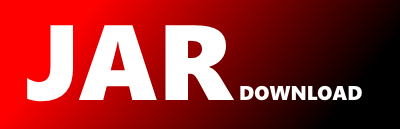
poem.boundary.internal.command_handler.DisplayRandomPoem Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of poem-hexagon Show documentation
Show all versions of poem-hexagon Show documentation
A simple example for a hexagonal architecture.
The newest version!
package poem.boundary.internal.command_handler;
import java.util.Arrays;
import java.util.List;
import java.util.Optional;
import java.util.function.Consumer;
import java.util.stream.Collectors;
import poem.boundary.driven_port.IObtainPoems;
import poem.boundary.driven_port.IWriteLines;
import poem.boundary.internal.domain.Poem;
import poem.boundary.internal.domain.RandomPoemPicker;
import poem.command.AskForPoem;
/**
* The command handler for displaying a random poem.
*
* @author b_muth
*
*/
public class DisplayRandomPoem implements Consumer {
private IObtainPoems poemObtainer;
private RandomPoemPicker randomPoemPicker;
private IWriteLines lineWriter;
public DisplayRandomPoem(IObtainPoems poemObtainer, IWriteLines lineWriter) {
this.poemObtainer = poemObtainer;
this.randomPoemPicker = new RandomPoemPicker();
this.lineWriter = lineWriter;
}
@Override
public void accept(AskForPoem askForPoem) {
List poems = obtainPoems(askForPoem);
Optional poem = pickRandomPoem(poems);
writeLines(poem);
}
private List obtainPoems(AskForPoem askForPoem) {
String language = askForPoem.getLanguage();
String[] poems = poemObtainer.getMePoems(language);
List poemDomainObjects =
Arrays.stream(poems)
.map(Poem::new)
.collect(Collectors.toList());
return poemDomainObjects;
}
private Optional pickRandomPoem(List poemList) {
Optional randomPoem = randomPoemPicker.pickPoem(poemList);
return randomPoem;
}
private void writeLines(Optional poem) {
poem.ifPresent(p -> lineWriter.writeLines(p.getVerses()));
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy