actual scope. When a scope is not
* specified, the server may either fallback to a well-documented default,
* or fail the request. "... OPTIONAL, if identical to the scope requested
* by the client; otherwise, REQUIRED. The scope of the access token as
* described by Section 3.3. ...", "... OPTIONAL. The scope of the access
* request as described by Section 3.3 ..."
* (https://tools.ietf.org/html/rfc6749)
*/
SCOPE(HeaderField.SCOPE),
/**
* "... RECOMMENDED. The lifetime in seconds of the access token. For
* example, the value "3600" denotes that the access token will expire in
* one hour from the time the response was generated. If omitted, the
* authorization server SHOULD provide the expiration time via other means
* or document the default value ..." (https://tools.ietf.org/html/rfc6749)
*/
EXPIRES_IN(HeaderField.EXPIRES_IN),
/**
* "... OPTIONAL. The refresh token, which can be used to obtain new access
* tokens using the same authorization grant as described in Section 6. ..."
* (https://tools.ietf.org/html/rfc6749)
*/
REFRESH_TOKEN(HeaderField.REFRESH_TOKEN),
/**
* Token type, see also {@link TokenType}. "... REQUIRED. The type of the
* token issued as described in Section 7.1. Value is case insensitive ..."
* (https://tools.ietf.org/html/rfc6749)
*/
TOKEN_TYPE(HeaderField.TOKEN_TYPE),
/**
* "... REQUIRED. The access token issued by the authorization server ..."
* (https://tools.ietf.org/html/rfc6749)
*/
ACCESS_TOKEN(HeaderField.ACCESS_TOKEN),
/**
* "... REQUIRED. The client identifier issued to the client during the
* registration process described by Section 2.2. ..."
* (https://tools.ietf.org/html/rfc6749)
*/
CLIENT_ID(HeaderField.CLIENT_ID),
/**
* "... REQUIRED. The client secret. The client MAY omit the parameter if
* the client secret is an empty string ..."
* (https://tools.ietf.org/html/rfc6749)
*/
CLIENT_SECRET(HeaderField.CLIENT_SECRET),
/**
* Must be set to {@link GrantType#AUTHORIZATION_CODE}. "... grant_type
* REQUIRED. Value MUST be set to "authorization_code" ..."
* (https://tools.ietf.org/html/rfc6749)
*/
GRANT_TYPE(HeaderField.GRANT_TYPE),
/**
* "... REQUIRED, if the "redirect_uri" parameter was included in the
* authorization request as described in Section 4.1.1, and their values
* MUST be identical ..." (https://tools.ietf.org/html/rfc6749)
*/
REDIRECT_URI(HeaderField.REDIRECT_URI),
/**
* "... REQUIRED. The resource owner username ..."
* (https://tools.ietf.org/html/rfc6749)
*/
USER_NAME(HeaderField.USERNAME),
/**
* "... REQUIRED. The resource owner password ..."
* (https://tools.ietf.org/html/rfc6749)
*/
PASSWORD(HeaderField.PASSWORD),
/**
* Probably legacy, encountered upon using KeyCloak.
*/
SESSION_STATE(HeaderField.SESSION_STATE),
/**
* Probably legacy, encountered upon using KeyCloak.
*/
REFRESH_EXPIRES_IN(HeaderField.REFRESH_EXPIRES_IN),
/**
* Probably legacy, encountered upon using KeyCloak.
*/
NOT_BEFORE_POLICY(HeaderField.NOT_BEFORE_POLICY);
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private HeaderField _field;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
private OauthField( HeaderField aField ) {
_field = aField;
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public HeaderField getHeaderField() {
return _field;
}
/**
* {@inheritDoc}
*/
@Override
public String getPath() {
return Delimiter.PATH.getChar() + _field.getName();
}
/**
* Returns the field's name, be it a {@link HeaderFields} or a
* {@link FormFields} entry. {@inheritDoc}
*/
@Override
public String getName() {
return _field.getName();
}
/**
* Resolves the {@link OauthField} from the {@link HttpBodyMap} path.
*
* @param aPath The path for which to retrieve the {@link OauthField}.
*
* @return The {@link OauthField} or null if none was determined.
*/
public static OauthField fromPath( String aPath ) {
if ( aPath != null ) {
if ( aPath.length() > 0 && aPath.charAt( 0 ) != Delimiter.PATH.getChar() ) {
aPath = Delimiter.PATH.getChar() + aPath;
}
for ( OauthField eToken : values() ) {
if ( eToken.getPath().equals( aPath ) ) {
return eToken;
}
}
}
return null;
}
/**
* Resolves the {@link OauthField} from the {@link HeaderField}.
*
* @param aHeaderField The {@link HeaderField} for which to retrieve the
* {@link OauthField}.
*
* @return The {@link OauthField} or null if none was determined.
*/
public static OauthField fromHeaderField( HeaderField aHeaderField ) {
if ( aHeaderField != null ) {
for ( OauthField eToken : values() ) {
if ( eToken.getHeaderField() == aHeaderField ) {
return eToken;
}
}
}
return null;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy