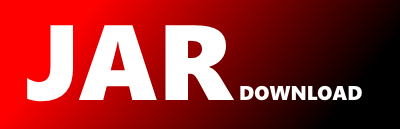
org.resthub.common.service.CrudService Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of resthub-common Show documentation
Show all versions of resthub-common Show documentation
RESThub core include Embeded datasource, Generic Repository and Generic CRUD services
package org.resthub.common.service;
import org.springframework.data.domain.Page;
import org.springframework.data.domain.Pageable;
import java.io.Serializable;
import java.util.List;
/**
* CRUD Service interface.
*
* @param Your resource POJO to manage, maybe an entity or DTO class
* @param Resource id type, usually Long or String
*/
public interface CrudService {
/**
* Create new resource.
*
* @param resource
* Resource to create
* @return new resource
*/
T create(T resource);
/**
* Update existing resource.
*
* @param resource
* Resource to update
* @return resource updated
*/
T update(T resource);
/**
* Delete existing resource.
*
* @param resource
* Resource to delete
*/
void delete(T resource);
/**
* Delete existing resource.
*
* @param id
* Resource id
*/
void delete(ID id);
/**
* Delete all existing resource. Do not use cascade remove (not a choice -> JPA specs)
*/
void deleteAll();
/**
* Delete all existing resource, including linked entities with cascade delete
*/
void deleteAllWithCascade();
/**
* Find resource by id.
*
* @param id
* Resource id
* @return resource
*/
T findById(ID id);
/**
* Find all resources.
*
* @return a list of all resources.
*/
List findAll();
/**
* Find all resources (pageable).
*
* @param pageRequest
* page request
* @return resources
*/
Page findAll(Pageable pageRequest);
/**
* Count all resources.
*
* @return number of resources
*/
Long count();
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy