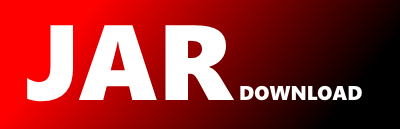
org.refcodes.web.OauthToken Maven / Gradle / Ivy
// /////////////////////////////////////////////////////////////////////////////
// REFCODES.ORG
// /////////////////////////////////////////////////////////////////////////////
// This code is copyright (c) by Siegfried Steiner, Munich, Germany, distributed
// on an "AS IS" BASIS WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, and licen-
// sed under the following (see "http://en.wikipedia.org/wiki/Multi-licensing")
// licenses:
// -----------------------------------------------------------------------------
// GNU General Public License, v3.0 ("http://www.gnu.org/licenses/gpl-3.0.html")
// -----------------------------------------------------------------------------
// Apache License, v2.0 ("http://www.apache.org/licenses/TEXT-2.0")
// -----------------------------------------------------------------------------
// Please contact the copyright holding author(s) of the software artifacts in
// question for licensing issues not being covered by the above listed licenses,
// also regarding commercial licensing models or regarding the compatibility
// with other open source licenses.
// /////////////////////////////////////////////////////////////////////////////
package org.refcodes.web;
import org.refcodes.mixin.Disposable;
import org.refcodes.mixin.ValidAccessor;
/**
* An OAuth-Token contains all relevant information to access a protected
* resource and refresh the the access token. "If the request for an access
* token is valid, the authorization server needs to generate an access token
* (and optional refresh token) and return these to the client, typically along
* with some additional properties about the authorization."
*
* HTTP/1.1 200 OK
* Content-Type: application/json
* Cache-Control: no-store
* Pragma: no-cache
*
* {
* "access_token":"MTQ0NjJkZmQ5OTM2NDE1ZTZjNGZmZjI3",
* "token_type":"bearer",
* "expires_in":3600,
* "refresh_token":"IwOGYzYTlmM2YxOTQ5MGE3YmNmMDFkNTVk",
* "scope":"create"
* }
*
See
* "https://www.oauth.com/oauth2-servers/access-tokens/access-token-response"
*/
public class OauthToken implements ValidAccessor, Disposable {
// /////////////////////////////////////////////////////////////////////////
// CONSTANTS:
// /////////////////////////////////////////////////////////////////////////
private static final String PREFIX_TOKEN_PATH = "token";
// /////////////////////////////////////////////////////////////////////////
// VARIABLES:
// /////////////////////////////////////////////////////////////////////////
private long _creationTimeMillis;
protected String _accessToken;
protected String _refreshToken;
protected String _tokenType;
protected Integer _expiresIn;
protected Integer _refreshExpiresIn;
protected String _scope;
protected String _notBeforePolicy;
protected String _sessionState;
protected GrantType _grantType;
protected String _customGrant;
protected boolean _isDisposed;
// /////////////////////////////////////////////////////////////////////////
// CONSTRUCTORS:
// /////////////////////////////////////////////////////////////////////////
/**
* Creates a new instance of the {@link OauthToken} with the current time
* being stored for determining validity of the access token via
* {@link #isValid()} as of {@link #getExpiresIn()}.
*
* @param aOauthToken The OAuth data to use.
*/
public OauthToken( OauthToken aOauthToken ) {
this( aOauthToken.getAccessToken(), aOauthToken.getRefreshToken(), aOauthToken.getTokenType(), aOauthToken.getGrantType(), aOauthToken._customGrant, aOauthToken.getNotBeforePolicy(), aOauthToken.getExpiresIn(), aOauthToken.getRefreshExpiresIn(), aOauthToken.getScope(), aOauthToken.getSessionState() );
}
/**
* Creates a new instance of the {@link OauthToken} with the current time
* being stored for determining validity of the access token via
* {@link #isValid()} as of {@link #getExpiresIn()}.
*
* @param aAccessToken The access token
* @param aRefreshToken The refresh token
* @param aTokenType The token type
* @param aExpiresIn The expires-time in seconds
* @param aScope The scope
*/
public OauthToken( String aAccessToken, String aRefreshToken, String aTokenType, Integer aExpiresIn, String aScope ) {
this( aAccessToken, aRefreshToken, aTokenType, null, null, null, aExpiresIn, null, aScope, null );
}
/**
* Creates a new instance of the {@link OauthToken} with the current time
* being stored for determining validity of the access token via
* {@link #isValid()} as of {@link #getExpiresIn()}.
*
* @param aAccessToken The access token
* @param aRefreshToken The refresh token
* @param aTokenType The token type
* @param aNotBeforePolicy The not-before-policy.
* @param aExpiresIn The expires-time in seconds
* @param aRefreshExpiresIn The refresh's expires-time in seconds
* @param aScope The scope
* @param aSessionState The session state.
*/
public OauthToken( String aAccessToken, String aRefreshToken, String aTokenType, String aNotBeforePolicy, Integer aExpiresIn, Integer aRefreshExpiresIn, String aScope, String aSessionState ) {
this( aAccessToken, aRefreshToken, aTokenType, null, null, aNotBeforePolicy, aExpiresIn, aRefreshExpiresIn, aScope, aSessionState );
}
/**
* Creates a new instance of the {@link OauthToken} with the current time
* being stored for determining validity of the access token via
* {@link #isValid()} as of {@link #getExpiresIn()}.
*
* @param aAccessToken The access token
* @param aRefreshToken The refresh token
* @param aTokenType The token type
* @param aGrantType The {@link GrantType} used for this token.
* @param aNotBeforePolicy The not-before-policy.
* @param aExpiresIn The expires-time in seconds
* @param aRefreshExpiresIn The refresh's expires-time in seconds
* @param aScope The scope
* @param aSessionState The session state.
*/
public OauthToken( String aAccessToken, String aRefreshToken, String aTokenType, GrantType aGrantType, String aNotBeforePolicy, Integer aExpiresIn, Integer aRefreshExpiresIn, String aScope, String aSessionState ) {
this( aAccessToken, aRefreshToken, aTokenType, aGrantType, null, aNotBeforePolicy, aExpiresIn, aRefreshExpiresIn, aScope, aSessionState );
}
/**
* Creates a new instance of the {@link OauthToken} with the current time
* being stored for determining validity of the access token via
* {@link #isValid()} as of {@link #getExpiresIn()}.
*
* @param aAccessToken The access token
* @param aRefreshToken The refresh token
* @param aTokenType The token type
* @param aGrantType The {@link GrantType} used for this token.
* @param aNotBeforePolicy The not-before-policy.
* @param aExpiresIn The expires-time in seconds
* @param aRefreshExpiresIn The refresh's expires-time in seconds
* @param aScope The scope
* @param aSessionState The session state.
*/
public OauthToken( String aAccessToken, String aRefreshToken, String aTokenType, String aGrantType, String aNotBeforePolicy, Integer aExpiresIn, Integer aRefreshExpiresIn, String aScope, String aSessionState ) {
this( aAccessToken, aRefreshToken, aTokenType, null, aGrantType, aNotBeforePolicy, aExpiresIn, aRefreshExpiresIn, aScope, aSessionState );
}
/**
* Creates a new instance of the {@link OauthToken} with the current time
* being stored for determining validity of the access token via
* {@link #isValid()} as of {@link #getExpiresIn()}.
*
* @param aAccessToken The access token
* @param aRefreshToken The refresh token
* @param aTokenType The token type
* @param aGrantType The {@link GrantType} used for this token.
* @param aCustomGrant The custom grant in case there is none such grant in
* the {@link GrantType} enumeration.
* @param aNotBeforePolicy The not-before-policy.
* @param aExpiresIn The expires-time in seconds
* @param aRefreshExpiresIn The refresh's expires-time in seconds
* @param aScope The scope
* @param aSessionState The session state.
*/
public OauthToken( String aAccessToken, String aRefreshToken, String aTokenType, GrantType aGrantType, String aCustomGrant, String aNotBeforePolicy, Integer aExpiresIn, Integer aRefreshExpiresIn, String aScope, String aSessionState ) {
_creationTimeMillis = System.currentTimeMillis();
_accessToken = aAccessToken;
_refreshToken = aRefreshToken;
_tokenType = aTokenType;
_notBeforePolicy = aNotBeforePolicy;
_expiresIn = aExpiresIn;
_refreshExpiresIn = aRefreshExpiresIn;
_scope = aScope;
_sessionState = aSessionState;
_grantType = aGrantType;
if ( _grantType == null ) {
_grantType = GrantType.fromName( aCustomGrant );
if ( _grantType == null ) {
_customGrant = aCustomGrant;
}
}
}
/**
* Creates a new instance of the {@link OauthToken} with the current time
* being stored for determining validity of the access token via
* {@link #isValid()} as of {@link #getExpiresIn()}.
*
* @param aOauthToken The {@link HttpBodyMap} containing the
* {@link OauthToken} data.
*/
public OauthToken( HttpBodyMap aOauthToken ) {
updateToken( aOauthToken );
}
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public void dispose() {
_isDisposed = true;
_accessToken = null;
_expiresIn = null;
_refreshToken = null;
_scope = null;
_tokenType = null;
_sessionState = null;
_refreshExpiresIn = null;
_notBeforePolicy = null;
}
/**
* Returns the access token. "The access token string as issued by the
* authorization server." See
* "https://www.oauth.com/oauth2-servers/access-tokens/access-token-response"
*
* @return the access token.
*/
public String getAccessToken() {
return _accessToken;
}
/**
* Returns the refresh token. "The access token string as issued by the
* authorization server." See
* "https://www.oauth.com/oauth2-servers/access-tokens/access-token-response"
*
* @return the refresh token.
*/
public String getRefreshToken() {
return _refreshToken;
}
/**
* Returns the OAuth scope. "If the scope the user granted is identical to
* the scope the app requested, this parameter is optional. If the granted
* scope is different from the requested scope, such as if the user modified
* the scope, then this parameter is required." See
* "https://www.oauth.com/oauth2-servers/access-tokens/access-token-response"
*
* @return the OAuth scope.
*/
public String getScope() {
return _scope;
}
/**
* Returns the token type. "The type of token this is, typically just the
* string bearer" See
* "https://www.oauth.com/oauth2-servers/access-tokens/access-token-response"
*
* @return the token type.
*/
public String getTokenType() {
return _tokenType;
}
/**
* Returns the {@link GrantType} used by this token.
*
* @return the according {@link GrantType}.
*/
public GrantType getGrantType() {
return _grantType;
}
/**
* Returns the time in seconds till the access token
* ({@link #getAccessToken()}) expires. "If the access token expires, the
* server should reply with the duration of time the access token is granted
* for." See
* "https://www.oauth.com/oauth2-servers/access-tokens/access-token-response"
*
* @return the Expires-In time in seconds
*/
public Integer getExpiresIn() {
return _expiresIn;
}
/**
* Returns the not-before-policy.
*
* @return the not-before-policy.
*/
public String getNotBeforePolicy() {
return _notBeforePolicy;
}
/**
* Returns the session state.
*
* @return the session state.
*/
public String getSessionState() {
return _sessionState;
}
/**
* Returns the time in seconds till the refresh token
* ({@link #getRefreshToken()}) expires.
*
* @return the Expires-In time in seconds
*/
public Integer getRefreshExpiresIn() {
return _refreshExpiresIn;
}
/**
* {@inheritDoc}
*/
@Override
public boolean isValid() {
if ( _isDisposed ) {
return false;
}
if ( _expiresIn == null ) {
return true;
}
return ( _creationTimeMillis + ( _expiresIn * 1000 ) > System.currentTimeMillis() );
}
// /////////////////////////////////////////////////////////////////////////
// HOOKS:
// /////////////////////////////////////////////////////////////////////////
/**
* Sets (updates) the internal fields with the data from the
* {@link HttpBodyMap} as of the {@link OauthField} definitions. Only fields
* present in the provided {@link HttpBodyMap} are updated, fields not
* provided are kept as were.
*
* @param aOauthToken The {@link HttpBodyMap} from which to gather the data.
*/
protected void updateToken( HttpBodyMap aOauthToken ) {
_creationTimeMillis = System.currentTimeMillis();
if ( aOauthToken.containsKey( OauthField.ACCESS_TOKEN.getPath() ) ) {
_accessToken = aOauthToken.get( OauthField.ACCESS_TOKEN.getPath() );
}
if ( aOauthToken.containsKey( OauthField.REFRESH_TOKEN.getPath() ) ) {
_refreshToken = aOauthToken.get( OauthField.REFRESH_TOKEN.getPath() );
}
if ( aOauthToken.containsKey( OauthField.TOKEN_TYPE.getPath() ) ) {
_tokenType = aOauthToken.get( OauthField.TOKEN_TYPE.getPath() );
}
if ( aOauthToken.containsKey( OauthField.NOT_BEFORE_POLICY.getPath() ) ) {
_notBeforePolicy = aOauthToken.get( OauthField.NOT_BEFORE_POLICY.getPath() );
}
if ( aOauthToken.containsKey( OauthField.EXPIRES_IN.getPath() ) ) {
_expiresIn = aOauthToken.getInt( OauthField.EXPIRES_IN.getPath() );
}
if ( aOauthToken.containsKey( OauthField.REFRESH_EXPIRES_IN.getPath() ) ) {
_refreshExpiresIn = aOauthToken.getInt( OauthField.REFRESH_EXPIRES_IN.getPath() );
}
if ( aOauthToken.containsKey( OauthField.SCOPE.getPath() ) ) {
_scope = aOauthToken.get( OauthField.SCOPE.getPath() );
}
if ( aOauthToken.containsKey( OauthField.SESSION_STATE.getPath() ) ) {
_sessionState = aOauthToken.get( OauthField.SESSION_STATE.getPath() );
}
if ( aOauthToken.containsKey( OauthField.GRANT_TYPE.getPath() ) ) {
_grantType = GrantType.fromName( aOauthToken.get( OauthField.GRANT_TYPE.getPath() ) );
}
if ( aOauthToken.containsKey( PREFIX_TOKEN_PATH, OauthField.ACCESS_TOKEN.getPath() ) && ( _accessToken == null || _accessToken.isEmpty() ) ) {
_accessToken = aOauthToken.get( PREFIX_TOKEN_PATH, OauthField.ACCESS_TOKEN.getPath() );
}
if ( aOauthToken.containsKey( PREFIX_TOKEN_PATH, OauthField.REFRESH_TOKEN.getPath() ) && ( _refreshToken == null || _refreshToken.isEmpty() ) ) {
_refreshToken = aOauthToken.get( PREFIX_TOKEN_PATH, OauthField.REFRESH_TOKEN.getPath() );
}
if ( aOauthToken.containsKey( PREFIX_TOKEN_PATH, OauthField.TOKEN_TYPE.getPath() ) && ( _tokenType == null || _tokenType.isEmpty() ) ) {
_tokenType = aOauthToken.get( PREFIX_TOKEN_PATH, OauthField.TOKEN_TYPE.getPath() );
}
if ( aOauthToken.containsKey( PREFIX_TOKEN_PATH, OauthField.NOT_BEFORE_POLICY.getPath() ) && ( _notBeforePolicy == null || _notBeforePolicy.isEmpty() ) ) {
_notBeforePolicy = aOauthToken.get( PREFIX_TOKEN_PATH, OauthField.NOT_BEFORE_POLICY.getPath() );
}
if ( aOauthToken.containsKey( PREFIX_TOKEN_PATH, OauthField.EXPIRES_IN.getPath() ) && ( _expiresIn == null ) ) {
_expiresIn = aOauthToken.getInt( PREFIX_TOKEN_PATH, OauthField.EXPIRES_IN.getPath() );
}
if ( aOauthToken.containsKey( PREFIX_TOKEN_PATH, OauthField.REFRESH_EXPIRES_IN.getPath() ) && ( _refreshExpiresIn == null ) ) {
_refreshExpiresIn = aOauthToken.getInt( PREFIX_TOKEN_PATH, OauthField.REFRESH_EXPIRES_IN.getPath() );
}
if ( aOauthToken.containsKey( PREFIX_TOKEN_PATH, OauthField.SCOPE.getPath() ) && ( _scope == null || _scope.isEmpty() ) ) {
_scope = aOauthToken.get( PREFIX_TOKEN_PATH, OauthField.SCOPE.getPath() );
}
if ( aOauthToken.containsKey( PREFIX_TOKEN_PATH, OauthField.SESSION_STATE.getPath() ) && ( _sessionState == null || _sessionState.isEmpty() ) ) {
_sessionState = aOauthToken.get( PREFIX_TOKEN_PATH, OauthField.SESSION_STATE.getPath() );
}
if ( aOauthToken.containsKey( PREFIX_TOKEN_PATH, OauthField.GRANT_TYPE.getPath() ) && ( _grantType == null ) ) {
_grantType = GrantType.fromName( aOauthToken.get( PREFIX_TOKEN_PATH, OauthField.GRANT_TYPE.getPath() ) );
}
if ( _grantType == null ) {
if ( aOauthToken.containsKey( OauthField.GRANT_TYPE.getPath() ) ) {
_customGrant = aOauthToken.get( OauthField.GRANT_TYPE.getPath() );
}
if ( _customGrant == null ) {
if ( aOauthToken.containsKey( PREFIX_TOKEN_PATH, OauthField.GRANT_TYPE.getPath() ) ) {
_customGrant = aOauthToken.get( PREFIX_TOKEN_PATH, OauthField.GRANT_TYPE.getPath() );
}
}
}
}
@Override
public String toString() {
return getClass().getSimpleName() + " [creationTimeMillis=" + _creationTimeMillis + ", tokenType=" + _tokenType + ", expiresIn=" + _expiresIn + ", refreshExpiresIn=" + _refreshExpiresIn + ", scope=" + _scope + ", notBeforePolicy=" + _notBeforePolicy + ", sessionState=" + _sessionState + ", grantType=" + ( _grantType != null ? _grantType.getValue() : _customGrant ) + "]";
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy