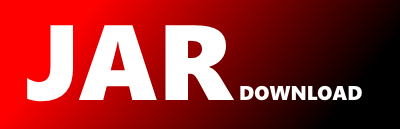
org.sackfix.fix41.AllocationMessage.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of sackfix-messages-fix41_2.11 Show documentation
Show all versions of sackfix-messages-fix41_2.11 Show documentation
All Fix 4.1 strongly typed messages, purely generated code, do not edit.
The newest version!
package org.sackfix.fix41
import org.sackfix.field._
import org.sackfix.common.validated.fields.{SfFixMessageBody, SfFixMessageDecoder, SfFixFieldsToAscii, SfFixRenderable}
import org.sackfix.common.message.SfRepeatingGroupCountException
import scala.annotation.tailrec
import scala.collection.immutable.HashSet
import scala.collection.mutable.ArrayBuffer
/**
* Generated by SackFix code generator on 20170404
* Source specification was read from:
* /quickfixj1.6.0/FIX41.xml
*/
case class AllocationMessage(allocIDField:AllocIDField,
allocTransTypeField:AllocTransTypeField,
refAllocIDField:Option[RefAllocIDField]=None,
allocLinkIDField:Option[AllocLinkIDField]=None,
allocLinkTypeField:Option[AllocLinkTypeField]=None,
noOrdersField:NoOrdersField,
ordersGroups: List[OrdersGroup],
noExecsField:Option[NoExecsField]=None,
execsGroups: Option[List[ExecsGroup]]=None,
sideField:SideField,
symbolField:SymbolField,
symbolSfxField:Option[SymbolSfxField]=None,
securityIDField:Option[SecurityIDField]=None,
iDSourceField:Option[IDSourceField]=None,
securityTypeField:Option[SecurityTypeField]=None,
maturityMonthYearField:Option[MaturityMonthYearField]=None,
maturityDayField:Option[MaturityDayField]=None,
putOrCallField:Option[PutOrCallField]=None,
strikePriceField:Option[StrikePriceField]=None,
optAttributeField:Option[OptAttributeField]=None,
securityExchangeField:Option[SecurityExchangeField]=None,
issuerField:Option[IssuerField]=None,
securityDescField:Option[SecurityDescField]=None,
sharesIntField:SharesIntField,
lastMktField:Option[LastMktField]=None,
avgPxField:AvgPxField,
currencyField:Option[CurrencyField]=None,
avgPrxPrecisionField:Option[AvgPrxPrecisionField]=None,
tradeDateField:TradeDateField,
transactTimeField:Option[TransactTimeField]=None,
settlmntTypField:Option[SettlmntTypField]=None,
futSettDateField:Option[FutSettDateField]=None,
netMoneyField:Option[NetMoneyField]=None,
openCloseField:Option[OpenCloseField]=None,
textField:Option[TextField]=None,
numDaysInterestField:Option[NumDaysInterestField]=None,
accruedInterestRateField:Option[AccruedInterestRateField]=None,
noAllocsField:NoAllocsField,
allocsGroups: List[AllocsGroup]) extends SfFixMessageBody("J") with SfFixRenderable with SfFixFieldsToAscii {
if (noOrdersField.value != ordersGroups.size)
throw SfRepeatingGroupCountException(NoOrdersField.TagId,noOrdersField.value, ordersGroups.size)
if (noExecsField.map(_.value).getOrElse(0) != execsGroups.map(_.size).getOrElse(0))
throw SfRepeatingGroupCountException(NoExecsField.TagId,noExecsField.map(_.value).getOrElse(0), execsGroups.map(_.size).getOrElse(0))
if (noAllocsField.value != allocsGroups.size)
throw SfRepeatingGroupCountException(NoAllocsField.TagId,noAllocsField.value, allocsGroups.size)
override lazy val fixStr : String = appendFixStr().toString
override def appendFixStr(b:StringBuilder = new StringBuilder): StringBuilder = format(formatForFix, b)
override def toString():String = appendStringBuilder().toString()
def appendStringBuilder(b:StringBuilder = new StringBuilder) : StringBuilder = format(formatForToString, b)
def format( fmt: ((StringBuilder,SfFixRenderable)=>Unit), b:StringBuilder = new StringBuilder()): StringBuilder = {
fmt(b,allocIDField)
fmt(b,allocTransTypeField)
refAllocIDField.foreach(fmt(b,_))
allocLinkIDField.foreach(fmt(b,_))
allocLinkTypeField.foreach(fmt(b,_))
fmt(b,noOrdersField)
noExecsField.foreach(fmt(b,_))
execsGroups.getOrElse(List.empty).foreach(fmt(b,_))
fmt(b,sideField)
fmt(b,symbolField)
symbolSfxField.foreach(fmt(b,_))
securityIDField.foreach(fmt(b,_))
iDSourceField.foreach(fmt(b,_))
securityTypeField.foreach(fmt(b,_))
maturityMonthYearField.foreach(fmt(b,_))
maturityDayField.foreach(fmt(b,_))
putOrCallField.foreach(fmt(b,_))
strikePriceField.foreach(fmt(b,_))
optAttributeField.foreach(fmt(b,_))
securityExchangeField.foreach(fmt(b,_))
issuerField.foreach(fmt(b,_))
securityDescField.foreach(fmt(b,_))
fmt(b,sharesIntField)
lastMktField.foreach(fmt(b,_))
fmt(b,avgPxField)
currencyField.foreach(fmt(b,_))
avgPrxPrecisionField.foreach(fmt(b,_))
fmt(b,tradeDateField)
transactTimeField.foreach(fmt(b,_))
settlmntTypField.foreach(fmt(b,_))
futSettDateField.foreach(fmt(b,_))
netMoneyField.foreach(fmt(b,_))
openCloseField.foreach(fmt(b,_))
textField.foreach(fmt(b,_))
numDaysInterestField.foreach(fmt(b,_))
accruedInterestRateField.foreach(fmt(b,_))
fmt(b,noAllocsField)
b
}
}
object AllocationMessage extends SfFixMessageDecoder {
val MsgType="J"
val MsgName="Allocation"
override val MandatoryFields = HashSet[Int](
AllocIDField.TagId, AllocTransTypeField.TagId, NoOrdersField.TagId, SideField.TagId, SymbolField.TagId,
SharesIntField.TagId, AvgPxField.TagId, TradeDateField.TagId, NoAllocsField.TagId)
override def isMandatoryField(tagId:Int) : Boolean = {
MandatoryFields.contains(tagId) ||
OrdersGroup.isMandatoryField(tagId) || ExecsGroup.isMandatoryField(tagId) || AllocsGroup.isMandatoryField(tagId)
}
override val OptionalFields = HashSet[Int](
RefAllocIDField.TagId, AllocLinkIDField.TagId, AllocLinkTypeField.TagId, NoExecsField.TagId, SymbolSfxField.TagId,
SecurityIDField.TagId, IDSourceField.TagId, SecurityTypeField.TagId, MaturityMonthYearField.TagId, MaturityDayField.TagId,
PutOrCallField.TagId, StrikePriceField.TagId, OptAttributeField.TagId, SecurityExchangeField.TagId, IssuerField.TagId,
SecurityDescField.TagId, LastMktField.TagId, CurrencyField.TagId, AvgPrxPrecisionField.TagId, TransactTimeField.TagId,
SettlmntTypField.TagId, FutSettDateField.TagId, NetMoneyField.TagId, OpenCloseField.TagId, TextField.TagId,
NumDaysInterestField.TagId, AccruedInterestRateField.TagId)
override def isOptionalField(tagId:Int) : Boolean = {
OptionalFields.contains(tagId) ||
OrdersGroup.isOptionalField(tagId) || ExecsGroup.isOptionalField(tagId) || AllocsGroup.isOptionalField(tagId)
}
override def isFieldOf(tagId:Int) : Boolean = isMandatoryField(tagId) || isOptionalField(tagId) ||
OrdersGroup.isFieldOf(tagId) || ExecsGroup.isFieldOf(tagId) || AllocsGroup.isFieldOf(tagId)
override lazy val RepeatingGroupsTags = HashSet[Int](
NoOrdersField.TagId, NoExecsField.TagId, NoAllocsField.TagId)
override def isFirstField(tagId:Int) : Boolean = tagId==AllocIDField.TagId
override def decode(flds: Seq[Tuple2[Int, Any]], startPos:Int = 0):Option[SfFixMessageBody] = {
val (pos, myFields, nextTagPosLookup) = extractMyFieldsAndPopulatePositions(false, flds, startPos)
validateMandatoryFieldsPresent(myFields)
if (MandatoryFields.isEmpty || myFields.nonEmpty) {
Some(AllocationMessage(AllocIDField.decode(myFields.get(AllocIDField.TagId)).get,
AllocTransTypeField.decode(myFields.get(AllocTransTypeField.TagId)).get,
myFields.get(RefAllocIDField.TagId).flatMap(f=>RefAllocIDField.decode(f)),
myFields.get(AllocLinkIDField.TagId).flatMap(f=>AllocLinkIDField.decode(f)),
myFields.get(AllocLinkTypeField.TagId).flatMap(f=>AllocLinkTypeField.decode(f)),
NoOrdersField.decode(myFields.get(NoOrdersField.TagId)).get,
if (nextTagPosLookup.contains(NoOrdersField.TagId)) OrdersGroup.decode(flds, nextTagPosLookup(NoOrdersField.TagId)).get else List.empty,
myFields.get(NoExecsField.TagId).flatMap(f=>NoExecsField.decode(f)),
if (nextTagPosLookup.contains(NoExecsField.TagId)) ExecsGroup.decode(flds, nextTagPosLookup(NoExecsField.TagId)) else None,
SideField.decode(myFields.get(SideField.TagId)).get,
SymbolField.decode(myFields.get(SymbolField.TagId)).get,
myFields.get(SymbolSfxField.TagId).flatMap(f=>SymbolSfxField.decode(f)),
myFields.get(SecurityIDField.TagId).flatMap(f=>SecurityIDField.decode(f)),
myFields.get(IDSourceField.TagId).flatMap(f=>IDSourceField.decode(f)),
myFields.get(SecurityTypeField.TagId).flatMap(f=>SecurityTypeField.decode(f)),
myFields.get(MaturityMonthYearField.TagId).flatMap(f=>MaturityMonthYearField.decode(f)),
myFields.get(MaturityDayField.TagId).flatMap(f=>MaturityDayField.decode(f)),
myFields.get(PutOrCallField.TagId).flatMap(f=>PutOrCallField.decode(f)),
myFields.get(StrikePriceField.TagId).flatMap(f=>StrikePriceField.decode(f)),
myFields.get(OptAttributeField.TagId).flatMap(f=>OptAttributeField.decode(f)),
myFields.get(SecurityExchangeField.TagId).flatMap(f=>SecurityExchangeField.decode(f)),
myFields.get(IssuerField.TagId).flatMap(f=>IssuerField.decode(f)),
myFields.get(SecurityDescField.TagId).flatMap(f=>SecurityDescField.decode(f)),
SharesIntField.decode(myFields.get(SharesIntField.TagId)).get,
myFields.get(LastMktField.TagId).flatMap(f=>LastMktField.decode(f)),
AvgPxField.decode(myFields.get(AvgPxField.TagId)).get,
myFields.get(CurrencyField.TagId).flatMap(f=>CurrencyField.decode(f)),
myFields.get(AvgPrxPrecisionField.TagId).flatMap(f=>AvgPrxPrecisionField.decode(f)),
TradeDateField.decode(myFields.get(TradeDateField.TagId)).get,
myFields.get(TransactTimeField.TagId).flatMap(f=>TransactTimeField.decode(f)),
myFields.get(SettlmntTypField.TagId).flatMap(f=>SettlmntTypField.decode(f)),
myFields.get(FutSettDateField.TagId).flatMap(f=>FutSettDateField.decode(f)),
myFields.get(NetMoneyField.TagId).flatMap(f=>NetMoneyField.decode(f)),
myFields.get(OpenCloseField.TagId).flatMap(f=>OpenCloseField.decode(f)),
myFields.get(TextField.TagId).flatMap(f=>TextField.decode(f)),
myFields.get(NumDaysInterestField.TagId).flatMap(f=>NumDaysInterestField.decode(f)),
myFields.get(AccruedInterestRateField.TagId).flatMap(f=>AccruedInterestRateField.decode(f)),
NoAllocsField.decode(myFields.get(NoAllocsField.TagId)).get,
if (nextTagPosLookup.contains(NoAllocsField.TagId)) AllocsGroup.decode(flds, nextTagPosLookup(NoAllocsField.TagId)).get else List.empty))
} else None
}
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy