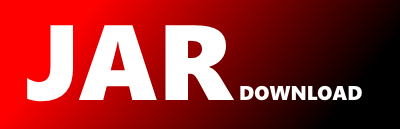
org.restcomm.connect.dao.mybatis.MybatisNotificationsDao Maven / Gradle / Ivy
/*
* TeleStax, Open Source Cloud Communications
* Copyright 2011-2014, Telestax Inc and individual contributors
* by the @authors tag.
*
* This program is free software: you can redistribute it and/or modify
* under the terms of the GNU Affero General Public License as
* published by the Free Software Foundation; either version 3 of
* the License, or (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU Affero General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see
*
*/
package org.restcomm.connect.dao.mybatis;
import org.apache.ibatis.session.SqlSession;
import org.apache.ibatis.session.SqlSessionFactory;
import org.joda.time.DateTime;
import org.restcomm.connect.commons.annotations.concurrency.ThreadSafe;
import org.restcomm.connect.dao.NotificationsDao;
import org.restcomm.connect.dao.entities.Notification;
import org.restcomm.connect.commons.dao.Sid;
import java.net.URI;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import static org.restcomm.connect.dao.DaoUtils.readDateTime;
import static org.restcomm.connect.dao.DaoUtils.readInteger;
import static org.restcomm.connect.dao.DaoUtils.readSid;
import static org.restcomm.connect.dao.DaoUtils.readString;
import static org.restcomm.connect.dao.DaoUtils.readUri;
import static org.restcomm.connect.dao.DaoUtils.writeDateTime;
import static org.restcomm.connect.dao.DaoUtils.writeSid;
import static org.restcomm.connect.dao.DaoUtils.writeUri;
/**
* @author [email protected] (Thomas Quintana)
*/
@ThreadSafe
public final class MybatisNotificationsDao implements NotificationsDao {
private static final String namespace = "org.mobicents.servlet.sip.restcomm.dao.NotificationsDao.";
private final SqlSessionFactory sessions;
public MybatisNotificationsDao(final SqlSessionFactory sessions) {
super();
this.sessions = sessions;
}
@Override
public void addNotification(final Notification notification) {
final SqlSession session = sessions.openSession();
try {
session.insert(namespace + "addNotification", toMap(notification));
session.commit();
} finally {
session.close();
}
}
@Override
public Notification getNotification(final Sid sid) {
final SqlSession session = sessions.openSession();
try {
final Map result = session.selectOne(namespace + "getNotification", sid.toString());
if (result != null) {
return toNotification(result);
} else {
return null;
}
} finally {
session.close();
}
}
@Override
public List getNotifications(final Sid accountSid) {
return getNotifications(namespace + "getNotifications", accountSid.toString());
}
@Override
public List getNotificationsByCall(final Sid callSid) {
return getNotifications(namespace + "getNotificationsByCall", callSid.toString());
}
@Override
public List getNotificationsByLogLevel(final int logLevel) {
return getNotifications(namespace + "getNotificationsByLogLevel", logLevel);
}
@Override
public List getNotificationsByMessageDate(final DateTime messageDate) {
final Map parameters = new HashMap();
parameters.put("start_date", messageDate.toDate());
parameters.put("end_date", messageDate.plusDays(1).toDate());
return getNotifications(namespace + "getNotificationsByMessageDate", parameters);
}
private List getNotifications(final String selector, final Object input) {
final SqlSession session = sessions.openSession();
try {
final List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy