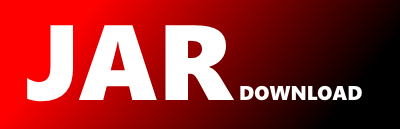
org.restheart.mongodb.interceptors.JsonSchemaAfterWriteChecker Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of restheart-mongodb Show documentation
Show all versions of restheart-mongodb Show documentation
RESTHeart MongoDB - MongoDB plugin
/*-
* ========================LICENSE_START=================================
* restheart-mongodb
* %%
* Copyright (C) 2014 - 2024 SoftInstigate
* %%
* This program is free software: you can redistribute it and/or modify
* it under the terms of the GNU Affero General Public License as published by
* the Free Software Foundation, either version 3 of the License, or
* (at your option) any later version.
*
* This program is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the
* GNU General Public License for more details.
*
* You should have received a copy of the GNU Affero General Public License
* along with this program. If not, see .
* =========================LICENSE_END==================================
*/
package org.restheart.mongodb.interceptors;
import java.util.ArrayList;
import java.util.List;
import org.bson.BsonDocument;
import org.json.JSONObject;
import org.restheart.exchange.MongoRequest;
import org.restheart.exchange.MongoResponse;
import org.restheart.mongodb.RHMongoClients;
import org.restheart.plugins.InterceptPoint;
import org.restheart.plugins.RegisterPlugin;
import org.restheart.utils.BsonUtils;
/**
*
* Checks documents according to the specified JSON schema
*
* This intercetor is able to check PATCH requests (excluding bulk PATCH). Other
* requests are checked by jsonSchemaBeforeWrite
*
* It checks the request content against the JSON schema specified by the
* 'jsonSchema' collection metadata:
*
* { "jsonSchema": { "schemaId": <schemaId> "schemaStoreDb":
* <schemaStoreDb> } }
*
* schemaStoreDb is optional, default value is same db
*
* @author Andrea Di Cesare {@literal }
*/
@RegisterPlugin(
name = "jsonSchemaAfterWrite",
description = "Checks the request content against the JSON schema specified by the 'jsonSchema' collection metadata",
interceptPoint = InterceptPoint.RESPONSE)
public class JsonSchemaAfterWriteChecker extends JsonSchemaBeforeWriteChecker {
@Override
public void handle(MongoRequest request, MongoResponse response) throws Exception {
super.handle(request, response);
var mclient = RHMongoClients.mclient();
if (mclient == null) {
throw new IllegalStateException("mclient not availabe");
}
if (request.isInError()) {
response.rollback(mclient);
}
}
@Override
public boolean resolve(MongoRequest request, MongoResponse response) {
return request.isHandledBy("mongo")
&& request.getCollectionProps() != null
&& (request.isPatch() && !request.isBulkDocuments())
&& request.getCollectionProps() != null
&& request.getCollectionProps()
.containsKey("jsonSchema")
&& request.getCollectionProps()
.get("jsonSchema")
.isDocument()
&& (response.getDbOperationResult() != null
&& response.getDbOperationResult().getHttpCode() < 300);
}
String documentToCheck(MongoRequest request, MongoResponse response) {
return response.getDbOperationResult().getNewData() == null
? "{}"
: BsonUtils.toJson(response.getDbOperationResult().getNewData(), request.getJsonMode());
}
@Override
List documentsToCheck(MongoRequest request, MongoResponse response) {
var ret = new ArrayList();
var content = response.getDbOperationResult().getNewData() == null
? new BsonDocument()
: response.getDbOperationResult().getNewData();
ret.add(new JSONObject(BsonUtils.toJson(content, request.getJsonMode())));
return ret;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy