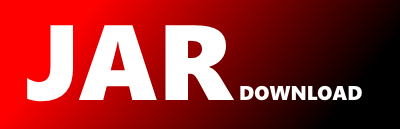
org.robolectric.shadows.ShadowVMRuntime Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of shadows-framework Show documentation
Show all versions of shadows-framework Show documentation
An alternative Android testing framework.
The newest version!
package org.robolectric.shadows;
import static android.os.Build.VERSION_CODES.Q;
import com.google.common.base.Preconditions;
import dalvik.system.VMRuntime;
import java.lang.ref.WeakReference;
import java.lang.reflect.Array;
import java.lang.reflect.Method;
import java.nio.ByteBuffer;
import java.nio.ByteOrder;
import java.util.Collections;
import java.util.HashMap;
import java.util.Map;
import java.util.WeakHashMap;
import java.util.concurrent.atomic.AtomicLong;
import javax.annotation.Nullable;
import org.robolectric.annotation.Implementation;
import org.robolectric.annotation.Implements;
import org.robolectric.annotation.Resetter;
@Implements(value = VMRuntime.class, isInAndroidSdk = false)
public class ShadowVMRuntime {
/**
* A map of allocated non movable arrays to the (Direct)ByteBuffer backing it
*
* The JVM does not directly support newNonMovableArray. So as a workaround, this class will
* allocate a direct ByteBuffer for use in native code. It is the responsibility the shadow code
* to update any associated buffers with the data from native code.
*/
private final Map
© 2015 - 2025 Weber Informatics LLC | Privacy Policy