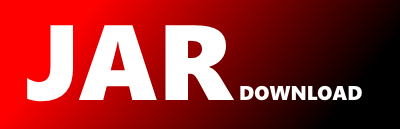
org.rootservices.otter.dispatch.config.DispatchAppFactory Maven / Gradle / Ivy
Show all versions of otter Show documentation
package org.rootservices.otter.dispatch.config;
import org.rootservices.otter.controller.entity.DefaultUser;
import org.rootservices.otter.dispatch.JsonErrorHandler;
import org.rootservices.otter.dispatch.JsonRouteRun;
import org.rootservices.otter.dispatch.RouteRunner;
import org.rootservices.otter.dispatch.translator.RestErrorHandler;
import org.rootservices.otter.dispatch.translator.rest.*;
import org.rootservices.otter.gateway.entity.rest.RestError;
import org.rootservices.otter.gateway.entity.rest.RestErrorTarget;
import org.rootservices.otter.router.builder.RestRouteBuilder;
import org.rootservices.otter.router.entity.RestRoute;
import org.rootservices.otter.translatable.Translatable;
import org.rootservices.otter.translator.JsonTranslator;
import org.rootservices.otter.translator.config.TranslatorAppFactory;
import java.util.HashMap;
public class DispatchAppFactory {
private static TranslatorAppFactory translatorAppFactory = new TranslatorAppFactory();
public RestRoute makeRestRoute(RestErrorTarget from) {
return new RestRouteBuilder()
.restResource(from.getResource())
.before(from.getBefore())
.after(from.getAfter())
.build();
}
public RouteRunner makeJsonRouteRun(RestRoute restRoute, Class extends Translatable> payload) {
Class castedPayload = toPayload(payload);
JsonTranslator
jsonTranslator = translatorAppFactory.jsonTranslator(castedPayload);
RestRequestTranslator restRequestTranslator = new RestRequestTranslator();
RestResponseTranslator
restResponseTranslator = new RestResponseTranslator
();
RestBtwnRequestTranslator restBtwnRequestTranslator = new RestBtwnRequestTranslator<>();
RestBtwnResponseTranslator
restBtwnResponseTranslator = new RestBtwnResponseTranslator<>();
RestRoute castedRestRoute = toRestRoute(restRoute);
return new JsonRouteRun(
castedRestRoute,
restResponseTranslator,
restRequestTranslator,
restBtwnRequestTranslator,
restBtwnResponseTranslator,
jsonTranslator,
new HashMap<>(),
new RestErrorRequestTranslator<>(),
new RestErrorResponseTranslator()
);
}
@SuppressWarnings("unchecked")
protected
Class
toPayload(Class extends Translatable> from) {
return (Class
) from;
}
@SuppressWarnings("unchecked")
protected RestRoute toRestRoute(RestRoute from) {
return (RestRoute) from;
}
public RestErrorHandler restErrorHandler(RestError restError) {
RestError castedRestErrorValue = toRestError(restError);
JsonTranslator
jsonTranslator = translatorAppFactory.jsonTranslator(castedRestErrorValue.getPayload());
return new JsonErrorHandler(
jsonTranslator,
castedRestErrorValue.getRestResource(),
new RestRequestTranslator<>(),
new RestResponseTranslator<>()
);
}
@SuppressWarnings("unchecked")
public RestError toRestError(RestError restError) {
return (RestError) restError;
}
}