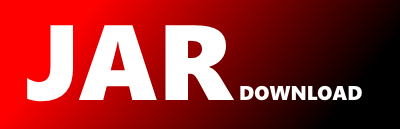
org.robolectric.shadows.ShadowSystemHealthManager Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shadows-framework Show documentation
Show all versions of shadows-framework Show documentation
An alternative Android testing framework.
The newest version!
package org.robolectric.shadows;
import static android.os.Build.VERSION_CODES.N;
import android.os.Process;
import android.os.health.HealthStats;
import android.os.health.SystemHealthManager;
import java.util.HashMap;
import org.robolectric.annotation.Implementation;
import org.robolectric.annotation.Implements;
import org.robolectric.annotation.Resetter;
/** Shadow for {@link android.os.health.SystemHealthManager} */
@Implements(value = SystemHealthManager.class, minSdk = N)
public class ShadowSystemHealthManager {
private static final HealthStats DEFAULT_HEALTH_STATS =
HealthStatsBuilder.newBuilder().setDataType("default_health_stats").build();
private static final HashMap uidToHealthStats = new HashMap<>();
@Resetter
public static void reset() {
uidToHealthStats.clear();
}
@Implementation
protected HealthStats takeMyUidSnapshot() {
return takeUidSnapshot(Process.myUid());
}
@Implementation
protected HealthStats takeUidSnapshot(int uid) {
return uidToHealthStats.getOrDefault(uid, DEFAULT_HEALTH_STATS);
}
@Implementation
protected HealthStats[] takeUidSnapshots(int[] uids) {
HealthStats[] stats = new HealthStats[uids.length];
for (int i = 0; i < uids.length; i++) {
stats[i] = takeUidSnapshot(uids[i]);
}
return stats;
}
/**
* Add {@link HealthStats} for the given UID. Calling {@link SystemHealthManager#takeUidSnapshot}
* with the given UID will return this HealthStats object.
*/
public void addHealthStatsForUid(int uid, HealthStats stats) {
uidToHealthStats.put(uid, stats);
}
/** The same as {@code addHealthStatsForUid(android.os.Process.myUid(), stats)}. */
public void addHealthStats(HealthStats stats) {
addHealthStatsForUid(Process.myUid(), stats);
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy