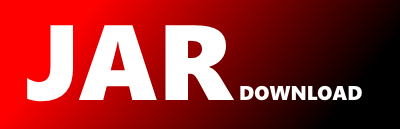
org.restcomm.timers.timer.FaultTolerantTimerTimerTask Maven / Gradle / Ivy
/*
* TeleStax, Open Source Cloud Communications
* Copyright 2011-2016, Telestax Inc and individual contributors
* by the @authors tag.
*
* This program is free software: you can redistribute it and/or modify
* under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation; either version 3 of
* the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see
*/
package org.restcomm.timers.timer;
import java.lang.reflect.Field;
import java.security.AccessController;
import java.security.PrivilegedAction;
import org.apache.log4j.Logger;
import org.restcomm.timers.FaultTolerantScheduler;
import org.restcomm.timers.TimerTask;
/**
* A concrete {@link FaultTolerantScheduler} {@link TimerTask} to wrap a {@link java.util.TimerTask}.
*
* @author martins
* @author András Kőkuti
*
*/
public class FaultTolerantTimerTimerTask extends TimerTask {
private static final Logger logger = Logger.getLogger(FaultTolerantTimerTimerTask.class);
/**
*
*/
private final FaultTolerantScheduler scheduler;
/**
*
*/
private final FaultTolerantTimerTimerTaskData taskData;
/**
*
* @param taskData
*/
public FaultTolerantTimerTimerTask(FaultTolerantTimerTimerTaskData taskData,FaultTolerantScheduler scheduler) {
super(taskData);
this.taskData = taskData;
this.scheduler = scheduler;
setPeriod(taskData.getJavaUtilTimerTask(),taskData.getPeriod());
}
/*
* (non-Javadoc)
* @see org.mobicents.timers.TimerTask#runTask()
*/
public void runTask() {
if (isCanceled()) {
scheduler.cancel(this.getData().getTaskID());
}
else {
try{
taskData.getJavaUtilTimerTask().run();
if(taskData.getPeriodicScheduleStrategy() == null) {
scheduler.cancel(this.getData().getTaskID());
}
else {
if (logger.isDebugEnabled()) {
logger.debug("task data has a periodic schedule strategy, not cancelling the task");
}
}
} catch(Throwable e) {
logger.error(e.getMessage(),e);
}
}
}
/**
*
* @return
*/
public boolean isCanceled() {
if(getTaskStatus(taskData.getJavaUtilTimerTask()) == 3 ) {
return true;
}
else {
return false;
}
}
private int getTaskStatus(final java.util.TimerTask timerTask) {
if(System.getSecurityManager()!=null)
{
return AccessController.doPrivileged(new PrivilegedAction(){
public Integer run() {
return _getTaskStatus(timerTask);
}
});
}else
{
return _getTaskStatus(timerTask);
}
}
private Integer _getTaskStatus(java.util.TimerTask timerTask) {
Class> cc = java.util.TimerTask.class;
try {
Field stateField=cc.getDeclaredField("state");
stateField.setAccessible(true);
Integer taskStatus= (Integer) stateField.get(taskData.getJavaUtilTimerTask());
stateField.setAccessible(false);
return taskStatus;
} catch (Exception e) {
throw new RuntimeException("Fialed to get status",e);
}
}
/**
* @param javaUtilTimerTask
* @param period
*/
private void setPeriod(final java.util.TimerTask javaUtilTimerTask,final long period) {
if(System.getSecurityManager()!=null)
{
AccessController.doPrivileged(new PrivilegedAction
© 2015 - 2025 Weber Informatics LLC | Privacy Policy