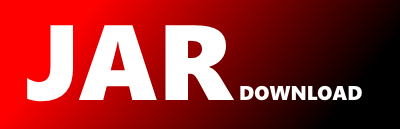
org.rribbit.RequestResponseBus Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of rribbit Show documentation
Show all versions of rribbit Show documentation
RRiBbit is an Open Source Java application framework that eliminates dependencies and simplifies code structure. It can be used as an Eventbus, but improves upon
this by being compatible with existing code and allowing bidirectional communication between components. It also supports Remoting, so that you can use Eventbuses that
run on other machines, complete with failover, loadbalancing and SSL/TLS support.
/**
* Copyright (C) 2012-2018 RRiBbit.org
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.rribbit;
import java.util.Collection;
/**
* The bus to which requests can be sent and responses received. The following rules apply to all methods of this interface:
*
*
* - When you send a request, ALL {@link Listener}s that match the request are executed, even if you only send for a single result
* - The order in which the {@link Listener}s are executed is not specified
* - The order in which the results are returned is not specified and does not necessarily match the execution order of the {@link Listener}s
* - If exactly one of the {@link Listener}s throws a {@link Throwable}, then that {@link Throwable} is thrown
* - If multiple {@link Listener}s throw a {@link Throwable}, then a {@link MultipleThrowablesOccurredException} is thrown, containing all the {@link Throwable}s that were thrown
* - If any method parameter is 'null', then an {@link IllegalArgumentException} is thrown (null values may occur in the 'parameters' varargs array, however)
*
*
* @author G.J. Schouten
*
*/
public interface RequestResponseBus {
/**
* Send a request to all {@link Listener}s that satisfy the following requirements.
*
* - They return an {@link Object} that the parameter 'returnType' is assignable from and
* - they belong to a method with parameters that match the given 'parameters' objects
*
*
* @param returnType the return type that has to be assignable from the return type of the {@link Listener}s
* @param parameters the parameters to give to the {@link Listener}s
* @return a single return value from one of the listeners that executed (which one is unspecified) or 'null' if no matching {@link Listener}s were found
*/
T sendForSingleOfClass(Class returnType, Object... parameters);
/**
* Send a request to all {@link Listener}s that satisfy the following requirements.
*
* - They return an {@link Object} that the parameter 'returnType' is assignable from and
* - they belong to a method with parameters that match the given 'parameters' objects
*
*
* The number of {@link Listener}s that are executed may be larger than the number of return values that is returned, because some {@link Listener}s may return nothing (void).
*
* @param returnType the return type that has to be assignable from the return type of the {@link Listener}s
* @param parameters the parameters to give to the {@link Listener}s
* @return all return values from the listeners that executed or an empty {@link Collection} if no matching {@link Listener}s were found. This method never returns null.
*/
Collection sendForMultipleOfClass(Class returnType, Object... parameters);
/**
* Send a request to all {@link Listener}s that satisfy the following requirements. Ignore all return values and return nothing.
*
* - They belong to a method with parameters that match the given 'parameters' objects
*
*
* @param parameters the parameters to give to the {@link Listener}s
*/
void sendForNothing(Object... parameters);
/**
* Send a request to all {@link Listener}s that satisfy the following requirements.
*
* - They have a hint that is equals to the 'hint' parameter and
* - they belong to a method with parameters that match the given 'parameters' objects
*
*
* @param hint the hint that has to be equal to the hint of the {@link Listener}s
* @param parameters the parameters to give to the {@link Listener}s
* @return a single return value from one of the listeners that executed (which one is unspecified) or 'null' if no matching {@link Listener}s were found
* or none of them returned anything
*/
T sendForSingleWithHint(String hint, Object... parameters);
/**
* Send a request to all {@link Listener}s that satisfy the following requirements.
*
* - They have a hint that is equals to the 'hint' parameter and
* - they belong to a method with parameters that match the given 'parameters' objects
*
*
* The number of {@link Listener}s that are executed may be larger than the number of return values that is returned, because some {@link Listener}s may return nothing (void).
*
* @param hint the hint that has to be equal to the hint of the {@link Listener}s
* @param parameters the parameters to give to the {@link Listener}s
* @return all return values from the listeners that executed or an empty {@link Collection} if no matching {@link Listener}s were found or none of them returned anything.
* This method never returns null.
*/
Collection sendForMultipleWithHint(String hint, Object... parameters);
/**
* Send a request to all {@link Listener}s that satisfy the following requirements. Ignore all return values and return nothing.
*
* - They have a hint that is equals to the 'hint' parameter and
* - they belong to a method with parameters that match the given 'parameters' objects
*
*
* @param hint the hint that has to be equal to the hint of the {@link Listener}s
* @param parameters the parameters to give to the {@link Listener}s
*/
void sendForNothingWithHint(String hint, Object... parameters);
/**
* Send a request to all {@link Listener}s that satisfy the following requirements.
*
* - They return an {@link Object} that the parameter 'returnType' is assignable from and
* - they have a hint that is equals to the 'hint' parameter and
* - they belong to a method with parameters that match the given 'parameters' objects
*
*
* @param returnType the return type that has to be assignable from the return type of the {@link Listener}s
* @param hint the hint that has to be equal to the hint of the {@link Listener}s
* @param parameters the parameters to give to the {@link Listener}s
* @return a single return value from one of the listeners that executed (which one is unspecified) or 'null' if no matching {@link Listener}s were found
*/
T sendForSingleOfClassWithHint(Class returnType, String hint, Object... parameters);
/**
* Send a request to all {@link Listener}s that satisfy the following requirements.
*
* - They return an {@link Object} that the parameter 'returnType' is assignable from and
* - they have a hint that is equals to the 'hint' parameter and
* - they belong to a method with parameters that match the given 'parameters' objects
*
*
* The number of {@link Listener}s that are executed may be larger than the number of return values that is returned, because some {@link Listener}s may return nothing (void).
*
* @param returnType the return type that has to be assignable from the return type of the {@link Listener}s
* @param hint the hint that has to be equal to the hint of the {@link Listener}s
* @param parameters the parameters to give to the {@link Listener}s
* @return all return values from the listeners that executed or an empty {@link Collection} if no matching {@link Listener}s were found. This method never returns null.
*/
Collection sendForMultipleOfClassWithHint(Class returnType, String hint, Object... parameters);
/**
* Equivalent to {@link #sendForSingleWithHint(String, Object...)}, since that is probably the most widely used method, because generally, when you know the hint, you know
* the returntype and you don't have to pass it specifically.
*
* This method is just a convenience shorthand method with a shorter name.
*
* @param hint
* @param parameters
* @return same as sendForSingleWithHint
*/
T send(String hint, Object... parameters);
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy