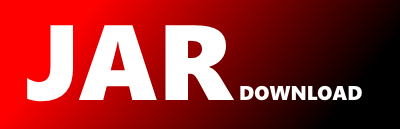
org.requirementsascode.builder.FlowlessStepPart Maven / Gradle / Ivy
Show all versions of requirementsascodecore Show documentation
package org.requirementsascode.builder;
import static org.requirementsascode.builder.FlowlessUserPart.flowlessOnPart;
import static org.requirementsascode.builder.FlowlessUserPart.flowlessUserPart;
import static org.requirementsascode.builder.StepPart.stepPartWithoutFlow;
import java.util.function.Supplier;
import org.requirementsascode.Actor;
import org.requirementsascode.Condition;
import org.requirementsascode.ModelRunner;
public class FlowlessStepPart {
private final StepPart stepPart;
private final long flowlessStepCounter;
private FlowlessStepPart(String stepName, UseCasePart useCasePart, Condition optionalCondition, long flowlessStepCounter) {
this.stepPart = stepPartWithoutFlow(stepName, useCasePart, optionalCondition);
this.flowlessStepCounter = flowlessStepCounter;
setDefaultActorAsActor(useCasePart);
}
private void setDefaultActorAsActor(UseCasePart useCasePart) {
stepPart.getStep().setActors(new Actor[] {useCasePart.getDefaultActor()});
}
static FlowlessStepPart flowlessStepPart(String stepName, UseCasePart useCasePart, Condition optionalCondition, long flowlessStepCounter) {
return new FlowlessStepPart(stepName, useCasePart, optionalCondition, flowlessStepCounter);
}
/**
* Defines the type of user commands that this step accepts. Commands of this
* type can cause a system reaction.
*
*
* Given that the step's condition is true, the system reacts to objects that
* are instances of the specified class or instances of any direct or indirect
* subclass of the specified class.
*
* @param commandClass the class of commands the system reacts to in this step
* @param the type of the class
* @return the created user part of this step
*/
public FlowlessUserPart user(Class commandClass) {
FlowlessUserPart flowlessUserPart = flowlessUserPart(commandClass, stepPart, flowlessStepCounter);
return flowlessUserPart;
}
/**
* Defines the type of system event objects or exceptions that this step
* handles. Events of the specified type can cause a system reaction.
*
*
* Given that the step's condition is true, the system reacts to objects that
* are instances of the specified class or instances of any direct or indirect
* subclass of the specified class.
*
* @param messageClass the class of messages the system reacts to
* @param the type of the class
* @return the created user part of this step
*/
public FlowlessUserPart on(Class messageClass) {
FlowlessUserPart flowlessUserPart = flowlessOnPart(messageClass, stepPart, flowlessStepCounter);
return flowlessUserPart;
}
/**
* Defines an "autonomous system reaction", meaning the system will react
* without needing a message provided via {@link ModelRunner#reactTo(Object)}.
*
* @param systemReaction the autonomous system reaction
* @return the created system part of this step
*/
public FlowlessSystemPart system(Runnable systemReaction) {
FlowlessSystemPart flowlessSystemPart = user(ModelRunner.class).system(systemReaction);
return flowlessSystemPart;
}
/**
* Defines an "autonomous system reaction", meaning the system will react
* without needing a message provided via {@link ModelRunner#reactTo(Object)}.
* After executing the system reaction, the runner will publish the returned
* event.
*
* @param systemReaction the autonomous system reaction, that returns a single
* event to be published.
* @return the created system part of this step
*/
public FlowlessSystemPart systemPublish(Supplier> systemReaction) {
@SuppressWarnings("unchecked")
FlowlessSystemPart flowlessSystemPart = user(ModelRunner.class).systemPublish(
(Supplier