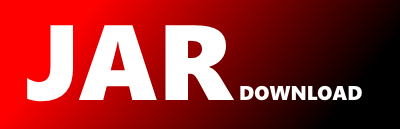
org.requirementsascode.builder.FlowlessUserPart Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of requirementsascodecore Show documentation
Show all versions of requirementsascodecore Show documentation
Enables you to define and run executable use case specifications, in your code.
package org.requirementsascode.builder;
import static org.requirementsascode.builder.FlowlessSystemPart.flowlessSystemPartWithConsumer;
import static org.requirementsascode.builder.FlowlessSystemPart.flowlessSystemPartWithFunction;
import static org.requirementsascode.builder.FlowlessSystemPart.flowlessSystemPartWithRunnable;
import static org.requirementsascode.builder.FlowlessSystemPart.flowlessSystemPartWithSupplier;
import java.util.Objects;
import java.util.function.Consumer;
import java.util.function.Function;
import java.util.function.Supplier;
import org.requirementsascode.Model;
import org.requirementsascode.ModelRunner;
/**
* Part used by the {@link ModelBuilder} to build a {@link Model}. Wraps
* {@link StepUserPart}.
*
* @author b_muth
*/
public class FlowlessUserPart {
private StepUserPart stepUserPart;
private long flowlessStepCounter;
private FlowlessUserPart(StepUserPart stepUserPart, long flowlessStepCounter) {
this.stepUserPart = Objects.requireNonNull(stepUserPart);
this.flowlessStepCounter = flowlessStepCounter;
}
static FlowlessUserPart flowlessUserPart(Class commandClass, StepPart stepPart, long flowlessStepCounter) {
StepUserPart stepUserPart = stepPart.user(commandClass);
return new FlowlessUserPart<>(stepUserPart, flowlessStepCounter);
}
static FlowlessUserPart flowlessOnPart(Class eventClass, StepPart stepPart, long flowlessStepCounter) {
StepUserPart stepUserPart = stepPart.on(eventClass);
return new FlowlessUserPart<>(stepUserPart, flowlessStepCounter);
}
/**
* Defines the system reaction. The system will react as specified to the
* message passed in, when {@link ModelRunner#reactTo(Object)} is called.
*
* @param systemReaction the specified system reaction
* @return the created flowless system part
*/
public FlowlessSystemPart system(Consumer super T> systemReaction) {
FlowlessSystemPart flowlessSystemPart = flowlessSystemPartWithConsumer(stepUserPart, systemReaction,
flowlessStepCounter);
return flowlessSystemPart;
}
/**
* Defines the system reaction. The system will react as specified, but it will
* ignore the message passed in, when {@link ModelRunner#reactTo(Object)} is
* called.
*
* @param systemReaction the specified system reaction
* @return the created flowless system part
*/
public FlowlessSystemPart system(Runnable systemReaction) {
FlowlessSystemPart flowlessSystemPart = flowlessSystemPartWithRunnable(stepUserPart, systemReaction,
flowlessStepCounter);
return flowlessSystemPart;
}
/**
* Defines the system reaction. The system will react as specified to the
* message passed in, when you call {@link ModelRunner#reactTo(Object)}. After
* executing the system reaction, the runner will publish the returned event.
*
* @param systemReaction the specified system reaction, that returns an event to
* be published.
* @return the created flowless system part
*/
public FlowlessSystemPart systemPublish(Function super T, ?> systemReaction) {
FlowlessSystemPart flowlessSystemPart = flowlessSystemPartWithFunction(stepUserPart, systemReaction,
flowlessStepCounter);
return flowlessSystemPart;
}
/**
* Defines the system reaction. After executing the system reaction, the runner
* will publish the returned event.
*
* @param systemReaction the specified system reaction, that returns an event to
* be published.
* @return the created flowless system part
*/
public FlowlessSystemPart systemPublish(Supplier> systemReaction) {
FlowlessSystemPart flowlessSystemPart = flowlessSystemPartWithSupplier(stepUserPart, systemReaction,
flowlessStepCounter);
return flowlessSystemPart;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy