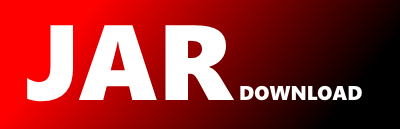
src.android.service.voice.HotwordRejectedResult Maven / Gradle / Ivy
/*
* Copyright (C) 2021 The Android Open Source Project
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package android.service.voice;
import android.annotation.IntDef;
import android.annotation.SystemApi;
import android.os.Parcelable;
import com.android.internal.util.DataClass;
/**
* Represents a result supporting the rejected hotword trigger.
*
* @hide
*/
@DataClass(
genConstructor = false,
genBuilder = true,
genEqualsHashCode = true,
genHiddenConstDefs = true,
genParcelable = true,
genToString = true
)
@SystemApi
public final class HotwordRejectedResult implements Parcelable {
/** No confidence in hotword detector result. */
public static final int CONFIDENCE_LEVEL_NONE = 0;
/** Small confidence in hotword detector result. */
public static final int CONFIDENCE_LEVEL_LOW = 1;
/** Medium confidence in hotword detector result. */
public static final int CONFIDENCE_LEVEL_MEDIUM = 2;
/** High confidence in hotword detector result. */
public static final int CONFIDENCE_LEVEL_HIGH = 3;
/** @hide */
@IntDef(prefix = {"CONFIDENCE_LEVEL_"}, value = {
CONFIDENCE_LEVEL_NONE,
CONFIDENCE_LEVEL_LOW,
CONFIDENCE_LEVEL_MEDIUM,
CONFIDENCE_LEVEL_HIGH
})
@interface HotwordConfidenceLevelValue {
}
/** Confidence level in the trigger outcome. */
@HotwordConfidenceLevelValue
private final int mConfidenceLevel;
private static int defaultConfidenceLevel() {
return CONFIDENCE_LEVEL_NONE;
}
// Code below generated by codegen v1.0.23.
//
// DO NOT MODIFY!
// CHECKSTYLE:OFF Generated code
//
// To regenerate run:
// $ codegen $ANDROID_BUILD_TOP/frameworks/base/core/java/android/service/voice/HotwordRejectedResult.java
//
// To exclude the generated code from IntelliJ auto-formatting enable (one-time):
// Settings > Editor > Code Style > Formatter Control
//@formatter:off
/** @hide */
@IntDef(prefix = "CONFIDENCE_LEVEL_", value = {
CONFIDENCE_LEVEL_NONE,
CONFIDENCE_LEVEL_LOW,
CONFIDENCE_LEVEL_MEDIUM,
CONFIDENCE_LEVEL_HIGH
})
@java.lang.annotation.Retention(java.lang.annotation.RetentionPolicy.SOURCE)
@DataClass.Generated.Member
public @interface ConfidenceLevel {}
/** @hide */
@DataClass.Generated.Member
public static String confidenceLevelToString(@ConfidenceLevel int value) {
switch (value) {
case CONFIDENCE_LEVEL_NONE:
return "CONFIDENCE_LEVEL_NONE";
case CONFIDENCE_LEVEL_LOW:
return "CONFIDENCE_LEVEL_LOW";
case CONFIDENCE_LEVEL_MEDIUM:
return "CONFIDENCE_LEVEL_MEDIUM";
case CONFIDENCE_LEVEL_HIGH:
return "CONFIDENCE_LEVEL_HIGH";
default: return Integer.toHexString(value);
}
}
@DataClass.Generated.Member
/* package-private */ HotwordRejectedResult(
@HotwordConfidenceLevelValue int confidenceLevel) {
this.mConfidenceLevel = confidenceLevel;
com.android.internal.util.AnnotationValidations.validate(
HotwordConfidenceLevelValue.class, null, mConfidenceLevel);
// onConstructed(); // You can define this method to get a callback
}
/**
* Confidence level in the trigger outcome.
*/
@DataClass.Generated.Member
public @HotwordConfidenceLevelValue int getConfidenceLevel() {
return mConfidenceLevel;
}
@Override
@DataClass.Generated.Member
public String toString() {
// You can override field toString logic by defining methods like:
// String fieldNameToString() { ... }
return "HotwordRejectedResult { " +
"confidenceLevel = " + mConfidenceLevel +
" }";
}
@Override
@DataClass.Generated.Member
public boolean equals(@android.annotation.Nullable Object o) {
// You can override field equality logic by defining either of the methods like:
// boolean fieldNameEquals(HotwordRejectedResult other) { ... }
// boolean fieldNameEquals(FieldType otherValue) { ... }
if (this == o) return true;
if (o == null || getClass() != o.getClass()) return false;
@SuppressWarnings("unchecked")
HotwordRejectedResult that = (HotwordRejectedResult) o;
//noinspection PointlessBooleanExpression
return true
&& mConfidenceLevel == that.mConfidenceLevel;
}
@Override
@DataClass.Generated.Member
public int hashCode() {
// You can override field hashCode logic by defining methods like:
// int fieldNameHashCode() { ... }
int _hash = 1;
_hash = 31 * _hash + mConfidenceLevel;
return _hash;
}
@Override
@DataClass.Generated.Member
public void writeToParcel(@android.annotation.NonNull android.os.Parcel dest, int flags) {
// You can override field parcelling by defining methods like:
// void parcelFieldName(Parcel dest, int flags) { ... }
dest.writeInt(mConfidenceLevel);
}
@Override
@DataClass.Generated.Member
public int describeContents() { return 0; }
/** @hide */
@SuppressWarnings({"unchecked", "RedundantCast"})
@DataClass.Generated.Member
/* package-private */ HotwordRejectedResult(@android.annotation.NonNull android.os.Parcel in) {
// You can override field unparcelling by defining methods like:
// static FieldType unparcelFieldName(Parcel in) { ... }
int confidenceLevel = in.readInt();
this.mConfidenceLevel = confidenceLevel;
com.android.internal.util.AnnotationValidations.validate(
HotwordConfidenceLevelValue.class, null, mConfidenceLevel);
// onConstructed(); // You can define this method to get a callback
}
@DataClass.Generated.Member
public static final @android.annotation.NonNull Parcelable.Creator CREATOR
= new Parcelable.Creator() {
@Override
public HotwordRejectedResult[] newArray(int size) {
return new HotwordRejectedResult[size];
}
@Override
public HotwordRejectedResult createFromParcel(@android.annotation.NonNull android.os.Parcel in) {
return new HotwordRejectedResult(in);
}
};
/**
* A builder for {@link HotwordRejectedResult}
*/
@SuppressWarnings("WeakerAccess")
@DataClass.Generated.Member
public static final class Builder {
private @HotwordConfidenceLevelValue int mConfidenceLevel;
private long mBuilderFieldsSet = 0L;
public Builder() {
}
/**
* Confidence level in the trigger outcome.
*/
@DataClass.Generated.Member
public @android.annotation.NonNull Builder setConfidenceLevel(@HotwordConfidenceLevelValue int value) {
checkNotUsed();
mBuilderFieldsSet |= 0x1;
mConfidenceLevel = value;
return this;
}
/** Builds the instance. This builder should not be touched after calling this! */
public @android.annotation.NonNull HotwordRejectedResult build() {
checkNotUsed();
mBuilderFieldsSet |= 0x2; // Mark builder used
if ((mBuilderFieldsSet & 0x1) == 0) {
mConfidenceLevel = defaultConfidenceLevel();
}
HotwordRejectedResult o = new HotwordRejectedResult(
mConfidenceLevel);
return o;
}
private void checkNotUsed() {
if ((mBuilderFieldsSet & 0x2) != 0) {
throw new IllegalStateException(
"This Builder should not be reused. Use a new Builder instance instead");
}
}
}
@DataClass.Generated(
time = 1621961370106L,
codegenVersion = "1.0.23",
sourceFile = "frameworks/base/core/java/android/service/voice/HotwordRejectedResult.java",
inputSignatures = "public static final int CONFIDENCE_LEVEL_NONE\npublic static final int CONFIDENCE_LEVEL_LOW\npublic static final int CONFIDENCE_LEVEL_MEDIUM\npublic static final int CONFIDENCE_LEVEL_HIGH\nprivate final @android.service.voice.HotwordRejectedResult.HotwordConfidenceLevelValue int mConfidenceLevel\nprivate static int defaultConfidenceLevel()\nclass HotwordRejectedResult extends java.lang.Object implements [android.os.Parcelable]\[email protected](genConstructor=false, genBuilder=true, genEqualsHashCode=true, genHiddenConstDefs=true, genParcelable=true, genToString=true)")
@Deprecated
private void __metadata() {}
//@formatter:on
// End of generated code
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy