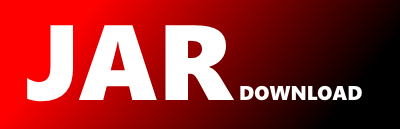
org.richfaces.renderkit.ComponentControlBehaviorRenderer Maven / Gradle / Ivy
/*
* JBoss, Home of Professional Open Source
* Copyright ${year}, Red Hat, Inc. and individual contributors
* by the @authors tag. See the copyright.txt in the distribution for a
* full listing of individual contributors.
*
* This is free software; you can redistribute it and/or modify it
* under the terms of the GNU Lesser General Public License as
* published by the Free Software Foundation; either version 2.1 of
* the License, or (at your option) any later version.
*
* This software is distributed in the hope that it will be useful,
* but WITHOUT ANY WARRANTY; without even the implied warranty of
* MERCHANTABILITY or FITNESS FOR A PARTICULAR PURPOSE. See the GNU
* Lesser General Public License for more details.
*
* You should have received a copy of the GNU Lesser General Public
* License along with this software; if not, write to the Free
* Software Foundation, Inc., 51 Franklin St, Fifth Floor, Boston, MA
* 02110-1301 USA, or see the FSF site: http://www.fsf.org.
*/
package org.richfaces.renderkit;
import java.util.ArrayList;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.regex.Pattern;
import javax.faces.application.ResourceDependencies;
import javax.faces.application.ResourceDependency;
import javax.faces.component.UIComponent;
import javax.faces.component.UIParameter;
import javax.faces.component.behavior.ClientBehavior;
import javax.faces.component.behavior.ClientBehaviorContext;
import javax.faces.context.FacesContext;
import javax.faces.render.ClientBehaviorRenderer;
import javax.faces.render.FacesBehaviorRenderer;
import javax.faces.render.RenderKitFactory;
import org.ajax4jsf.javascript.JSFunction;
import org.ajax4jsf.javascript.JSFunctionDefinition;
import org.ajax4jsf.javascript.JSReference;
import org.ajax4jsf.javascript.ScriptUtils;
import org.richfaces.component.UIHashParameter;
import org.richfaces.component.behavior.ComponentControlBehavior;
import org.richfaces.renderkit.util.RendererUtils;
/**
* @author Anton Belevich
*
*/
@FacesBehaviorRenderer(rendererType = "org.richfaces.behavior.ComponentControlBehavior", renderKitId = RenderKitFactory.HTML_BASIC_RENDER_KIT)
@ResourceDependencies({ @ResourceDependency(library = "org.richfaces", name = "ajax.reslib"),
@ResourceDependency(library = "org.richfaces", name = "base-component.reslib"),
@ResourceDependency(name = "richfaces-event.js"),
@ResourceDependency(library = "org.richfaces", name = "component-control.js") })
public class ComponentControlBehaviorRenderer extends ClientBehaviorRenderer {
/**
*
*/
private static final RendererUtils RENDERER_UTILS = RendererUtils.getInstance();
private static final String FUNC_NAME = "RichFaces.ui.ComponentControl.execute";
private static final String REF_EVENT = "event";
private static final String REF_COMPONENT = "component";
private static final String PARAM_CALLBACK = "callback";
private static final String PARAM_TARGET = "target";
private static final String PARAM_SELECTOR = "selector";
private static final String PARAM_ONBEFOREOPERATION = "onbeforeoperation";
private static final Pattern COMMA_SEPARATED_STRING = Pattern.compile("\\s*,\\s*");
private boolean isEmpty(String value) {
return (value == null || value.trim().length() == 0);
}
@Override
public String getScript(ClientBehaviorContext behaviorContext, ClientBehavior behavior) {
FacesContext facesContext = behaviorContext.getFacesContext();
ComponentControlBehavior controlBehavior = (ComponentControlBehavior) behavior;
String apiFunctionName = controlBehavior.getOperation();
String targetSourceString = controlBehavior.getTarget();
String selector = controlBehavior.getSelector();
// Fix https://issues.jboss.org/browse/RF-9745
if (isEmpty(apiFunctionName) || (isEmpty(targetSourceString) && isEmpty(selector))) {
throw new IllegalArgumentException(
"One of the necessary attributes is null or empty. Check operation attribute and selector or target attributes.");
}
JSFunctionDefinition callback = new JSFunctionDefinition();
callback.addParameter(new JSReference(REF_EVENT));
callback.addParameter(new JSReference(REF_COMPONENT));
// create callback function
StringBuffer script = new StringBuffer();
script.append(REF_COMPONENT).append("['").append(apiFunctionName).append("'].").append("apply").append("(");
// get client api function parameters
List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy