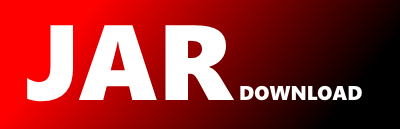
org.revenj.patterns.DataContext Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of revenj-core Show documentation
Show all versions of revenj-core Show documentation
DSL Platform compatible backend (https://dsl-platform.com)
The newest version!
package org.revenj.patterns;
import rx.Observable;
import java.io.IOException;
import java.util.*;
public interface DataContext {
List find(Class manifest, Collection uris);
default Optional find(Class manifest, String uri) {
List found = find(manifest, Collections.singletonList(uri));
return found.size() == 1 ? Optional.of(found.get(0)) : Optional.empty();
}
default List find(Class manifest, String[] uris) {
return find(manifest, Arrays.asList(uris));
}
Query query(Class manifest, Specification filter);
default Query query(Class manifest) {
return query(manifest, null);
}
List search(Class manifest, Specification filter, Integer limit, Integer offset);
default List search(Class manifest) {
return search(manifest, null, null, null);
}
default List search(Class manifest, Specification filter) {
return search(manifest, filter, null, null);
}
default List search(Class manifest, Specification filter, int limit) {
return search(manifest, filter, limit, null);
}
long count(Class manifest, Specification filter);
default long count(Class manifest) {
return count(manifest, null);
}
boolean exists(Class manifest, Specification filter);
default boolean exists(Class manifest) {
return exists(manifest, null);
}
void create(Collection aggregates) throws IOException;
default void create(T aggregate) throws IOException {
create(Collections.singletonList(aggregate));
}
void updatePairs(Collection> pairs) throws IOException;
default void update(T oldAggregate, T newAggregate) throws IOException {
updatePairs(Collections.singletonList(new HashMap.SimpleEntry<>(oldAggregate, newAggregate)));
}
default void update(T aggregate) throws IOException {
updatePairs(Collections.singletonList(new HashMap.SimpleEntry<>(null, aggregate)));
}
default void update(Collection aggregates) throws IOException {
Collection> collection = new ArrayList<>(aggregates.size());
for (T item : aggregates) {
collection.add(new AbstractMap.SimpleEntry<>(null, item));
}
updatePairs(collection);
}
void delete(Collection aggregates) throws IOException;
default void delete(T aggregate) throws IOException {
delete(Collections.singletonList(aggregate));
}
void submit(Collection events);
default void submit(T event) {
submit(Collections.singletonList(event));
}
void queue(Collection events);
default void queue(T event) {
queue(Collections.singletonList(event));
}
T populate(Report report);
Observable> track(Class manifest);
List> history(Class manifest, Collection uris);
default Optional> history(Class manifest, String uri) {
List> found = history(manifest, Collections.singletonList(uri));
return found.size() == 1 ? Optional.of(found.get(0)) : Optional.>empty();
}
default List> history(Class manifest, String[] uris) {
return history(manifest, Arrays.asList(uris));
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy