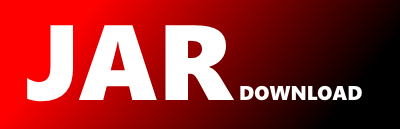
org.robolectric.shadows.AutoValue_ShadowCompanionDeviceManager_RoboAssociationInfo Maven / Gradle / Ivy
package org.robolectric.shadows;
import androidx.annotation.Nullable;
import javax.annotation.Generated;
@Generated("com.google.auto.value.processor.AutoValueProcessor")
final class AutoValue_ShadowCompanionDeviceManager_RoboAssociationInfo extends ShadowCompanionDeviceManager.RoboAssociationInfo {
private final int id;
private final int userId;
private final String packageName;
private final String deviceMacAddress;
private final CharSequence displayName;
private final String deviceProfile;
private final boolean selfManaged;
private final boolean notifyOnDeviceNearby;
private final long timeApprovedMs;
private final long lastTimeConnectedMs;
private AutoValue_ShadowCompanionDeviceManager_RoboAssociationInfo(
int id,
int userId,
@Nullable String packageName,
@Nullable String deviceMacAddress,
@Nullable CharSequence displayName,
@Nullable String deviceProfile,
boolean selfManaged,
boolean notifyOnDeviceNearby,
long timeApprovedMs,
long lastTimeConnectedMs) {
this.id = id;
this.userId = userId;
this.packageName = packageName;
this.deviceMacAddress = deviceMacAddress;
this.displayName = displayName;
this.deviceProfile = deviceProfile;
this.selfManaged = selfManaged;
this.notifyOnDeviceNearby = notifyOnDeviceNearby;
this.timeApprovedMs = timeApprovedMs;
this.lastTimeConnectedMs = lastTimeConnectedMs;
}
@Override
public int id() {
return id;
}
@Override
public int userId() {
return userId;
}
@Nullable
@Override
public String packageName() {
return packageName;
}
@Nullable
@Override
public String deviceMacAddress() {
return deviceMacAddress;
}
@Nullable
@Override
public CharSequence displayName() {
return displayName;
}
@Nullable
@Override
public String deviceProfile() {
return deviceProfile;
}
@Override
public boolean selfManaged() {
return selfManaged;
}
@Override
public boolean notifyOnDeviceNearby() {
return notifyOnDeviceNearby;
}
@Override
public long timeApprovedMs() {
return timeApprovedMs;
}
@Override
public long lastTimeConnectedMs() {
return lastTimeConnectedMs;
}
@Override
public String toString() {
return "RoboAssociationInfo{"
+ "id=" + id + ", "
+ "userId=" + userId + ", "
+ "packageName=" + packageName + ", "
+ "deviceMacAddress=" + deviceMacAddress + ", "
+ "displayName=" + displayName + ", "
+ "deviceProfile=" + deviceProfile + ", "
+ "selfManaged=" + selfManaged + ", "
+ "notifyOnDeviceNearby=" + notifyOnDeviceNearby + ", "
+ "timeApprovedMs=" + timeApprovedMs + ", "
+ "lastTimeConnectedMs=" + lastTimeConnectedMs
+ "}";
}
@Override
public boolean equals(Object o) {
if (o == this) {
return true;
}
if (o instanceof ShadowCompanionDeviceManager.RoboAssociationInfo) {
ShadowCompanionDeviceManager.RoboAssociationInfo that = (ShadowCompanionDeviceManager.RoboAssociationInfo) o;
return this.id == that.id()
&& this.userId == that.userId()
&& (this.packageName == null ? that.packageName() == null : this.packageName.equals(that.packageName()))
&& (this.deviceMacAddress == null ? that.deviceMacAddress() == null : this.deviceMacAddress.equals(that.deviceMacAddress()))
&& (this.displayName == null ? that.displayName() == null : this.displayName.equals(that.displayName()))
&& (this.deviceProfile == null ? that.deviceProfile() == null : this.deviceProfile.equals(that.deviceProfile()))
&& this.selfManaged == that.selfManaged()
&& this.notifyOnDeviceNearby == that.notifyOnDeviceNearby()
&& this.timeApprovedMs == that.timeApprovedMs()
&& this.lastTimeConnectedMs == that.lastTimeConnectedMs();
}
return false;
}
@Override
public int hashCode() {
int h$ = 1;
h$ *= 1000003;
h$ ^= id;
h$ *= 1000003;
h$ ^= userId;
h$ *= 1000003;
h$ ^= (packageName == null) ? 0 : packageName.hashCode();
h$ *= 1000003;
h$ ^= (deviceMacAddress == null) ? 0 : deviceMacAddress.hashCode();
h$ *= 1000003;
h$ ^= (displayName == null) ? 0 : displayName.hashCode();
h$ *= 1000003;
h$ ^= (deviceProfile == null) ? 0 : deviceProfile.hashCode();
h$ *= 1000003;
h$ ^= selfManaged ? 1231 : 1237;
h$ *= 1000003;
h$ ^= notifyOnDeviceNearby ? 1231 : 1237;
h$ *= 1000003;
h$ ^= (int) ((timeApprovedMs >>> 32) ^ timeApprovedMs);
h$ *= 1000003;
h$ ^= (int) ((lastTimeConnectedMs >>> 32) ^ lastTimeConnectedMs);
return h$;
}
static final class Builder extends ShadowCompanionDeviceManager.RoboAssociationInfo.Builder {
private int id;
private int userId;
private String packageName;
private String deviceMacAddress;
private CharSequence displayName;
private String deviceProfile;
private boolean selfManaged;
private boolean notifyOnDeviceNearby;
private long timeApprovedMs;
private long lastTimeConnectedMs;
private byte set$0;
Builder() {
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo.Builder setId(int id) {
this.id = id;
set$0 |= 1;
return this;
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo.Builder setUserId(int userId) {
this.userId = userId;
set$0 |= 2;
return this;
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo.Builder setPackageName(String packageName) {
this.packageName = packageName;
return this;
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo.Builder setDeviceMacAddress(String deviceMacAddress) {
this.deviceMacAddress = deviceMacAddress;
return this;
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo.Builder setDisplayName(CharSequence displayName) {
this.displayName = displayName;
return this;
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo.Builder setDeviceProfile(String deviceProfile) {
this.deviceProfile = deviceProfile;
return this;
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo.Builder setSelfManaged(boolean selfManaged) {
this.selfManaged = selfManaged;
set$0 |= 4;
return this;
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo.Builder setNotifyOnDeviceNearby(boolean notifyOnDeviceNearby) {
this.notifyOnDeviceNearby = notifyOnDeviceNearby;
set$0 |= 8;
return this;
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo.Builder setTimeApprovedMs(long timeApprovedMs) {
this.timeApprovedMs = timeApprovedMs;
set$0 |= 0x10;
return this;
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo.Builder setLastTimeConnectedMs(long lastTimeConnectedMs) {
this.lastTimeConnectedMs = lastTimeConnectedMs;
set$0 |= 0x20;
return this;
}
@Override
public ShadowCompanionDeviceManager.RoboAssociationInfo build() {
if (set$0 != 0x3f) {
StringBuilder missing = new StringBuilder();
if ((set$0 & 1) == 0) {
missing.append(" id");
}
if ((set$0 & 2) == 0) {
missing.append(" userId");
}
if ((set$0 & 4) == 0) {
missing.append(" selfManaged");
}
if ((set$0 & 8) == 0) {
missing.append(" notifyOnDeviceNearby");
}
if ((set$0 & 0x10) == 0) {
missing.append(" timeApprovedMs");
}
if ((set$0 & 0x20) == 0) {
missing.append(" lastTimeConnectedMs");
}
throw new IllegalStateException("Missing required properties:" + missing);
}
return new AutoValue_ShadowCompanionDeviceManager_RoboAssociationInfo(
this.id,
this.userId,
this.packageName,
this.deviceMacAddress,
this.displayName,
this.deviceProfile,
this.selfManaged,
this.notifyOnDeviceNearby,
this.timeApprovedMs,
this.lastTimeConnectedMs);
}
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy