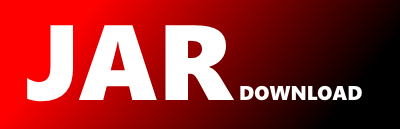
org.robokind.client.basic.RkSpeechConnector Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 Hanson Robokind LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.robokind.client.basic;
import java.net.URISyntaxException;
import javax.jms.Destination;
import javax.jms.JMSException;
import javax.jms.Session;
import org.jflux.api.core.util.EmptyAdapter;
import org.jflux.api.messaging.rk.MessageAsyncReceiver;
import org.jflux.api.messaging.rk.MessageSender;
import org.robokind.api.messaging.services.ServiceCommand;
import org.robokind.api.messaging.services.ServiceError;
import org.robokind.api.speech.SpeechConfig;
import org.robokind.api.speech.SpeechEventList;
import org.robokind.api.speech.SpeechRequest;
import org.robokind.api.speech.messaging.RemoteSpeechServiceClient;
import org.robokind.impl.messaging.services.PortableServiceCommand;
import org.robokind.impl.speech.PortableSpeechRequest;
import org.robokind.client.basic.ConnectionContext.RkServiceConnector;
import org.robokind.impl.messaging.ServiceErrorRecord;
import org.robokind.impl.speech.SpeechEventListRecord;
/**
*
* @author Matthew Stevenson
*/
final class RkSpeechConnector extends RkServiceConnector{
final static String CMD_SENDER = "spCommandSender";
final static String CONFIG_SENDER = "spConfigSender";
final static String ERROR_RECEIVER = "spErrorReceiver";
final static String SPEECH_SENDER = "spRequestSender";
final static String EVENT_RECEIVER = "spEventReceiver";
static RkSpeechConnector theRkSpeechConnector;
private String myCommandDest = "speechCommand";
private String myConfigDest = "speechCommand"; //Same as command
private String myErrorDest = "speechError";
private String myRequestDest = "speechRequest";
private String myEventDest = "speechEvent";
static synchronized RkSpeechConnector getConnector(){
if(theRkSpeechConnector == null){
theRkSpeechConnector = new RkSpeechConnector();
}
return theRkSpeechConnector;
}
@Override
protected synchronized void addConnection(Session session)
throws JMSException, URISyntaxException{
if(myConnectionContext == null || myConnectionsFlag){
return;
}
Destination cmdDest = ConnectionContext.getQueue(myCommandDest);
Destination confDest = ConnectionContext.getQueue(myConfigDest);
Destination errDest = ConnectionContext.getTopic(myErrorDest);
Destination reqDest = ConnectionContext.getQueue(myRequestDest);
Destination evtDest = ConnectionContext.getTopic(myEventDest);
myConnectionContext.addSender(CMD_SENDER, session, cmdDest,
new EmptyAdapter());
myConnectionContext.addSender(CONFIG_SENDER, session, confDest,
new EmptyAdapter());
myConnectionContext.addAsyncReceiver(ERROR_RECEIVER, session, errDest,
ServiceErrorRecord.class, ServiceErrorRecord.SCHEMA$,
new EmptyAdapter());
myConnectionContext.addSender(SPEECH_SENDER, session, reqDest,
new EmptyAdapter());
myConnectionContext.addAsyncReceiver(EVENT_RECEIVER, session, evtDest,
SpeechEventListRecord.class, SpeechEventListRecord.SCHEMA$,
new EmptyAdapter());
myConnectionsFlag = true;
}
synchronized RemoteSpeechServiceClient buildRemoteClient(){
if(myConnectionContext == null || !myConnectionsFlag){
return null;
}
MessageSender cmdSender =
myConnectionContext.getSender(CMD_SENDER);
MessageSender confSender =
myConnectionContext.getSender(CONFIG_SENDER);
MessageAsyncReceiver errReceiver =
myConnectionContext.getAsyncReceiver(ERROR_RECEIVER);
MessageSender reqSender =
myConnectionContext.getSender(SPEECH_SENDER);
MessageAsyncReceiver evtReceiver =
myConnectionContext.getAsyncReceiver(EVENT_RECEIVER);
RemoteSpeechServiceClient client =
new RemoteSpeechServiceClient(SpeechConfig.class,
"speechServiceId", "remoteSpeechServiceId",
cmdSender, confSender, errReceiver,
new PortableServiceCommand.Factory(),
reqSender, evtReceiver,
new PortableSpeechRequest.Factory());
return client;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy