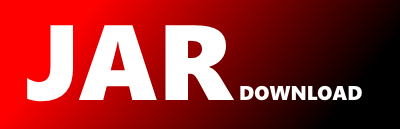
org.robokind.api.motion.servos.ServoRobot Maven / Gradle / Ivy
/*
* Copyright 2011 Hanson Robokind LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.robokind.api.motion.servos;
import java.beans.PropertyChangeEvent;
import java.util.*;
import java.util.Map.Entry;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.robokind.api.common.config.VersionProperty;
import org.robokind.api.common.position.NormalizedDouble;
import org.robokind.api.common.property.PropertyChangeAction;
import org.robokind.api.common.property.PropertyChangeMonitor;
import org.robokind.api.common.services.ServiceContext;
import org.robokind.api.motion.AbstractRobot;
import org.robokind.api.motion.Joint;
import org.robokind.api.motion.Robot;
import org.robokind.api.motion.servos.ServoController.ServoId;
import org.robokind.api.motion.servos.config.ServoConfig;
import org.robokind.api.motion.servos.config.ServoControllerConfig;
import org.robokind.api.motion.servos.config.ServoRobotConfig;
import org.robokind.api.motion.servos.utils.EmptyServoJoint;
import org.robokind.api.motion.servos.utils.ServoJointAdapter;
/**
* Robot implementation using Servos.
*
* @author Matthew Stevenson
*/
public class ServoRobot extends AbstractRobot {
private final static Logger theLogger = Logger.getLogger(ServoRobot.class.getName());
/**
* Controller type version name.
*/
public final static String VERSION_NAME = "Servo Robot Implementation";
/**
* Controller type version number.
*/
public final static String VERSION_NUMBER = "1.0";
/**
* Controller type VersionProperty.
*/
public final static VersionProperty VERSION = new VersionProperty(VERSION_NAME, VERSION_NUMBER);
private Map myServoIdMap;
private Map myServoJointAdapters;
private Map myControllers;
private List myControllerList;
private List myMissingJoints;
private PropertyChangeMonitor myChangeMonitor;
private boolean myConnectionFlag;
private ServoRobotConfig myRobotConfig;
/**
* Creates a new ServoRobot with the given BundleContext and RobotConfig.
* @param config Robot's configuration parameters
*/
public ServoRobot(ServoRobotConfig config){
super(config.getRobotId());
myRobotConfig = config;
myServoIdMap = new HashMap();
myControllers = new HashMap();
myControllerList = new ArrayList();
myServoJointAdapters = new HashMap();
myMissingJoints = new ArrayList();
myConnectionFlag = false;
Map ids = config.getIdMap();
if(ids == null){
throw new NullPointerException();
}
for(Entry e : ids.entrySet()){
Joint.Id jointId = e.getKey();
ServoController.ServoId servoId = e.getValue();
if(jointId == null || servoId == null){
throw new NullPointerException();
}
Robot.JointId jId = new Robot.JointId(getRobotId(), jointId);
myServoIdMap.put(jId, servoId);
}
for(ServoControllerContext scc : config.getControllerContexts()){
if(scc == null){
throw new NullPointerException();
}
ServoController controller = scc.getServoController();
ServoJointAdapter adapter = scc.getServoJointAdapter();
if(controller == null || adapter == null){
throw new NullPointerException();
}
ServoController.Id scId = controller.getId();
myControllers.put(scId, controller);
myControllerList.add(controller);
myServoJointAdapters.put(scId, adapter);
}
initChangeMonitor();
setEnabled(true);
}
@Override
public void setEnabled(boolean val) {
super.setEnabled(val);
for(ServoController sc : myControllerList){
sc.setEnabled(val);
}
}
private void initChangeMonitor(){
myChangeMonitor = new PropertyChangeMonitor();
PropertyChangeAction pca = new PropertyChangeAction() {
@Override
protected void run(PropertyChangeEvent event) {
jointsChanged(event);
}
};
myChangeMonitor.addAction(ServoController.PROP_SERVOS, pca);
myChangeMonitor.addAction(ServoController.PROP_SERVO_ADD, pca);
myChangeMonitor.addAction(ServoController.PROP_SERVO_REMOVE, pca);
for(ServoController c : myControllerList){
c.addPropertyChangeListener(myChangeMonitor);
}
}
@Override
public boolean connect(){
for(ServoController,?,?,?> controller : myControllerList){
try{
controller.connect();
}catch(Throwable t){
theLogger.log(Level.WARNING, "Unable to connect to ServoController.", t);
}
}
setServos();
boolean oldVal = myConnectionFlag;
myConnectionFlag = true;
firePropertyChange(PROP_CONNECTED, oldVal, myConnectionFlag);
return true;
}
private void setServos(){
clearJoints();
myMissingJoints.clear();
for(Entry e : myServoIdMap.entrySet()){
JointId jId = e.getKey();
ServoId sId = e.getValue();
ServoController.Id scId = sId.getControllerId();
ServoController sc = myControllers.get(scId);
ServoJointAdapter sja = myServoJointAdapters.get(scId);
if(sc == null || sja == null){
myMissingJoints.add(jId);
continue;
}
Servo s = sc.getServo(sId);
if(s == null){
myMissingJoints.add(jId);
continue;
}
ServoJoint j = sja.getJoint(jId.getJointId(), s);
if(j == null){
myMissingJoints.add(jId);
continue;
}
addJoint(j);
}
for(JointId robotJointId : myMissingJoints){
Joint.Id jointId = robotJointId.getJointId();
ServoId sId = myServoIdMap.get(robotJointId);
ServoController.Id scId = sId.getControllerId();
ServoConfig servoConf = null;
for(ServoControllerContext scc : myRobotConfig.getControllerContexts()){
ServiceContext<
ServoController,?,?,?>,
ServoControllerConfig,?> context = scc.myConnectionContext;
if(context == null){
continue;
}
ServoControllerConfig controllerConfig = context.getServiceConfiguration();
ServoController.Id controllerId = controllerConfig.getServoControllerId();
if(!scId.equals(controllerId)){
continue;
}
Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy