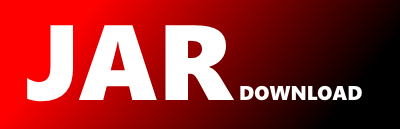
org.robokind.api.animation.library.AnimationLibraryLoader Maven / Gradle / Ivy
/*
* Copyright 2011 Hanson Robokind LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.robokind.api.animation.library;
import java.io.File;
import java.io.FilenameFilter;
import java.util.ArrayList;
import java.util.List;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.robokind.api.animation.Animation;
import org.robokind.api.animation.xml.AnimationFileReader;
/**
*
* @author Matthew Stevenson
*/
public class AnimationLibraryLoader{
private final static Logger theLogger = Logger.getLogger(AnimationLibraryLoader.class.getName());
public static AnimationLibrary loadAnimationFolder(String libraryId,
AnimationFileReader reader, String path, boolean recursive){
if(libraryId == null || reader == null || path == null){
throw new NullPointerException();
}
File animDir = new File(path);
List files = getFiles(animDir, null, recursive);
List anims = loadAnimations(files, reader);
AnimationLibrary lib = new DefaultAnimationLibrary(libraryId);
for(Animation anim : anims){
lib.add(anim);
}
return lib;
}
private static List loadAnimations(
List files, AnimationFileReader reader){
List anims = new ArrayList(files.size());
for(File file : files){
try{
Animation anim = reader.readAnimation(file.getAbsolutePath());
if(anim != null){
anim.setVersion(file.getName(), anim.getVersion().getNumber());
anims.add(anim);
}
}catch(Exception ex){
theLogger.log(Level.WARNING, "Could not load animation at " +
file.getAbsolutePath(), ex);
}
}
return anims;
}
private static List getFiles(
File animDir, FilenameFilter filenameFilter, boolean recursive){
if(!animDir.exists()){
throw new IllegalArgumentException("Cannot find dir: " + animDir);
}else if(!animDir.isDirectory()){
throw new IllegalArgumentException("Not a dir: " + animDir);
}
File[] files;
if(filenameFilter == null){
files = animDir.listFiles();
}else{
files = animDir.listFiles(filenameFilter);
}
List fileList = new ArrayList(files.length);
List dirList = new ArrayList();
for(File f : files){
if(f.isFile()){
fileList.add(f);
}else if(f.isDirectory()){
dirList.add(f);
}
}
if(recursive){
for(File dir : dirList){
fileList.addAll(getFiles(dir, filenameFilter, recursive));
}
}
return fileList;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy