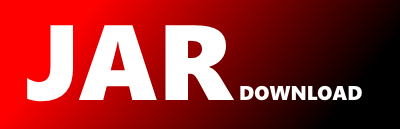
org.rundeck.storage.conf.ConverterTree Maven / Gradle / Ivy
The newest version!
/*
* Copyright 2016 SimplifyOps, Inc. (http://simplifyops.com)
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.rundeck.storage.conf;
import org.rundeck.storage.impl.DelegateResource;
import org.rundeck.storage.impl.DelegateTree;
import org.rundeck.storage.api.*;
/**
* Tree that can convert resource content with a {@link ContentConverter}
*/
public class ConverterTree extends DelegateTree {
ContentConverter converter;
PathSelector pathSelector;
ResourceSelector resourceSelector;
/**
* Convert content from the delegate with the given converter if it matches the selector
*
* @param delegate delegate
* @param converter converter
* @param pathSelector path selection
*/
public ConverterTree(Tree delegate, ContentConverter converter, PathSelector pathSelector) {
this(delegate, converter, pathSelector, null);
}
public ConverterTree(Tree delegate, ContentConverter converter, ResourceSelector resourceSelector) {
this(delegate, converter, null, resourceSelector);
}
public ConverterTree(Tree delegate, ContentConverter converter, PathSelector pathSelector,
ResourceSelector resourceSelector) {
super(delegate);
this.converter = converter;
this.pathSelector = pathSelector;
this.resourceSelector = resourceSelector;
}
private T filterReadData(Path path, T contents) {
return null != converter ? converter.convertReadData(path, contents) : contents;
}
private T filterCreateData(Path path, T contents) {
return null != converter ? converter.convertCreateData(path, contents) : contents;
}
private T filterUpdateData(Path path, T content) {
return null != converter ? converter.convertUpdateData(path, content) : content;
}
@Override
public Resource getResource(Path path) {
final Resource resource = super.getResource(path);
if (!resource.isDirectory() && MatcherUtil.matches(path, resource.getContents(), pathSelector, resourceSelector)) {
return filterGetResource(path, resource);
}
return resource;
}
@Override
public Resource getPath(Path path) {
final Resource resource = super.getPath(path);
if (!resource.isDirectory() && MatcherUtil.matches(path, resource.getContents(), pathSelector, resourceSelector)) {
return filterGetResource(path, resource);
}
return resource;
}
private Resource filterGetResource(final Path path, final Resource resource) {
return new DelegateResource(resource) {
@Override
public T getContents() {
return filterReadData(path, resource.getContents());
}
};
}
@Override
public Resource createResource(Path path, T content) {
if (MatcherUtil.matches(path, content, pathSelector, resourceSelector)) {
Resource created=super.createResource(path, filterCreateData(path, content));
return filterGetResource(path, created);
}
return super.createResource(path, content);
}
@Override
public Resource updateResource(Path path, T content) {
if (MatcherUtil.matches(path, content, pathSelector, resourceSelector)) {
Resource updated = super.updateResource(path, filterUpdateData(path, content));
return filterGetResource(path, updated);
}
return super.updateResource(path, content);
}
}
© 2015 - 2024 Weber Informatics LLC | Privacy Policy