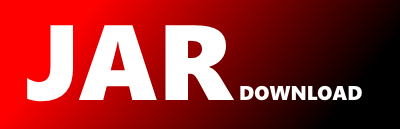
org.robokind.api.animation.messaging.RemoteAnimationPlayerHost Maven / Gradle / Ivy
/*
* Copyright 2012 Hanson Robokind LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.robokind.api.animation.messaging;
import java.util.HashMap;
import java.util.List;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.robokind.api.animation.Animation;
import org.robokind.api.animation.player.AnimationPlayer;
import org.robokind.api.animation.protocol.AnimationEvent;
import org.jflux.api.core.Listener;
import org.robokind.api.animation.player.AnimationJob;
import org.robokind.api.animation.protocol.AnimationSignal;
import org.robokind.api.common.playable.PlayState;
import org.robokind.api.common.utils.TimeUtils;
import org.robokind.api.messaging.MessageAsyncReceiver;
import org.robokind.api.messaging.MessageSender;
/**
*
* @author Matthew Stevenson
*/
public class RemoteAnimationPlayerHost implements Listener {
private final static Logger theLogger =
Logger.getLogger(RemoteAnimationPlayerHost.class.getName());
private AnimationPlayer myAnimationPlayer;
private MessageAsyncReceiver myAnimationReceiver;
private Listener myEventPlayer;
private MessageSender mySignalSender;
public RemoteAnimationPlayerHost(){
myEventPlayer = new HackedEventPlayer();
}
public void setAnimationPlayer(AnimationPlayer player){
if(myAnimationPlayer != null) {
myAnimationPlayer.removeAnimationSignalListener(this);
}
myAnimationPlayer = player;
if(myAnimationPlayer != null) {
myAnimationPlayer.addAnimationSignalListener(this);
}
}
public void setAnimationReceiver(
MessageAsyncReceiver receiver){
if(myAnimationReceiver != null){
myAnimationReceiver.removeListener(myEventPlayer);
}
myAnimationReceiver = receiver;
if(myAnimationReceiver != null){
myAnimationReceiver.addListener(myEventPlayer);
}
}
public void setSignalSender(MessageSender sender){
mySignalSender = sender;
}
@Override
public void handleEvent(AnimationSignal t) {
if(mySignalSender != null){
mySignalSender.notifyListeners(t);
}
}
public class AnimationEventPlayer implements Listener{
@Override
public void handleEvent(AnimationEvent event) {
if(event == null){
theLogger.warning("Ignoring null AnimaionEvent.");
return;
}
Animation anim = event.getAnimation();
if(anim == null){
theLogger.warning(
"Ignoring null Animaion from AnimationEvent.");
return;
}else if(myAnimationPlayer == null){
theLogger.warning("Animation Player is null. "
+ "Ignoring AnimaionEvent.");
return;
}
theLogger.log(Level.INFO,
"Sending Animation: {0}, to AnimationPlayer: {1}.",
new Object[]{
anim.getVersion().display(),
myAnimationPlayer.getAnimationPlayerId()});
myAnimationPlayer.playAnimation(event.getAnimation());
}
}
public class HackedEventPlayer implements Listener{
private Map myAnimationMap;
public HackedEventPlayer(){
myAnimationMap = new HashMap();
}
@Override
public void handleEvent(AnimationEvent event) {
if(event == null){
theLogger.warning("Ignoring null AnimaionEvent.");
return;
}
Animation anim = event.getAnimation();
if(anim == null){
theLogger.warning(
"Ignoring null Animaion from AnimationEvent.");
return;
}else if(myAnimationPlayer == null){
theLogger.warning("Animation Player is null. "
+ "Ignoring AnimaionEvent.");
return;
}
if("CLEAR".equals(event.getDestinationId())){
theLogger.log(Level.INFO,
"Clearing all animations from AnimationPlayer: {0}.",
myAnimationPlayer.getAnimationPlayerId());
List jobs = myAnimationPlayer.getCurrentAnimations();
if(jobs == null){
return;
}
for(AnimationJob job : jobs){
if(job == null){
continue;
}
myAnimationPlayer.removeAnimationJob(job);
}
return;
}
AnimationJob job = myAnimationMap.get(anim);
if("STOP".equals(event.getDestinationId())){
if(job != null){
theLogger.log(Level.INFO,
"Stopping Animation: {0}, from AnimationPlayer: {1}.",
new Object[]{anim.getVersion().display(),
myAnimationPlayer.getAnimationPlayerId()});
job.stop(TimeUtils.now());
}else{
theLogger.log(Level.INFO,
"Could not find Animation to stop: {0}, from AnimationPlayer: {1}.",
new Object[]{anim.getVersion().display(),
myAnimationPlayer.getAnimationPlayerId()});
}
return;
}
if(job == null){
job = myAnimationPlayer.playAnimation(event.getAnimation());
}else if(PlayState.RUNNING != job.getPlayState()){
job.start(TimeUtils.now());
}
boolean loop = "LOOP".equals(event.getDestinationId());
job.setLoop(loop);
String msg = loop ? "Looping" : "Playing";
theLogger.log(Level.INFO,
"{0} Animation: {1}, from AnimationPlayer: {2}.",
new Object[]{msg, anim.getVersion().display(),
myAnimationPlayer.getAnimationPlayerId()});
myAnimationMap.put(anim, job);
}
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy