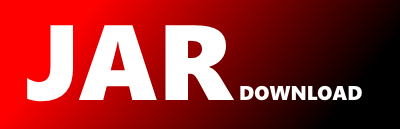
org.robolectric.shadows.ShadowCountDownTimer Maven / Gradle / Ivy
package org.robolectric.shadows;
import android.os.CountDownTimer;
import org.robolectric.annotation.Implementation;
import org.robolectric.annotation.Implements;
import org.robolectric.annotation.RealObject;
/**
* Shadow for {@link android.os.CountDownTimer}.
*/
@Implements(CountDownTimer.class)
public class ShadowCountDownTimer {
private boolean started;
private long countDownInterval;
private long millisInFuture;
@RealObject CountDownTimer countDownTimer;
public void __constructor__(long millisInFuture, long countDownInterval) {
this.countDownInterval = countDownInterval;
this.millisInFuture = millisInFuture;
this.started = false;
}
@Implementation
public final synchronized CountDownTimer start() {
started = true;
return countDownTimer;
}
@Implementation
public final void cancel() {
started = false;
}
public void invokeTick(long millisUntilFinished) {
countDownTimer.onTick(millisUntilFinished);
}
public void invokeFinish() {
countDownTimer.onFinish();
}
public boolean hasStarted() {
return started;
}
public long getCountDownInterval() {
return countDownInterval;
}
public long getMillisInFuture() {
return millisInFuture;
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy