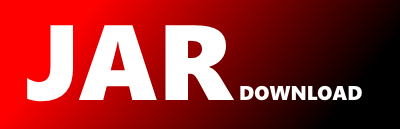
org.robolectric.shadows.ShadowMessage Maven / Gradle / Ivy
package org.robolectric.shadows;
import android.os.Handler;
import android.os.Message;
import javax.annotation.Generated;
import org.robolectric.annotation.Implementation;
import org.robolectric.annotation.Implements;
import org.robolectric.annotation.RealObject;
import org.robolectric.annotation.Resetter;
import static org.robolectric.Shadows.shadowOf;
import static org.robolectric.internal.Shadow.*;
import static org.robolectric.util.ReflectionHelpers.*;
/**
* Shadow for {@link android.os.Message}.
*/
@Implements(Message.class)
public class ShadowMessage {
@RealObject
private Message realMessage;
private Runnable scheduledRunnable;
private static final Object lock = getStaticField(Message.class, "sPoolSync");
private void unschedule() {
Handler target = realMessage.getTarget();
if (target != null && scheduledRunnable != null) {
shadowOf(target.getLooper()).getScheduler().remove(scheduledRunnable);
scheduledRunnable = null;
}
}
@Implementation
/**
* Hook to unscheduled the callback when the message is recycled.
* Invokes {@link #unschedule()} and then calls through to
* {@link Message#recycle()} on the real object.
*/
public void recycle() {
unschedule();
directlyOn(realMessage, Message.class, "recycle");
}
/**
* Invokes {@link #recycle()}. Provided for forward compatibility
* with API 21.
*/
public void recycleUnchecked() {
recycle();
}
/**
* Stores the Runnable
instance that has been scheduled
* to invoke this message. This is called when the message is
* enqueued by {@link ShadowMessageQueue#enqueueMessage} and is used when
* the message is recycled to ensure that the correct
* {@link Runnable} instance is removed from the associated scheduler.
*
* @param r the Runnable
instance that is scheduled to
* trigger this message.
*
* @see #recycle()
*/
public void setScheduledRunnable(Runnable r) {
scheduledRunnable = r;
}
@Implementation
/**
* Convenience method to provide access to the private Message.isInUse()
* method. Note that the definition of "in use" changed with API 21:
*
* In API 19, a message was only considered "in use" during its dispatch. In API 21, the
* message is considered "in use" from the time it is enqueued until the time that
* it is freshly obtained via a call to {@link Message#obtain()}. This means that
* in API 21 messages that are in the recycled pool will still be marked as "in use".
*
* @return true
if the message is currently "in use", false
* otherwise.
*/
public boolean isInUse() {
return directlyOn(realMessage, Message.class, "isInUse");
}
/**
* Convenience method to provide getter access to the private field
* Message.next
.
*
* @return The next message in the current message chain.
* @see #setNext(Message)
*/
public Message getNext() {
return getField(realMessage, "next");
}
/**
* Convenience method to provide setter access to the private field
* Message.next
.
*
* @param next the new next message for the current message.
* @see #getNext()
*/
public void setNext(Message next) {
setField(realMessage, "next", next);
}
/**
* Resets the static state of the {@link Message} class by
* emptying the message pool.
*/
@Resetter
public static void reset() {
synchronized (lock) {
setStaticField(Message.class, "sPoolSize", 0);
setStaticField(Message.class, "sPool", null);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy