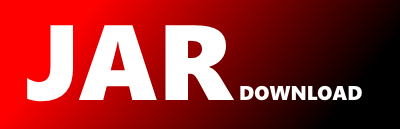
org.requirementsascode.builder.StepAsPart Maven / Gradle / Ivy
Show all versions of requirementsascodecore Show documentation
package org.requirementsascode.builder;
import static org.requirementsascode.builder.StepUserPart.stepUserPart;
import java.util.Objects;
import java.util.function.Supplier;
import org.requirementsascode.AbstractActor;
import org.requirementsascode.FlowStep;
import org.requirementsascode.Model;
import org.requirementsascode.ModelRunner;
import org.requirementsascode.Step;
import org.requirementsascode.exception.NoSuchElementInModel;
import org.requirementsascode.systemreaction.ContinuesAfter;
import org.requirementsascode.systemreaction.ContinuesAt;
/**
* Part used by the {@link ModelBuilder} to build a {@link Model}.
*
* @see Step#setActors(AbstractActor[])
* @author b_muth
*/
public class StepAsPart {
private Step step;
private StepPart stepPart;
private StepAsPart(AbstractActor[] actors, StepPart stepPart) {
Objects.requireNonNull(actors);
this.stepPart = Objects.requireNonNull(stepPart);
this.step = stepPart.getStep();
step.setActors(actors);
}
static StepAsPart stepAsPart(AbstractActor[] actors, StepPart stepPart) {
return new StepAsPart(actors, stepPart);
}
/**
* Defines the type of user commands that this step accepts. Commands of this
* type can cause a system reaction.
*
*
* Given that the step's condition is true, and the actor is right, the system
* reacts to objects that are instances of the specified class or instances of
* any direct or indirect subclass of the specified class.
*
* @param commandClass the class of commands the system reacts to in this step
* @param the type of the class
* @return the created user part of this step
*/
public StepUserPart user(Class commandClass) {
Objects.requireNonNull(commandClass);
return stepUserPart(commandClass, stepPart);
}
/**
* Defines an "autonomous system reaction", meaning the system will react
* without needing a message provided via {@link ModelRunner#reactTo(Object)}.
*
* @param systemReaction the autonomous system reaction
* @return the created system part of this step
*/
public StepSystemPart system(Runnable systemReaction) {
StepSystemPart systemPart = user(ModelRunner.class).system(systemReaction);
return systemPart;
}
/**
* Defines an "autonomous system reaction", meaning the system will react
* without needing a message provided via {@link ModelRunner#reactTo(Object)}.
* After executing the system reaction, the runner will publish the returned
* event.
*
* @param systemReaction the autonomous system reaction, that returns a single
* event to be published.
* @return the created system part of this step
*/
public StepSystemPart systemPublish(Supplier> systemReaction) {
@SuppressWarnings("unchecked")
StepSystemPart systemPart = user(ModelRunner.class).systemPublish((Supplier