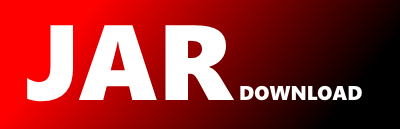
org.sakaiproject.util.BaseDbDoubleStorage Maven / Gradle / Ivy
/**********************************************************************************
* $URL: https://source.sakaiproject.org/svn/kernel/trunk/kernel-util/src/main/java/org/sakaiproject/util/BaseDbDoubleStorage.java $
* $Id: BaseDbDoubleStorage.java 66393 2009-09-10 08:19:24Z [email protected] $
***********************************************************************************
*
* Copyright (c) 2005, 2006, 2007, 2008 Sakai Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ECL-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
**********************************************************************************/
package org.sakaiproject.util;
import java.sql.Connection;
import java.sql.ResultSet;
import java.sql.SQLException;
import java.util.Hashtable;
import java.util.Iterator;
import java.util.List;
import java.util.Map;
import java.util.Stack;
import java.util.Vector;
import lombok.extern.slf4j.Slf4j;
import org.apache.commons.lang3.ArrayUtils;
import org.w3c.dom.Document;
import org.w3c.dom.Element;
import org.sakaiproject.db.api.SqlReader;
import org.sakaiproject.db.api.SqlReaderFinishedException;
import org.sakaiproject.db.api.SqlService;
import org.sakaiproject.entity.api.Edit;
import org.sakaiproject.entity.api.Entity;
import org.sakaiproject.entity.api.ResourceProperties;
import org.sakaiproject.event.cover.UsageSessionService;
import org.sakaiproject.javax.Filter;
import org.sakaiproject.javax.Order;
import org.sakaiproject.javax.PagingPosition;
import org.sakaiproject.javax.Search;
import org.sakaiproject.javax.SearchFilter;
import org.sakaiproject.time.api.Time;
import org.sakaiproject.time.cover.TimeService;
/**
*
* BaseDbDoubleStorage is a class that stores collections of Resources (of some type) in a database,
* provides locked access, and generally implements a services "storage" class. The
* service's storage class can extend this to provide covers to turn Resource and
* Edit into something more type specific to the service.
*
*
* Note: the methods here are all "id" based, with the following assumptions:
*
* - just the Resource Id field is enough to distinguish one Resource from another (or, for resource, the container's id and the resource id).
* - a resource's reference is based on no more than the resource id, for containers
* - and no more than resource and container id for resources
* - a resource's id and container id cannot change
*
*
*
* In order to handle Unicode characters properly, the SQL statements executed by this class should not embed Unicode characters into the SQL
* statement text;
* rather, Unicode values should be inserted as fields in a PreparedStatement. Databases handle Unicode better in fields.
*
*/
@Slf4j
public class BaseDbDoubleStorage
{
/** Table name for container records. */
protected String m_containerTableName = null;
/** The field in the table that holds the container id. */
protected String m_containerTableIdField = null;
/** Table name for resource records. */
protected String m_resourceTableName = null;
/** The field in the resource table that holds the resource id. */
protected String m_resourceTableIdField = null;
/** The field in the resource table that holds the container id. */
protected String m_resourceTableContainerIdField = null;
/** The additional field names in the resource table that go between the two ids and the xml. */
protected String[] m_resourceTableOtherFields = null;
/** The string searchable field names in the resource table. This must be either null
* (i.e. no fields) or this is assumed to be the only fields which participate in search.
*/
protected String[] m_resourceTableSearchFields = null;
/** The field name in the resource table for ordering. */
protected String m_resourceTableOrderField = null;
/** The xml tag name for the element holding each actual resource entry. */
protected String m_resourceEntryTagName = null;
/** The xml tag name for the element holding each actual container entry. */
protected String m_containerEntryTagName = null;
/** The field in the record that has the user id of the resource owner. */
protected String m_resourceTableOwnerField = null;
/** The field in the record that has the draft indicator ('0' for no, '1' for yes). */
protected String m_resourceTableDraftField = null;
/** The field in the record that has the pubview indicator ('0' for no, '1' for yes). */
protected String m_resourceTablePubViewField = null;
/** If true, we do our locks in the remote database. */
protected boolean m_locksAreInDb = true;
/** If true, we do our locks in the remove database using a separate locking table. */
protected boolean m_locksAreInTable = true;
/** The StorageUser to callback for new Resource and Edit objects. */
protected DoubleStorageUser m_user = null;
/**
* Locks, keyed by reference, holding Connections (or, if locks are done locally, holding an Edit). Note: keying by reference allows botu
* container and resource locks to be stored, the reference distinguishes them.
*/
protected Hashtable m_locks = null;
/** For container, the extra field is (no longer used) NEXT_ID */
protected static final String[] M_containerExtraFields = {"NEXT_ID"};
/** Injected (by constructor) SqlService. */
protected SqlService m_sql = null;
/** contains a map of the database dependent handlers. */
protected static Map databaseBeans;
/** The db handler we are using. */
protected DoubleStorageSql doubleStorageSql;
public void setDatabaseBeans(Map databaseBeans)
{
this.databaseBeans = databaseBeans;
}
/**
* sets which bean containing database dependent code should be used depending on the database vendor.
*/
public void setDoubleStorageSql(String vendor)
{
this.doubleStorageSql = (databaseBeans.containsKey(vendor) ? databaseBeans.get(vendor) : databaseBeans.get("default"));
}
// since spring is not used and this class is instatiated directly, we need to "inject" these values ourselves
static
{
databaseBeans = new Hashtable();
databaseBeans.put("default", new DoubleStorageSqlDefault());
databaseBeans.put("hsqldb", new DoubleStorageSqlHSql());
databaseBeans.put("mysql", new DoubleStorageSqlMySql());
databaseBeans.put("oracle", new DoubleStorageSqlOracle());
}
/**
* Construct.
*
* @param containerTableName
* Table name for containers.
* @param containerTableIdField
* The field in the container table that holds the id.
* @param resourceTableName
* Table name for resources.
* @param resourceTableIdField
* The field in the resource table that holds the id.
* @param resourceTableContainerIdField
* The field in the resource table that holds the container id.
* @param resourceTableOrderField
* The field in the resource table that is used for ordering results.
* @param resourceTableOtherFields
* The other fields in the resource table (between the two id fields and the xml field).
* @param locksInDb
* If true, we do our locks in the remote database, otherwise we do them here.
* @param containerEntryName
* The xml tag name for the element holding each actual container entry.
* @param resourceEntryName
* The xml tag name for the element holding each actual resource entry.
* @param user
* The StorageUser class to call back for creation of Resource and Edit objects.
* @param sqlService
* The SqlService.
*/
public BaseDbDoubleStorage(String containerTableName, String containerTableIdField,
String resourceTableName, String resourceTableIdField,
String resourceTableContainerIdField, String resourceTableOrderField,
String resourceTableOwnerField, String resourceTableDraftField,
String resourceTablePubViewField, String[] resourceTableOtherFields, String[] resourceTableSearchFields,
boolean locksInDb, String containerEntryName,
String resourceEntryName, DoubleStorageUser user, SqlService sqlService)
{
m_containerTableName = containerTableName;
m_containerTableIdField = containerTableIdField;
m_resourceTableName = resourceTableName;
m_resourceTableIdField = resourceTableIdField;
m_resourceTableContainerIdField = resourceTableContainerIdField;
m_resourceTableOrderField = resourceTableOrderField;
m_resourceTableOtherFields = resourceTableOtherFields;
m_resourceTableSearchFields = resourceTableSearchFields;
m_locksAreInDb = locksInDb;
m_containerEntryTagName = containerEntryName;
m_resourceEntryTagName = resourceEntryName;
m_resourceTableOwnerField = resourceTableOwnerField;
m_resourceTableDraftField = resourceTableDraftField;
m_resourceTablePubViewField = resourceTablePubViewField;
m_user = user;
m_sql = sqlService;
setDoubleStorageSql(m_sql.getVendor());
}
/** Backwards compatibility constructor for using DbDouble without search fields */
public BaseDbDoubleStorage(String containerTableName, String containerTableIdField,
String resourceTableName, String resourceTableIdField,
String resourceTableContainerIdField, String resourceTableOrderField,
String resourceTableOwnerField, String resourceTableDraftField,
String resourceTablePubViewField, String[] resourceTableOtherFields, // String[] resourceTableSearchFields,
boolean locksInDb, String containerEntryName,
String resourceEntryName, DoubleStorageUser user, SqlService sqlService)
{
m_containerTableName = containerTableName;
m_containerTableIdField = containerTableIdField;
m_resourceTableName = resourceTableName;
m_resourceTableIdField = resourceTableIdField;
m_resourceTableContainerIdField = resourceTableContainerIdField;
m_resourceTableOrderField = resourceTableOrderField;
m_resourceTableOtherFields = resourceTableOtherFields;
m_resourceTableSearchFields = null; // resourceTableSearchFields;
m_locksAreInDb = locksInDb;
m_containerEntryTagName = containerEntryName;
m_resourceEntryTagName = resourceEntryName;
m_resourceTableOwnerField = resourceTableOwnerField;
m_resourceTableDraftField = resourceTableDraftField;
m_resourceTablePubViewField = resourceTablePubViewField;
m_user = user;
m_sql = sqlService;
setDoubleStorageSql(m_sql.getVendor());
}
/**
* Open and be ready to read / write.
*/
public void open()
{
// setup for locks
m_locks = new Hashtable();
}
/**
* Close.
*/
public void close()
{
if (!m_locks.isEmpty())
{
log.warn("close(): locks remain!");
// %%%
}
m_locks.clear();
m_locks = null;
}
/**
* Read one Container Resource from xml
*
* @param xml
* An string containing the xml which describes the Container resource.
* @return The Container Resource object created from the xml.
*/
protected Entity readContainer(String xml)
{
try
{
if ( m_user instanceof SAXEntityReader ) {
SAXEntityReader sm_user = (SAXEntityReader) m_user;
DefaultEntityHandler deh = sm_user.getDefaultHandler(sm_user.getServices());
StorageUtils.processString(xml, deh);
return deh.getEntity();
} else {
// read the xml
Document doc = StorageUtils.readDocumentFromString(xml);
// verify the root element
Element root = doc.getDocumentElement();
if (!root.getTagName().equals(m_containerEntryTagName))
{
log.warn("readContainer(): not = " + m_containerEntryTagName + " : " + root.getTagName());
return null;
}
// re-create a resource
Entity entry = m_user.newContainer(root);
return entry;
}
}
catch (Exception e)
{
log.warn("readContainer(): "+e.getMessage());
log.info("readContainer(): ", e);
return null;
}
}
/**
* Check if a Container by this id exists.
*
* @param ref
* The container reference.
* @return true if a Container by this id exists, false if not.
*/
public boolean checkContainer(String ref)
{
// just see if the record exists
String sql = doubleStorageSql.getSelect1Sql(m_containerTableName, m_containerTableIdField);
Object[] fields = new Object[1];
fields[0] = ref;
List ids = m_sql.dbRead(sql, fields, null);
return (!ids.isEmpty());
}
/**
* Get the Container with this id, or null if not found.
*
* @param ref
* The container reference.
* @return The Container with this id, or null if not found.
*/
public Entity getContainer(String ref)
{
Entity entry = null;
// get the user from the db
String sql = doubleStorageSql.getSelectXml2Sql(m_containerTableName, m_containerTableIdField);
Object[] fields = new Object[1];
fields[0] = ref;
List xml = m_sql.dbRead(sql, fields, null);
if (!xml.isEmpty())
{
// create the Resource from the db xml
entry = readContainer((String) xml.get(0));
}
return entry;
}
/**
* Get all Containers.
*
* @return The list (Resource) of all Containers.
*/
public List getAllContainers()
{
List all = new Vector();
// read all users from the db
String sql = doubleStorageSql.getSelectXml1Sql(m_containerTableName);
// %%% order by...
List xml = m_sql.dbRead(sql);
// process all result xml into user objects
if (!xml.isEmpty())
{
for (int i = 0; i < xml.size(); i++)
{
Entity entry = readContainer((String) xml.get(i));
if (entry != null) all.add(entry);
}
}
return all;
}
/**
* Add a new Container with this id.
*
* @param ref
* The container reference.
* @return The locked Container object with this id, or null if the id is in use.
*/
public Edit putContainer(String ref)
{
// create one with just the id
Entity entry = m_user.newContainer(ref);
// form the XML and SQL for the insert
Document doc = StorageUtils.createDocument();
entry.toXml(doc, new Stack());
String xml = StorageUtils.writeDocumentToString(doc);
String statement = doubleStorageSql.getInsertSql(m_containerTableName, insertFields(m_containerTableIdField, null, M_containerExtraFields,
"XML"));
Object[] fields = new Object[2];
fields[0] = entry.getReference();
fields[1] = xml;
// process the insert
boolean ok = m_sql.dbWrite(statement, fields);
// if this failed, assume a key conflict (i.e. id in use)
if (!ok) return null;
// now get a lock on the record for edit
Edit edit = editContainer(ref);
if (edit == null)
{
log.warn("putContainer(): didn't get a lock!");
return null;
}
return edit;
}
/**
* Get a lock on the Container with this id, or null if a lock cannot be gotten.
*
* @param ref
* The container reference.
* @return The locked Container with this id, or null if this cannot be locked.
*/
public Edit editContainer(String ref)
{
Edit edit = null;
if (m_locksAreInDb)
{
if ("oracle".equals(m_sql.getVendor()))
{
// read the record and get a lock on it (non blocking)
String statement = doubleStorageSql.getSelectXml3Sql(m_containerTableName, m_containerTableIdField, StorageUtils.escapeSql(ref));
StringBuilder result = new StringBuilder();
Connection lock = m_sql.dbReadLock(statement, result);
// for missing or already locked...
if ((lock == null) || (result.length() == 0)) return null;
// make first a Resource, then an Edit
Entity entry = readContainer(result.toString());
edit = m_user.newContainerEdit(entry);
// store the lock for this object
m_locks.put(entry.getReference(), lock);
}
else
{
throw new UnsupportedOperationException("Record locking only available when configured with Oracle database");
}
}
// if the locks are in a separate table in the db
else if (m_locksAreInTable)
{
// get, and return if not found
Entity entry = getContainer(ref);
if (entry == null) return null;
// write a lock to the lock table - if we can do it, we get the lock
String statement = doubleStorageSql.getInsertSql2();
// we need session id
String sessionId = UsageSessionService.getSessionId();
if (sessionId == null)
{
sessionId = "";
}
// collect the fields
Object fields[] = new Object[4];
fields[0] = m_containerTableName;
fields[1] = doubleStorageSql.getRecordId(ref);
fields[2] = TimeService.newTime();
fields[3] = sessionId;
// add the lock - if fails, someone else has the lock
boolean ok = m_sql.dbWriteFailQuiet(null, statement, fields);
if (!ok)
{
return null;
}
// make the edit from the Resource
edit = m_user.newContainerEdit(entry);
}
// otherwise, get the lock locally
else
{
// get, and return if not found
Entity entry = getContainer(ref);
if (entry == null) return null;
// we only sync this getting - someone may release a lock out of sync
synchronized (m_locks)
{
// if already locked
if (m_locks.containsKey(entry.getReference())) return null;
// make the edit from the Resource
edit = m_user.newContainerEdit(entry);
// store the edit in the locks by reference
m_locks.put(entry.getReference(), edit);
}
}
return edit;
}
/**
* Commit the changes and release the lock.
*
* @param user
* The Edit to commit.
*/
public void commitContainer(Edit edit)
{
// form the SQL statement and the var w/ the XML
Document doc = StorageUtils.createDocument();
edit.toXml(doc, new Stack());
String xml = StorageUtils.writeDocumentToString(doc);
String statement = doubleStorageSql.getUpdateSql(m_containerTableName, m_containerTableIdField);
Object[] fields = new Object[2];
fields[0] = xml;
fields[1] = edit.getReference();
if (m_locksAreInDb)
{
// use this connection that is stored with the lock
Connection lock = (Connection) m_locks.get(edit.getReference());
if (lock == null)
{
log.warn("commitContainer(): edit not in locks");
return;
}
// update, commit, release the lock's connection
m_sql.dbUpdateCommit(statement, fields, null, lock);
// remove the lock
m_locks.remove(edit.getReference());
}
else if (m_locksAreInTable)
{
// process the update
m_sql.dbWrite(statement, fields);
// remove the lock
statement = doubleStorageSql.getDeleteLocksSql();
// collect the fields
Object lockFields[] = new Object[2];
lockFields[0] = m_containerTableName;
lockFields[1] = doubleStorageSql.getRecordId(edit.getReference());
boolean ok = m_sql.dbWrite(statement, lockFields);
if (!ok)
{
log.warn("commitContainer: missing lock for table: " + lockFields[0] + " key: " + lockFields[1]);
}
}
else
{
// just process the update
m_sql.dbWrite(statement, fields);
// remove the lock
m_locks.remove(edit.getReference());
}
}
/**
* Cancel the changes and release the lock.
*
* @param user
* The Edit to cancel.
*/
public void cancelContainer(Edit edit)
{
if (m_locksAreInDb)
{
// use this connection that is stored with the lock
Connection lock = (Connection) m_locks.get(edit.getReference());
if (lock == null)
{
log.warn("cancelContainer(): edit not in locks");
return;
}
// rollback and release the lock's connection
m_sql.dbCancel(lock);
// release the lock
m_locks.remove(edit.getReference());
}
else if (m_locksAreInTable)
{
// remove the lock
String statement = doubleStorageSql.getDeleteLocksSql();
// collect the fields
Object lockFields[] = new Object[2];
lockFields[0] = m_containerTableName;
lockFields[1] = doubleStorageSql.getRecordId(edit.getReference());
boolean ok = m_sql.dbWrite(statement, lockFields);
if (!ok)
{
log.warn("cancelContainer: missing lock for table: " + lockFields[0] + " key: " + lockFields[1]);
}
}
else
{
// release the lock
m_locks.remove(edit.getReference());
}
}
/**
* Remove this (locked) Container.
*
* @param user
* The Edit to remove.
*/
public void removeContainer(Edit edit)
{
// form the SQL delete statement
String statement = doubleStorageSql.getDeleteSql(m_containerTableName, m_containerTableIdField);
Object[] fields = new Object[1];
fields[0] = edit.getReference();
if (m_locksAreInDb)
{
// use this connection that is stored with the lock
Connection lock = (Connection) m_locks.get(edit.getReference());
if (lock == null)
{
log.warn("removeContainer(): edit not in locks");
return;
}
// process the delete statement, commit, and release the lock's connection
m_sql.dbUpdateCommit(statement, fields, null, lock);
// release the lock
m_locks.remove(edit.getReference());
}
else if (m_locksAreInTable)
{
// process the delete statement
m_sql.dbWrite(statement, fields);
// remove the lock
statement = doubleStorageSql.getDeleteLocksSql();
// collect the fields
Object lockFields[] = new Object[2];
lockFields[0] = m_containerTableName;
lockFields[1] = doubleStorageSql.getRecordId(edit.getReference());
boolean ok = m_sql.dbWrite(statement, lockFields);
if (!ok)
{
log.warn("remove: missing lock for table: " + lockFields[0] + " key: " + lockFields[1]);
}
}
else
{
// process the delete statement
m_sql.dbWrite(statement, fields);
// release the lock
m_locks.remove(edit.getReference());
}
}
/**
* Read one Resource from xml
*
* @param container
* The container for this resource.
* @param xml
* An string containing the xml which describes the resource.
* @return The Resource object created from the xml.
*/
protected Entity readResource(Entity container, String xml)
{
try
{
if ( m_user instanceof SAXEntityReader ) {
SAXEntityReader sm_user = (SAXEntityReader) m_user;
DefaultEntityHandler deh = sm_user.getDefaultHandler(sm_user.getServices());
deh.setContainer(container);
StorageUtils.processString(xml, deh);
return deh.getEntity();
} else {
// read the xml
Document doc = StorageUtils.readDocumentFromString(xml);
//The resulting doc could be null
if (doc == null) {
log.warn("null xml document passed to readResource for container" + container.getId());
return null;
}
// verify the root element
Element root = doc.getDocumentElement();
if (!root.getTagName().equals(m_resourceEntryTagName))
{
log.warn("readResource(): not = " + m_resourceEntryTagName + " : " + root.getTagName());
return null;
}
// re-create a resource
Entity entry = m_user.newResource(container, root);
return entry;
}
}
catch (Exception e)
{
log.warn("readResource(): "+e.getMessage());
log.info("readResource(): ", e);
return null;
}
}
/**
* Check if a Resource by this id exists.
*
* @param container
* The container for this resource.
* @param id
* The id.
* @return true if a Resource by this id exists, false if not.
*/
public boolean checkResource(Entity container, String id)
{
// just see if the record exists
String sql = doubleStorageSql.getSelectIdSql(m_resourceTableName, m_resourceTableIdField, m_resourceTableContainerIdField);
Object[] fields = new Object[2];
fields[0] = container.getReference();
fields[1] = id;
List ids = m_sql.dbRead(sql, fields, null);
return (!ids.isEmpty());
}
/**
* Get the Resource with this id, or null if not found.
*
* @param container
* The container for this resource.
* @param id
* The id.
* @return The Resource with this id, or null if not found.
*/
public Entity getResource(Entity container, String id)
{
Entity entry = null;
// get the user from the db
String sql = doubleStorageSql.getSelectXml4Sql(m_resourceTableName, m_resourceTableIdField, m_resourceTableContainerIdField);
Object[] fields = new Object[2];
fields[0] = container.getReference();
fields[1] = id;
List xml = m_sql.dbRead(sql, fields, null);
if (!xml.isEmpty())
{
// create the Resource from the db xml
entry = readResource(container, (String) xml.get(0));
}
return entry;
}
/**
* Count all Resources
* @param container
* The container for this resource.
*/
public int getCount(Entity container)
{
// read With or without a filter
String sql = doubleStorageSql.getCountSql(m_resourceTableName, m_resourceTableContainerIdField);
Object[] fields = new Object[1];
fields[0] = container.getReference();
List countList = m_sql.dbRead(sql, fields, null);
if ( countList.isEmpty() ) return 0;
Object obj = countList.get(0);
String str = (String) obj;
return Integer.parseInt(str);
}
/**
* Count all Resources - This takes two approaches depending
* on whether this table has search fields. If searchfields
* are available we can do a SELECT COUNT WHERE. Otherwise
* we retrieve the records and run the filter on each record.
* @param container
* The container for this resource.
* @param filter
* A filter object to accept / reject the searches
* @param search
* Search string
*/
public int getCount(Entity container, Filter filter)
{
if ( filter == null ) return getCount(container);
// If we have search fields - do a quick select count with a where clause
if ( m_resourceTableSearchFields != null && filter instanceof SearchFilter )
{
int searchFieldCount = 0;
String searchString = ((SearchFilter) filter).getSearchString();
if ( searchString != null && searchString.length() > 0 )
{
String searchWhere = doubleStorageSql.getSearchWhereClause(m_resourceTableSearchFields);
if ( searchWhere != null && searchWhere.length() > 0 )
{
searchFieldCount = m_resourceTableSearchFields.length;
String sql = doubleStorageSql.getCountSqlWhere(m_resourceTableName,
m_resourceTableContainerIdField, searchWhere);
Object[] fields = new Object[1+searchFieldCount];
fields[0] = container.getReference();
for ( int i=0; i < searchFieldCount; i++) fields[i+1] = "%" + searchString + "%";
List countList = m_sql.dbRead(sql, fields, null);
if ( countList.isEmpty() ) return 0;
Object obj = countList.get(0);
String str = (String) obj;
return Integer.parseInt(str);
}
}
}
// No search fields - retrieve, filter and count
String sql = doubleStorageSql.getSelectXml5Sql(m_resourceTableName, m_resourceTableContainerIdField, null, false);
Object[] fields = new Object[1];
fields[0] = container.getReference();
List all = m_sql.dbRead(sql, fields, new SearchFilterReader(container, filter, null, true));
int count = all.size();
return count;
}
/**
* Get all Resources.
*
* @param container
* The container for this resource.
* @return The list (Resource) of all Resources.
*/
public List getAllResources(Entity container)
{
return getAllResources(container, null, null, true, null);
}
/**
* Get all Resources.
*
* @param container
* The container for this resource.
* @param filter
* conditional for select statement
* @return The list (Resource) of all Resources.
*/
public List getAllResources(Entity container, Filter filter)
{
return getAllResources(container, filter, null, true, null);
}
/**
* Get all Resources.
*
* @param container
* The container for this resource.
* @param sqlFilter
* conditional for select statement
* @return The list (Resource) of all Resources.
*/
public List getAllResources(Entity container, String sqlFilter)
{
return getAllResources(container, null, sqlFilter, true, null);
}
/**
* Deal with the fact that we can get a PagingPosition from a query filter
* or directly as a parameter. In time remove the option to use
* PagingPosition.
*/
// TODO: Remove all methods with PagingPostition and switch to Filter
private PagingPosition fixPagingPosition(Filter filter, PagingPosition pos)
{
if ( filter == null ) return pos;
if ( pos != null )
{
log.warn("The use of methods with PagingPosition should switch to using Search (SAK-13584) - Chuck");
return pos;
}
if ( filter instanceof Search )
{
Search q = (Search) filter;
if ( q.getLimit() > 0 && q.getLimit() >= q.getStart() )
{
return new PagingPosition((int) q.getStart(), (int) q.getLimit());
}
}
return null;
}
/**
* Get all Resources.
*
* @param container
* The container for this resource.
* @param softFilter
* an optional software filter
* @param sqlFilter
* an optional conditional for select statement
* @param asc
* true means ascending
* @param pager
* an optional range of elements to return inclusive
* @return The list (Resource) of all Resources.
*/
public List getAllResources(Entity container, Filter softFilter, String sqlFilter, boolean asc, PagingPosition pager) {
return getAllResources(container, softFilter, sqlFilter, asc, pager, null);
}
/**
* Get all Resources.
*
* @param container
* The container for this resource.
* @param softFilter
* an optional software filter
* @param sqlFilter
* an optional conditional for select statement
* @param asc
* true means ascending
* @param pager
* an optional range of elements to return inclusive
* @param bindVariables
* an optional list of bind variables
* @return The list (Resource) of all Resources.
*/
public List getAllResources(Entity container, Filter softFilter, String sqlFilter, boolean asc, PagingPosition pager, List
© 2015 - 2025 Weber Informatics LLC | Privacy Policy