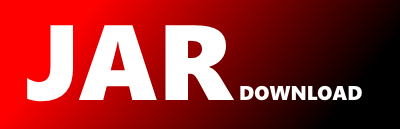
org.sahagin.share.yaml.YamlUtils Maven / Gradle / Ivy
package org.sahagin.share.yaml;
import java.io.File;
import java.io.FileInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.util.ArrayList;
import java.util.List;
import java.util.Map;
import org.apache.commons.io.Charsets;
import org.apache.commons.io.output.FileWriterWithEncoding;
import org.apache.commons.lang.StringUtils;
import org.openqa.selenium.io.IOUtils;
import org.sahagin.runlib.external.CaptureStyle;
import org.sahagin.runlib.external.Locale;
import org.yaml.snakeyaml.Yaml;
public class YamlUtils {
private static final String MSG_KEY_NOT_FOUND = "key \"%s\" is not found";
private static final String MSG_MUST_BE_BOOLEAN
= "value for \"%s\" must be \"true\" or \"false\", but is \"%s\"";
private static final String MSG_VALUE_NOT_INT = "can't convert value to int; key: %s; vaule: %s";
private static final String MSG_VALUE_NOT_CAPTURE_STYLE
= "can't convert value to CaptureStyle; key: %s; vaule: %s";
private static final String MSG_VALUE_NOT_LOCALE
= "can't convert value to Locale; key: %s; vaule: %s";
private static final String MSG_NOT_EQUALS_TO_EXPECTED = "\"%s\" is not equals to \"%s\"";
private static final String MSG_LIST_MUST_NOT_BE_NULL = "list must not be null";
// if allowsEmpty and key entry is not found, just returns null.
// (null may mean null value for the specified key)
public static Object getObjectValue(Map yamlObject, String key, boolean allowsEmpty)
throws YamlConvertException {
if (yamlObject == null) {
throw new NullPointerException();
}
Object obj = yamlObject.get(key);
if (obj == null && !yamlObject.containsKey(key) && !allowsEmpty) {
throw new YamlConvertException(String.format(MSG_KEY_NOT_FOUND, key));
}
return obj;
}
public static Object getObjectValue(Map yamlObject, String key)
throws YamlConvertException {
return getObjectValue(yamlObject, key, false);
}
// if allowsEmpty, returns null for the case no key entry or null value
public static Boolean getBooleanValue(Map yamlObject, String key, boolean allowsEmpty)
throws YamlConvertException {
Object obj = getObjectValue(yamlObject, key, allowsEmpty);
if (obj == null) {
if (allowsEmpty) {
return null;
} else {
throw new YamlConvertException(String.format(MSG_MUST_BE_BOOLEAN, key, obj));
}
} else if (obj.toString().equalsIgnoreCase("true")) {
return Boolean.TRUE;
} else if (obj.toString().equalsIgnoreCase("false")) {
return Boolean.FALSE;
} else {
throw new YamlConvertException(String.format(MSG_MUST_BE_BOOLEAN, key, obj));
}
}
public static Boolean getBooleanValue(Map yamlObject, String key)
throws YamlConvertException {
return getBooleanValue(yamlObject, key, false);
}
public static String getStrValue(Map yamlObject, String key, boolean allowsEmpty)
throws YamlConvertException {
Object obj = getObjectValue(yamlObject, key, allowsEmpty);
if (obj == null) {
return null;
} else {
return obj.toString();
}
}
public static String getStrValue(Map yamlObject, String key)
throws YamlConvertException {
return getStrValue(yamlObject, key, false);
}
public static void strValueEqualsCheck(Map yamlObject,
String key, String expected, String defaultValue)
throws YamlConvertException {
String value = getStrValue(yamlObject, key, true);
if (value == null) {
value = defaultValue;
}
if (!StringUtils.equals(value, expected)) {
throw new YamlConvertException(String.format(
MSG_NOT_EQUALS_TO_EXPECTED, value, expected));
}
}
public static void strValueEqualsCheck(Map yamlObject, String key, String expected)
throws YamlConvertException {
String value = getStrValue(yamlObject, key);
if (!StringUtils.equals(value, expected)) {
throw new YamlConvertException(String.format(
MSG_NOT_EQUALS_TO_EXPECTED, value, expected));
}
}
// if allowsEmpty, returns null for the case no key entry or null value
public static Integer getIntValue(Map yamlObject, String key, boolean allowsEmpty)
throws YamlConvertException {
Object obj = getObjectValue(yamlObject, key, allowsEmpty);
if (obj == null && allowsEmpty) {
return null;
}
String objStr;
if (obj == null) {
objStr = null;
} else {
objStr = obj.toString();
}
try {
return new Integer(objStr);
} catch (NumberFormatException e) {
throw new YamlConvertException(String.format(MSG_VALUE_NOT_INT, key, objStr));
}
}
public static Integer getIntValue(Map yamlObject, String key)
throws YamlConvertException {
return getIntValue(yamlObject, key, false);
}
// if allowsEmpty, returns null for the case no key entry or null value
public static CaptureStyle getCaptureStyleValue(Map yamlObject,
String key, boolean allowsEmpty) throws YamlConvertException {
Object obj = getObjectValue(yamlObject, key, allowsEmpty);
if (obj == null && allowsEmpty) {
return null;
}
String objStr;
if (obj == null) {
objStr = null;
} else {
objStr = obj.toString();
}
CaptureStyle result = CaptureStyle.getEnum(objStr);
if (result != null) {
return result;
} else {
throw new YamlConvertException(String.format(MSG_VALUE_NOT_CAPTURE_STYLE, key, objStr));
}
}
public static CaptureStyle getCaptureStyleValue(Map yamlObject,
String key) throws YamlConvertException {
return getCaptureStyleValue(yamlObject, key, false);
}
public static Locale getLocaleValue(Map yamlObject, String key, boolean allowsEmpty)
throws YamlConvertException {
Object obj = getObjectValue(yamlObject, key, allowsEmpty);
if (obj == null && allowsEmpty) {
return null;
}
String objStr;
if (obj == null) {
objStr = null;
} else {
objStr = obj.toString();
}
Locale result = Locale.getEnum(objStr);
if (result != null) {
return result;
} else {
throw new YamlConvertException(String.format(MSG_VALUE_NOT_LOCALE, key, objStr));
}
}
public static Locale getLocaleValue(Map yamlObject,
String key) throws YamlConvertException {
return getLocaleValue(yamlObject, key, false);
}
// returns null for empty
public static Map getYamlObjectValue(Map yamlObject,
String key, boolean allowsEmpty) throws YamlConvertException {
Object obj = getObjectValue(yamlObject, key, allowsEmpty);
@SuppressWarnings("unchecked")
Map result = (Map) obj;
return result;
}
// for null or not found, returns null
public static Map getYamlObjectValue(Map yamlObject,
String key) throws YamlConvertException {
return getYamlObjectValue(yamlObject, key, false);
}
// for null or not found key, returns empty list
public static List getStrListValue(Map yamlObject, String key,
boolean allowsEmpty) throws YamlConvertException {
Object obj = getObjectValue(yamlObject, key, allowsEmpty);
@SuppressWarnings("unchecked")
List result = (List) obj;
if (result == null) {
if (!allowsEmpty) {
throw new YamlConvertException(MSG_LIST_MUST_NOT_BE_NULL);
}
result = new ArrayList(0);
}
return result;
}
public static List getStrListValue(Map yamlObject, String key)
throws YamlConvertException {
return getStrListValue(yamlObject, key, false);
}
// for null or not found key, returns empty list
public static List getIntListValue(Map yamlObject, String key,
boolean allowsEmpty) throws YamlConvertException {
Object obj = getObjectValue(yamlObject, key, allowsEmpty);
@SuppressWarnings("unchecked")
List result = (List) obj;
if (result == null) {
if (!allowsEmpty) {
throw new YamlConvertException(MSG_LIST_MUST_NOT_BE_NULL);
}
result = new ArrayList(0);
}
return result;
}
public static List getIntListValue(Map yamlObject, String key)
throws YamlConvertException {
return getIntListValue(yamlObject, key, false);
}
public static Map toYamlObject(YamlConvertible src) {
if (src == null) {
return null;
} else {
return src.toYamlObject();
}
}
// for null or not found key, returns empty list
public static List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy