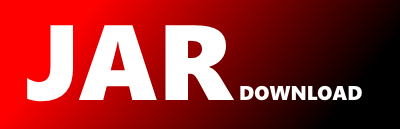
org.robokind.client.basic.Robokind Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2013 Hanson Robokind LLC.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.robokind.client.basic;
import java.util.HashMap;
import java.util.Map;
import java.util.logging.Level;
import java.util.logging.Logger;
import org.robokind.api.animation.Animation;
import org.robokind.api.animation.messaging.RemoteAnimationPlayerClient;
import org.robokind.api.common.utils.TimeUtils;
import org.robokind.api.motion.messaging.RemoteRobot;
import org.robokind.api.motion.messaging.RemoteRobotClient;
import org.robokind.api.sensor.gpio.RemoteGpioServiceClient;
import org.robokind.api.sensor.imu.RemoteAccelerometerServiceClient;
import org.robokind.api.sensor.imu.RemoteCompassServiceClient;
import org.robokind.api.sensor.imu.RemoteGyroscopeServiceClient;
import org.robokind.api.speech.SpeechConfig;
import org.robokind.api.speech.messaging.RemoteSpeechServiceClient;
import org.robokind.api.vision.config.CameraServiceConfig;
import org.robokind.api.vision.config.FaceDetectServiceConfig;
import org.robokind.api.vision.messaging.RemoteImageRegionServiceClient;
import org.robokind.api.vision.messaging.RemoteImageServiceClient;
import org.robokind.impl.animation.xml.AnimationXMLReader;
import org.robokind.impl.animation.xml.XPP3AnimationXMLWriter;
import org.robokind.impl.sensor.HeaderRecord;
/**
* Framework utility methods for the RoboKind Basic API
*
* @author Matthew Stevenson
*/
public final class Robokind {
private final static Logger theLogger = Logger.getLogger(Robokind.class.getName());
private final static Map theConnectionMap = new HashMap();
private final static String theRobotContext = "robotContext";
private final static String theSpeechContext = "speechContext";
private final static String theAnimationContext = "animContext";
private final static String theSensorContext = "sensorContext";
private final static String theCameraContext = "cameraContext";
private final static String theImageRegionContext = "imageRegionContext";
/**
* Connects to the RobokindRobot for communicating with an avatar and robot.
* @return RobokindRobot object for controlling an avatar and robot
*/
public static RemoteRobot connectRobot(){
try{
ConnectionContext context = getContext(theRobotContext);
context.addConnection(RkRobotConnector.getConnector(), UserSettings.getRobotAddress());
RemoteRobotClient robotClient =
RkRobotConnector.getConnector().buildRemoteClient();
context.start();
return new RemoteRobot(robotClient);
}catch(Exception ex){
theLogger.log(Level.SEVERE,
"Unable to connect to Robokind Robot.", ex);
return null;
}
}
/**
* Connects to the animation service.
* @return the animation client
*/
public static RemoteAnimationPlayerClient connectAnimationPlayer(){
try{
ConnectionContext context = getContext(theAnimationContext);
context.addConnection(
RkAnimationConnector.getConnector(),
UserSettings.getAnimationAddress());
context.start();
return RkAnimationConnector.getConnector().buildRemoteClient();
}catch(Exception ex){
theLogger.log(Level.SEVERE,
"Unable to connect to Robokind Sensors.", ex);
return null;
}
}
/**
* Connects to the speech service.
* @return the speech client
*/
public static RemoteSpeechServiceClient connectSpeechService(){
try{
ConnectionContext context = getContext(theSpeechContext);
context.addConnection(RkSpeechConnector.getConnector(),
UserSettings.getSpeechAddress());
RemoteSpeechServiceClient speechClient =
RkSpeechConnector.getConnector().buildRemoteClient();
context.start();
speechClient.start();
return speechClient;
}catch(Exception ex){
theLogger.log(Level.SEVERE,
"Unable to connect to Robokind Robot.", ex);
return null;
}
}
/**
* Connects to the sensor controller.
* @return the sensor client
*/
public static RemoteGpioServiceClient connectSensors(){
try{
ConnectionContext context = getContext(theSensorContext);
context.addConnection(
RkSensorConnector.getConnector(), UserSettings.getSensorAddress());
RemoteGpioServiceClient gpioClient =
RkSensorConnector.getConnector().buildRemoteClient();
context.start();
RkSensorConnector.initializeGpioClient(gpioClient);
return gpioClient;
}catch(Exception ex){
theLogger.log(Level.SEVERE,
"Unable to connect to Robokind Sensors.", ex);
return null;
}
}
/**
* Connects to the accelerometer controller.
* @return the accelerometer client
*/
public static RemoteAccelerometerServiceClient connectAccelerometer(){
try{
ConnectionContext context = getContext(theSensorContext);
context.addConnection(
RkAccelerometerConnector.getConnector(),
UserSettings.getAccelerometerAddress());
RemoteAccelerometerServiceClient accelClient =
RkAccelerometerConnector.getConnector().buildRemoteClient();
context.start();
return accelClient;
}catch(Exception ex){
theLogger.log(Level.SEVERE,
"Unable to connect to Robokind Accelerometer.", ex);
return null;
}
}
/**
* Connects to the gyroscope controller.
* @return the gyroscope client
*/
public static RemoteGyroscopeServiceClient connectGyroscope(){
try{
ConnectionContext context = getContext(theSensorContext);
context.addConnection(
RkGyroscopeConnector.getConnector(),
UserSettings.getGyroscopeAddress());
RemoteGyroscopeServiceClient gyroClient =
RkGyroscopeConnector.getConnector().buildRemoteClient();
context.start();
return gyroClient;
}catch(Exception ex){
theLogger.log(Level.SEVERE,
"Unable to connect to Robokind Gyroscope.", ex);
return null;
}
}
/**
* Connects to the compass controller.
* @return the compass client
*/
public static RemoteCompassServiceClient connectCompass(){
try{
ConnectionContext context = getContext(theSensorContext);
context.addConnection(
RkCompassConnector.getConnector(),
UserSettings.getCompassAddress());
RemoteCompassServiceClient compassClient =
RkCompassConnector.getConnector().buildRemoteClient();
context.start();
return compassClient;
}catch(Exception ex){
theLogger.log(Level.SEVERE,
"Unable to connect to Robokind Compass.", ex);
return null;
}
}
/**
* Connects to the robot's cameras.
* @return RemoteImageServiceClient for controlling the robot's cameras
*/
public static RemoteImageServiceClient connectCameraService(){
try{
ConnectionContext context = getContext(theCameraContext);
context.addConnection(RkCameraConnector.getConnector(),
UserSettings.getCameraAddress());
RemoteImageServiceClient cameraClient =
RkCameraConnector.getConnector().buildRemoteClient();
context.start();
cameraClient.start();
return cameraClient;
}catch(Exception ex){
theLogger.log(Level.SEVERE,
"Unable to connect to Robokind Camera.", ex);
return null;
}
}
/**
* Connects to the robot's face-detection service.
* @return RemoteImageRegionServiceClient for controlling the robot's face detection
*/
public static RemoteImageRegionServiceClient connectImageRegionService(){
try{
ConnectionContext context = getContext(theImageRegionContext);
context.addConnection(RkImageRegionConnector.getConnector(),
UserSettings.getImageRegionAddress());
RemoteImageRegionServiceClient imageRegionClient =
RkImageRegionConnector.getConnector().buildRemoteClient();
context.start();
imageRegionClient.start();
return imageRegionClient;
}catch(Exception ex){
theLogger.log(Level.SEVERE,
"Unable to connect to Robokind image region service.", ex);
return null;
}
}
private static synchronized ConnectionContext getContext(String key){
ConnectionContext cc = theConnectionMap.get(key);
if(cc == null){
cc = new ConnectionContext();
theConnectionMap.put(key, cc);
}
return cc;
}
/**
* Loads an animation from file.
* @param filepath path to the animation
* @return Animation object loaded from the file
*/
public static Animation loadAnimation(String filepath){
try{
return new AnimationXMLReader().readAnimation(filepath);
}catch(Exception ex){
theLogger.log(Level.WARNING, "Unable to load animation.", ex);
return null;
}
}
/**
* Saves an animation to disk.
* @param filepath path to save the animation
* @param anim the animation to save
* @return true if successful, false if failed
*/
public static boolean saveAnimation(String filepath, Animation anim){
try{
new XPP3AnimationXMLWriter().writeAnimation(filepath, anim, null, null);
return true;
}catch(Exception ex){
theLogger.log(Level.WARNING, "Unable to load animation.", ex);
return false;
}
}
/**
* Gets the current time, in Unix format.
* @return the current time
*/
public static long currentTime(){
return TimeUtils.now();
}
/**
* Halts program execution for the given number of milliseconds.
* @param milliseconds number of milliseconds to sleep
*/
public static void sleep(long milliseconds){
try{
Thread.sleep(milliseconds);
}catch(InterruptedException ex){
theLogger.log(Level.WARNING, "Sleep interrupted.", ex);
}
}
/**
* Disconnects from the RobokindRobot.
*/
public static void disconnect(){
for(ConnectionContext cc : theConnectionMap.values()){
cc.stop();
}
theConnectionMap.clear();
RkRobotConnector.clearConnector();
RkAnimationConnector.theRkAnimationConnector = null;
RkSpeechConnector.theRkSpeechConnector = null;
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy