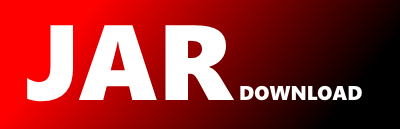
org.refcodes.web.RequestCookie Maven / Gradle / Ivy
package org.refcodes.web;
import java.io.UnsupportedEncodingException;
import java.net.URLDecoder;
import java.net.URLEncoder;
import org.refcodes.data.Delimiter;
import org.refcodes.data.Encoding;
import org.refcodes.struct.Property;
import org.refcodes.struct.PropertyImpl;
import org.refcodes.struct.PropertyImpl.PropertyBuilderImpl;
/**
* The {@link RequestCookie} represents a request cookies: We use URL encoding /
* decoding for the cookie value (regarding {@link #fromHttpCookie(String)} and
* {@link #toHttpCookie()}) to make life easier and not fall into the trap of
* unescaped values.
*/
public class RequestCookie extends PropertyBuilderImpl implements Cookie {
// /////////////////////////////////////////////////////////////////////////
// METHODS:
// /////////////////////////////////////////////////////////////////////////
/**
* {@inheritDoc}
*/
@Override
public RequestCookie withHttpCookie( String aCookie ) {
fromHttpCookie( aCookie );
return this;
}
/**
* {@inheritDoc}
*/
@Override
public void fromHttpCookie( String aHttpCookie ) {
final Property theProperty = new PropertyImpl( aHttpCookie );
setKey( theProperty.getKey() );
// Cookie value URL decoding -->
try {
setValue( URLDecoder.decode( theProperty.getValue(), Encoding.UTF_8.getCode() ) );
}
catch ( UnsupportedEncodingException ignore ) {
setValue( theProperty.getValue() );
}
// <-- Cookie value URL decoding
}
/**
* {@inheritDoc}
*/
@Override
public String toHttpCookie() {
// Cookie value URL encoding -->
String theValue = null;
try {
theValue = URLEncoder.encode( getValue(), Encoding.UTF_8.getCode() );
}
catch ( UnsupportedEncodingException ignore ) {
theValue = getValue();
}
// <-- Cookie value URL encoding
return getKey() + Delimiter.COOKIE_TUPEL.getChar() + theValue;
}
/**
* {@inheritDoc}
*/
@Override
public String toString() {
return getClass().getName() + "@[key=" + _key + ", value=" + _value + "]";
}
/**
* Constructs a {@link RequestCookie}.
*/
public RequestCookie() {}
/**
* Constructs a {@link RequestCookie} with the given cookie name and value.
*
* @param aCookieName The name of the cookie.
* @param aValue The value for the cookie.
*/
public RequestCookie( String aCookieName, String aValue ) {
super( aCookieName, aValue );
}
/**
* Constructs a {@link RequestCookie}.
*
* @param aHttpCookie The text as being found in the according HTTP header
* field.
*/
public RequestCookie( String aHttpCookie ) {
fromHttpCookie( aHttpCookie );
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy