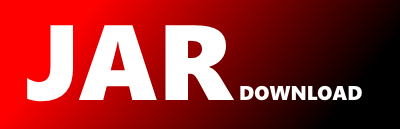
android.app.RobolectricActivityManager Maven / Gradle / Ivy
package android.app;
import android.content.ComponentName;
import android.content.IIntentReceiver;
import android.content.IIntentSender;
import android.content.Intent;
import android.content.IntentFilter;
import android.content.IntentSender;
import android.content.pm.ApplicationInfo;
import android.content.pm.ConfigurationInfo;
import android.content.pm.IPackageDataObserver;
import android.content.pm.ParceledListSlice;
import android.content.pm.UserInfo;
import android.content.res.Configuration;
import android.graphics.Bitmap;
import android.graphics.Point;
import android.graphics.Rect;
import android.net.Uri;
import android.os.Bundle;
import android.os.Debug;
import android.os.IBinder;
import android.os.ParcelFileDescriptor;
import android.app.ActivityManager.RunningTaskInfo;
import android.app.IThumbnailReceiver;
import android.os.RemoteException;
import android.os.StrictMode;
import java.util.List;
/**
* Robolectric implementation of {@link android.app.ActivityManager}.
*/
public class RobolectricActivityManager implements IActivityManager {
@Override
public final int startActivity(IApplicationThread caller, String callingPackage, Intent intent, String resolvedType, IBinder resultTo, String resultWho, int requestCode, int startFlags, String profileFile, ParcelFileDescriptor profileFd, Bundle options) {
return 0;
}
@Override
public final int startActivityAsUser(IApplicationThread caller, String callingPackage, Intent intent, String resolvedType, IBinder resultTo, String resultWho, int requestCode, int startFlags, String profileFile, ParcelFileDescriptor profileFd, Bundle options, int userId) {
return 0;
}
@Override
public final WaitResult startActivityAndWait(IApplicationThread caller, String callingPackage, Intent intent, String resolvedType, IBinder resultTo, String resultWho, int requestCode, int startFlags, String profileFile, ParcelFileDescriptor profileFd, Bundle options, int userId) {
return null;
}
@Override
public int startActivityWithConfig(IApplicationThread caller, String callingPackage, Intent intent, String resolvedType, IBinder resultTo, String resultWho, int requestCode, int startFlags, Configuration newConfig, Bundle options, int userId) throws RemoteException {
return 0;
}
@Override
public int startActivityIntentSender(IApplicationThread caller, IntentSender intent, Intent fillInIntent, String resolvedType, IBinder resultTo, String resultWho, int requestCode, int flagsMask, int flagsValues, Bundle options) throws RemoteException {
return 0;
}
@Override
public boolean startNextMatchingActivity(IBinder callingActivity, Intent intent, Bundle options) throws RemoteException {
return false;
}
@Override
public final boolean finishActivity(IBinder token, int resultCode, Intent resultData) {
return false;
}
@Override
public void finishSubActivity(IBinder token, String resultWho, int requestCode) throws RemoteException {
}
@Override
public boolean finishActivityAffinity(IBinder token) throws RemoteException {
return false;
}
@Override
public boolean willActivityBeVisible(IBinder token) throws RemoteException {
return false;
}
@Override
public Intent registerReceiver(IApplicationThread caller, String callerPackage, IIntentReceiver receiver, IntentFilter filter, String requiredPermission, int userId) throws RemoteException {
return null;
}
@Override
public void unregisterReceiver(IIntentReceiver receiver) throws RemoteException {
}
@Override
public int broadcastIntent(IApplicationThread caller, Intent intent, String resolvedType, IIntentReceiver resultTo, int resultCode, String resultData, Bundle map, String requiredPermission, int appOp, boolean serialized, boolean sticky, int userId) throws RemoteException {
return 0;
}
@Override
public void unbroadcastIntent(IApplicationThread caller, Intent intent, int userId) throws RemoteException {
}
@Override
public void finishReceiver(IBinder who, int resultCode, String resultData, Bundle map, boolean abortBroadcast) throws RemoteException {
}
@Override
public void attachApplication(IApplicationThread app) throws RemoteException {
}
@Override
public void activityResumed(IBinder token) throws RemoteException {
}
@Override
public void activityIdle(IBinder token, Configuration config, boolean stopProfiling) throws RemoteException {
}
@Override
public void activityPaused(IBinder token) throws RemoteException {
}
@Override
public final void activityStopped(IBinder token, Bundle icicle, Bitmap thumbnail,
CharSequence description) {
}
@Override
public void activitySlept(IBinder token) throws RemoteException {
}
@Override
public void activityDestroyed(IBinder token) throws RemoteException {
}
@Override
public String getCallingPackage(IBinder token) throws RemoteException {
return null;
}
@Override
public ComponentName getCallingActivity(IBinder token) throws RemoteException {
return null;
}
@Override
public List getTasks(int maxNum, int flags, IThumbnailReceiver receiver) {
return null;
}
@Override
public List getRecentTasks(int maxNum, int flags, int userId) throws RemoteException {
return null;
}
@Override
public ActivityManager.TaskThumbnails getTaskThumbnails(int id) {
return null;
}
@Override
public Bitmap getTaskTopThumbnail(int id) {
return null;
}
@Override
public List getServices(int maxNum, int flags) throws RemoteException {
return null;
}
@Override
public List getProcessesInErrorState() throws RemoteException {
return null;
}
@Override
public void moveTaskToFront(int task, int flags, Bundle options) throws RemoteException {
}
@Override
public void moveTaskToBack(int task) throws RemoteException {
}
@Override
public boolean moveActivityTaskToBack(IBinder token, boolean nonRoot) throws RemoteException {
return false;
}
@Override
public void moveTaskBackwards(int task) throws RemoteException {
}
@Override
public int getTaskForActivity(IBinder token, boolean onlyRoot) throws RemoteException {
return 0;
}
@Override
public ContentProviderHolder getContentProvider(IApplicationThread caller, String name, int userId, boolean stable) throws RemoteException {
return null;
}
@Override
public ContentProviderHolder getContentProviderExternal(String name, int userId, IBinder token) throws RemoteException {
return null;
}
@Override
public void removeContentProvider(IBinder connection, boolean stable) throws RemoteException {
}
@Override
public void removeContentProviderExternal(String name, IBinder token) throws RemoteException {
}
@Override
public void publishContentProviders(IApplicationThread caller, List providers) throws RemoteException {
}
@Override
public boolean refContentProvider(IBinder connection, int stableDelta, int unstableDelta) throws RemoteException {
return false;
}
@Override
public void unstableProviderDied(IBinder connection) throws RemoteException {
}
@Override
public PendingIntent getRunningServiceControlPanel(ComponentName service) throws RemoteException {
return null;
}
@Override
public ComponentName startService(IApplicationThread caller, Intent service, String resolvedType, int userId) throws RemoteException {
return null;
}
@Override
public int stopService(IApplicationThread caller, Intent service, String resolvedType, int userId) throws RemoteException {
return 0;
}
@Override
public boolean stopServiceToken(ComponentName className, IBinder token, int startId) throws RemoteException {
return false;
}
@Override
public void setServiceForeground(ComponentName className, IBinder token, int id, Notification notification, boolean keepNotification) throws RemoteException {
}
@Override
public int bindService(IApplicationThread caller, IBinder token, Intent service, String resolvedType, IServiceConnection connection, int flags, int userId) throws RemoteException {
return 0;
}
@Override
public boolean unbindService(IServiceConnection connection) throws RemoteException {
return false;
}
@Override
public void publishService(IBinder token, Intent intent, IBinder service) throws RemoteException {
}
@Override
public void unbindFinished(IBinder token, Intent service, boolean doRebind) throws RemoteException {
}
@Override
public void serviceDoneExecuting(IBinder token, int type, int startId, int res) throws RemoteException {
}
@Override
public IBinder peekService(Intent service, String resolvedType) throws RemoteException {
return null;
}
@Override
public boolean bindBackupAgent(ApplicationInfo appInfo, int backupRestoreMode) throws RemoteException {
return false;
}
@Override
public void clearPendingBackup() throws RemoteException {
}
@Override
public void backupAgentCreated(String packageName, IBinder agent) throws RemoteException {
}
@Override
public void unbindBackupAgent(ApplicationInfo appInfo) throws RemoteException {
}
@Override
public void killApplicationProcess(String processName, int uid) throws RemoteException {
}
@Override
public boolean startInstrumentation(ComponentName className, String profileFile, int flags, Bundle arguments, IInstrumentationWatcher watcher, IUiAutomationConnection uiAutomationConnection, int userId) {
return false;
}
@Override
public void finishInstrumentation(IApplicationThread target, int resultCode, Bundle results) throws RemoteException {
}
@Override
public Configuration getConfiguration() throws RemoteException {
return null;
}
@Override
public void updateConfiguration(Configuration values) throws RemoteException {
}
@Override
public void setRequestedOrientation(IBinder token, int requestedOrientation) throws RemoteException {
}
@Override
public int getRequestedOrientation(IBinder token) throws RemoteException {
return 0;
}
@Override
public ComponentName getActivityClassForToken(IBinder token) throws RemoteException {
return null;
}
@Override
public String getPackageForToken(IBinder token) throws RemoteException {
return null;
}
@Override
public IIntentSender getIntentSender(int type, String packageName, IBinder token, String resultWho, int requestCode, Intent[] intents, String[] resolvedTypes, int flags, Bundle options, int userId) throws RemoteException {
return null;
}
@Override
public void cancelIntentSender(IIntentSender sender) throws RemoteException {
}
@Override
public boolean clearApplicationUserData(String packageName, IPackageDataObserver observer, int userId) throws RemoteException {
return false;
}
@Override
public String getPackageForIntentSender(IIntentSender sender) throws RemoteException {
return null;
}
@Override
public int getUidForIntentSender(IIntentSender sender) throws RemoteException {
return 0;
}
@Override
public int handleIncomingUser(int callingPid, int callingUid, int userId, boolean allowAll, boolean requireFull, String name, String callerPackage) throws RemoteException {
return 0;
}
@Override
public void setProcessLimit(int max) throws RemoteException {
}
@Override
public int getProcessLimit() throws RemoteException {
return 0;
}
@Override
public void setProcessForeground(IBinder token, int pid, boolean isForeground) throws RemoteException {
}
@Override
public int checkPermission(String permission, int pid, int uid) throws RemoteException {
return 0;
}
@Override
public int checkUriPermission(Uri uri, int pid, int uid, int modeFlags) {
return 0;
}
@Override
public void grantUriPermission(IApplicationThread caller, String targetPkg, Uri uri, int modeFlags) {
}
@Override
public void revokeUriPermission(IApplicationThread caller, Uri uri, int modeFlags) {
}
@Override
public void showWaitingForDebugger(IApplicationThread who, boolean waiting) throws RemoteException {
}
@Override
public void getMemoryInfo(ActivityManager.MemoryInfo outInfo) throws RemoteException {
}
@Override
public void killBackgroundProcesses(String packageName, int userId) throws RemoteException {
}
@Override
public void killAllBackgroundProcesses() throws RemoteException {
}
@Override
public void forceStopPackage(String packageName, int userId) throws RemoteException {
}
@Override
public void setLockScreenShown(boolean shown) throws RemoteException {
}
@Override
public void unhandledBack() throws RemoteException {
}
@Override
public ParcelFileDescriptor openContentUri(Uri uri) throws RemoteException {
return null;
}
@Override
public void setDebugApp(String packageName, boolean waitForDebugger, boolean persistent) throws RemoteException {
}
@Override
public void setAlwaysFinish(boolean enabled) throws RemoteException {
}
@Override
public void setActivityController(IActivityController watcher) throws RemoteException {
}
@Override
public void enterSafeMode() throws RemoteException {
}
@Override
public void noteWakeupAlarm(IIntentSender sender) {
}
@Override
public boolean killPids(int[] pids, String reason, boolean secure) throws RemoteException {
return false;
}
@Override
public boolean killProcessesBelowForeground(String reason) throws RemoteException {
return false;
}
@Override
public void handleApplicationCrash(IBinder app, ApplicationErrorReport.CrashInfo crashInfo) throws RemoteException {
}
@Override
public boolean handleApplicationWtf(IBinder app, String tag, ApplicationErrorReport.CrashInfo crashInfo) {
return false;
}
@Override
public void handleApplicationStrictModeViolation(IBinder app, int violationMask, StrictMode.ViolationInfo crashInfo) throws RemoteException {
}
@Override
public void signalPersistentProcesses(int signal) throws RemoteException {
}
@Override
public List getRunningAppProcesses() throws RemoteException {
return null;
}
@Override
public List getRunningExternalApplications() throws RemoteException {
return null;
}
@Override
public void getMyMemoryState(ActivityManager.RunningAppProcessInfo outInfo) throws RemoteException {
}
@Override
public ConfigurationInfo getDeviceConfigurationInfo() throws RemoteException {
return null;
}
@Override
public boolean profileControl(String process, int userId, boolean start, String path, ParcelFileDescriptor fd, int profileType) throws RemoteException {
return false;
}
@Override
public boolean shutdown(int timeout) throws RemoteException {
return false;
}
@Override
public void stopAppSwitches() throws RemoteException {
}
@Override
public void resumeAppSwitches() throws RemoteException {
}
@Override
public void killApplicationWithAppId(String pkg, int appid) throws RemoteException {
}
@Override
public void closeSystemDialogs(String reason) throws RemoteException {
}
@Override
public Debug.MemoryInfo[] getProcessMemoryInfo(int[] pids) throws RemoteException {
return new Debug.MemoryInfo[0];
}
@Override
public void overridePendingTransition(IBinder token, String packageName, int enterAnim, int exitAnim) throws RemoteException {
}
@Override
public boolean isUserAMonkey() throws RemoteException {
return false;
}
@Override
public void setUserIsMonkey(boolean monkey) throws RemoteException {
}
@Override
public void finishHeavyWeightApp() throws RemoteException {
}
@Override
public void setImmersive(IBinder token, boolean immersive) throws RemoteException {
}
@Override
public boolean isImmersive(IBinder token) throws RemoteException {
return false;
}
@Override
public boolean isTopActivityImmersive() throws RemoteException {
return false;
}
@Override
public void crashApplication(int uid, int initialPid, String packageName, String message) throws RemoteException {
}
@Override
public String getProviderMimeType(Uri uri, int userId) throws RemoteException {
return null;
}
@Override
public IBinder newUriPermissionOwner(String name) throws RemoteException {
return null;
}
@Override
public void grantUriPermissionFromOwner(IBinder token, int fromUid, String targetPkg, Uri uri, int modeFlags) {
}
@Override
public int checkGrantUriPermission(int callingUid, String targetPkg, Uri uri, int modeFlags) {
return 0;
}
@Override
public void revokeUriPermissionFromOwner(IBinder token, Uri uri, int mode) {
}
@Override
public boolean dumpHeap(String process, int userId, boolean managed, String path, ParcelFileDescriptor fd) throws RemoteException {
return false;
}
@Override
public int startActivities(IApplicationThread caller, String callingPackage, Intent[] intents, String[] resolvedTypes, IBinder resultTo, Bundle options, int userId) throws RemoteException {
return 0;
}
@Override
public int getFrontActivityScreenCompatMode() throws RemoteException {
return 0;
}
@Override
public void setFrontActivityScreenCompatMode(int mode) throws RemoteException {
}
@Override
public int getPackageScreenCompatMode(String packageName) throws RemoteException {
return 0;
}
@Override
public void setPackageScreenCompatMode(String packageName, int mode) throws RemoteException {
}
@Override
public boolean getPackageAskScreenCompat(String packageName) throws RemoteException {
return false;
}
@Override
public void setPackageAskScreenCompat(String packageName, boolean ask) throws RemoteException {
}
@Override
public boolean switchUser(int userid) throws RemoteException {
return false;
}
@Override
public int stopUser(int userid, IStopUserCallback callback) throws RemoteException {
return 0;
}
@Override
public UserInfo getCurrentUser() throws RemoteException {
return null;
}
@Override
public boolean isUserRunning(int userid, boolean orStopping) throws RemoteException {
return false;
}
@Override
public int[] getRunningUserIds() throws RemoteException {
return new int[0];
}
@Override
public void registerProcessObserver(IProcessObserver observer) throws RemoteException {
}
@Override
public void unregisterProcessObserver(IProcessObserver observer) throws RemoteException {
}
@Override
public boolean isIntentSenderTargetedToPackage(IIntentSender sender) throws RemoteException {
return false;
}
@Override
public boolean isIntentSenderAnActivity(IIntentSender sender) throws RemoteException {
return false;
}
@Override
public Intent getIntentForIntentSender(IIntentSender sender) throws RemoteException {
return null;
}
@Override
public void updatePersistentConfiguration(Configuration values) throws RemoteException {
}
@Override
public long[] getProcessPss(int[] pids) throws RemoteException {
return new long[0];
}
@Override
public void showBootMessage(CharSequence msg, boolean always) throws RemoteException {
}
@Override
public boolean navigateUpTo(IBinder token, Intent target, int resultCode, Intent resultData) throws RemoteException {
return false;
}
@Override
public int getLaunchedFromUid(IBinder activityToken) throws RemoteException {
return 0;
}
@Override
public String getLaunchedFromPackage(IBinder activityToken) throws RemoteException {
return null;
}
@Override
public void registerUserSwitchObserver(IUserSwitchObserver observer) throws RemoteException {
}
@Override
public void unregisterUserSwitchObserver(IUserSwitchObserver observer) throws RemoteException {
}
@Override
public void requestBugReport() throws RemoteException {
}
@Override
public long inputDispatchingTimedOut(int pid, boolean aboveSystem) throws RemoteException {
return 0;
}
@Override
public void killUid(int uid, String reason) throws RemoteException {
}
@Override
public void hang(IBinder who, boolean allowRestart) throws RemoteException {
}
@Override
public boolean testIsSystemReady() {
return false;
}
@Override
public IBinder asBinder() {
return null;
}
@Override
public boolean removeTask(int taskId, int flags) throws RemoteException {
return false;
}
@Override
public boolean targetTaskAffinityMatchesActivity(IBinder token, String destAffinity) {
return false;
}
@Override
public void dismissKeyguardOnNextActivity() {
}
@Override
public boolean removeSubTask(int taskId, int subTaskIndex) {
return false;
}
public final void startRunning(String pkg, String cls, String action, String data) {
}
@Override
public void wakingUp() {
}
@Override
public void goingToSleep() {
}
@Override
public void reportThumbnail(IBinder token, Bitmap thumbnail, CharSequence description) {
}
@Override
public Bundle getTopActivityExtras(int requestType) throws RemoteException {
return null;
}
@Override
public void reportTopActivityExtras(IBinder token, Bundle extras) throws RemoteException {
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy