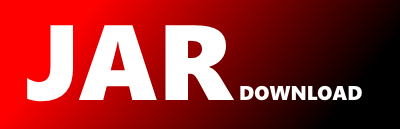
org.robolectric.shadows.AutoValue_ShadowBluetoothManager_BleDevice Maven / Gradle / Ivy
package org.robolectric.shadows;
import android.bluetooth.BluetoothDevice;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ShadowBluetoothManager_BleDevice extends ShadowBluetoothManager.BleDevice {
private final int profile;
private final int state;
private final BluetoothDevice device;
private AutoValue_ShadowBluetoothManager_BleDevice(
int profile,
int state,
BluetoothDevice device) {
this.profile = profile;
this.state = state;
this.device = device;
}
@Override
int profile() {
return profile;
}
@Override
int state() {
return state;
}
@Override
BluetoothDevice device() {
return device;
}
@Override
public String toString() {
return "BleDevice{"
+ "profile=" + profile + ", "
+ "state=" + state + ", "
+ "device=" + device
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ShadowBluetoothManager.BleDevice) {
ShadowBluetoothManager.BleDevice that = (ShadowBluetoothManager.BleDevice) o;
return this.profile == that.profile()
&& this.state == that.state()
&& this.device.equals(that.device());
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= profile;
h$ *= 1000003;
h$ ^= state;
h$ *= 1000003;
h$ ^= device.hashCode();
return h$;
}
static final class Builder extends ShadowBluetoothManager.BleDevice.Builder {
private int profile;
private int state;
private BluetoothDevice device;
private byte set$0;
Builder() {
}
@Override
ShadowBluetoothManager.BleDevice.Builder setProfile(int profile) {
this.profile = profile;
set$0 |= 1;
return this;
}
@Override
ShadowBluetoothManager.BleDevice.Builder setState(int state) {
this.state = state;
set$0 |= 2;
return this;
}
@Override
ShadowBluetoothManager.BleDevice.Builder setDevice(BluetoothDevice device) {
if (device == null) {
throw new NullPointerException("Null device");
}
this.device = device;
return this;
}
@Override
ShadowBluetoothManager.BleDevice build() {
if (set$0 != 3
|| this.device == null) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" profile");
}
if ((set$0 & 2) == 0) {
missing.append(" state");
}
if (this.device == null) {
missing.append(" device");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ShadowBluetoothManager_BleDevice(
this.profile,
this.state,
this.device);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy