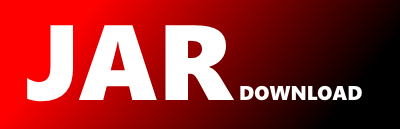
org.robokind.client.basic.RkCompassConnector Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 Hanson Robokind LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.robokind.client.basic;
import java.net.URISyntaxException;
import javax.jms.Destination;
import javax.jms.JMSException;
import javax.jms.Session;
import org.jflux.api.core.util.EmptyAdapter;
import org.jflux.api.messaging.rk.MessageAsyncReceiver;
import org.jflux.api.messaging.rk.MessageSender;
import org.robokind.api.sensor.CompassConfigEvent;
import org.robokind.api.sensor.DeviceReadPeriodEvent;
import org.robokind.api.sensor.FilteredVector3Event;
import org.robokind.api.sensor.imu.RemoteCompassServiceClient;
import org.robokind.impl.sensor.CompassConfigRecord;
import org.robokind.impl.sensor.DeviceReadPeriodRecord;
import org.robokind.impl.sensor.FilteredVector3Record;
import org.robokind.impl.sensor.HeaderRecord;
import org.robokind.client.basic.ConnectionContext.RkServiceConnector;
/**
*
* @author Jason G. Pallack
*/
final class RkCompassConnector extends RkServiceConnector{
final static String COMPASS_VALUE_RECEIVER = "compassValueReceiver";
final static String COMPASS_CONFIG_SENDER = "compassConfigSender";
final static String COMPASS_READ_PERIOD_SENDER = "compassReadPeriodSender";
private static RkCompassConnector theRkCompassConnector;
private String theCompassInputDest = "compassEvent";
private String theCompassConfigDest = "compassConfig";
private String theCompassReadDest = "compassRead";
static synchronized RkCompassConnector getConnector(){
if(theRkCompassConnector == null){
theRkCompassConnector = new RkCompassConnector();
}
return theRkCompassConnector;
}
@Override
protected synchronized void addConnection(Session session)
throws JMSException, URISyntaxException{
if(myConnectionContext == null || myConnectionsFlag){
return;
}
Destination compassValReceiver = ConnectionContext.getTopic(theCompassInputDest);
myConnectionContext.addAsyncReceiver(COMPASS_VALUE_RECEIVER, session, compassValReceiver,
FilteredVector3Record.class, FilteredVector3Record.SCHEMA$,
new EmptyAdapter());
Destination compassCfgSender = ConnectionContext.getTopic(theCompassConfigDest);
myConnectionContext.addSender(COMPASS_CONFIG_SENDER, session, compassCfgSender,
new EmptyAdapter());
Destination compassPerSender = ConnectionContext.getTopic(theCompassReadDest);
myConnectionContext.addSender(COMPASS_READ_PERIOD_SENDER, session, compassPerSender,
new EmptyAdapter());
myConnectionsFlag = true;
}
synchronized RemoteCompassServiceClient buildRemoteClient() {
if(myConnectionContext == null || !myConnectionsFlag){
return null;
}
MessageAsyncReceiver compassValReceiver =
myConnectionContext.getAsyncReceiver(COMPASS_VALUE_RECEIVER);
MessageSender> compassCfgSender =
myConnectionContext.getSender(COMPASS_CONFIG_SENDER);
MessageSender> compassPerSender =
myConnectionContext.getSender(COMPASS_READ_PERIOD_SENDER);
RemoteCompassServiceClient client =
new RemoteCompassServiceClient(
compassCfgSender, compassPerSender, compassValReceiver);
return client;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy