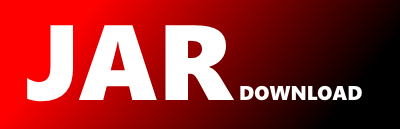
org.restler.spring.mvc.files.RestlerMultipartFile Maven / Gradle / Ivy
package org.restler.spring.mvc.files;
import org.springframework.web.multipart.MultipartFile;
import java.io.*;
public class RestlerMultipartFile implements MultipartFile {
private final String name;
private final File file;
private final String contentType;
public RestlerMultipartFile(String name, File file, String contentType) {
this.name = name;
this.file = file;
this.contentType = contentType;
}
@Override
public String getName() {
return name;
}
@Override
public String getOriginalFilename() {
return file.getName();
}
@Override
public String getContentType() {
return contentType;
}
@Override
public boolean isEmpty() {
return getSize() == 0;
}
@Override
public long getSize() {
return file.length();
}
@Override
public byte[] getBytes() throws IOException {
InputStream inputStream = getInputStream();
byte[] buffer = new byte[8192];
int bytesRead;
ByteArrayOutputStream output = new ByteArrayOutputStream();
while ((bytesRead = inputStream.read(buffer)) != -1) {
output.write(buffer, 0, bytesRead);
}
return output.toByteArray();
}
@Override
public InputStream getInputStream() throws IOException {
return new FileInputStream(file);
}
@Override
public void transferTo(File dest) throws IOException, IllegalStateException {
throw new UnsupportedOperationException();
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy