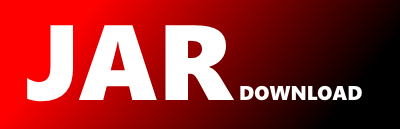
org.sakaiproject.util.Web Maven / Gradle / Ivy
/**********************************************************************************
* $URL$
* $Id$
***********************************************************************************
*
* Copyright (c) 2003, 2004, 2005, 2006, 2007, 2008 Sakai Foundation
*
* Licensed under the Educational Community License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.opensource.org/licenses/ECL-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*
**********************************************************************************/
package org.sakaiproject.util;
import java.io.ByteArrayOutputStream;
import java.io.PrintWriter;
import java.io.UnsupportedEncodingException;
import java.net.URLEncoder;
import java.util.Enumeration;
import java.util.regex.Matcher;
import java.util.regex.Pattern;
import javax.servlet.ServletConfig;
import javax.servlet.http.HttpServletRequest;
import lombok.extern.slf4j.Slf4j;
import org.sakaiproject.component.cover.ComponentManager;
import org.sakaiproject.tool.api.SessionManager;
/**
*
* Web is a web (html, http, etc) technlogies collection of helper methods.
*
* @deprecated use apache commons utils for {@link org.sakaiproject.util.api.FormattedText}, this will be removed after 2.9 - Dec 2011
*/
@Deprecated
@Slf4j
public class Web
{
// used to remove javascript from html
private static final String START_JAVASCRIPT = "");
}
/**
* Compute the URL that would return to this server based on the current request.
*
* Note: this method is duplicated in the /sakai-kernel-api/src/main/java/org/sakaiproject/util/RequestFilter.java
*
* @param req
* The request.
* @return The URL back to this server based on the current request.
* @deprecated use RequestFilter.serverUrl
*/
public static String serverUrl(HttpServletRequest req)
{
return RequestFilter.serverUrl(req);
}
public static String snoop(PrintWriter out, boolean html, ServletConfig config, HttpServletRequest req)
{
// if no out, send to system out
ByteArrayOutputStream ostream = null;
if (out == null)
{
ostream = new ByteArrayOutputStream();
out = new PrintWriter(ostream);
html = false;
}
String h1 = "";
String h1x = "";
String pre = "";
String prex = "";
String b = "";
String bx = "";
String p = "";
if (html)
{
h1 = "";
h1x = "
";
pre = "";
prex = "
";
b = "";
bx = "";
p = "";
}
Enumeration> e = null;
out.println(h1 + "Snoop for request" + h1x);
out.println(req.toString());
if (config != null)
{
e = config.getInitParameterNames();
if (e != null)
{
boolean first = true;
while (e.hasMoreElements())
{
if (first)
{
out.println(h1 + "Init Parameters" + h1x);
out.println(pre);
first = false;
}
String param = (String) e.nextElement();
out.println(" " + param + ": " + config.getInitParameter(param));
}
out.println(prex);
}
}
out.println(h1 + "Request information:" + h1x);
out.println(pre);
print(out, "Request method", req.getMethod());
String requestUri = req.getRequestURI();
print(out, "Request URI", requestUri);
displayStringChars(out, requestUri);
print(out, "Request protocol", req.getProtocol());
String servletPath = req.getServletPath();
print(out, "Servlet path", servletPath);
displayStringChars(out, servletPath);
String contextPath = req.getContextPath();
print(out, "Context path", contextPath);
displayStringChars(out, contextPath);
String pathInfo = req.getPathInfo();
print(out, "Path info", pathInfo);
displayStringChars(out, pathInfo);
print(out, "Path translated", req.getPathTranslated());
print(out, "Query string", req.getQueryString());
print(out, "Content length", req.getContentLength());
print(out, "Content type", req.getContentType());
print(out, "Server name", req.getServerName());
print(out, "Server port", req.getServerPort());
print(out, "Remote user", req.getRemoteUser());
print(out, "Remote address", req.getRemoteAddr());
// print(out, "Remote host", req.getRemoteHost());
print(out, "Authorization scheme", req.getAuthType());
out.println(prex);
e = req.getHeaderNames();
if (e.hasMoreElements())
{
out.println(h1 + "Request headers:" + h1x);
out.println(pre);
while (e.hasMoreElements())
{
String name = (String) e.nextElement();
out.println(" " + name + ": " + req.getHeader(name));
}
out.println(prex);
}
e = req.getParameterNames();
if (e.hasMoreElements())
{
out.println(h1 + "Servlet parameters (Single Value style):" + h1x);
out.println(pre);
while (e.hasMoreElements())
{
String name = (String) e.nextElement();
out.println(" " + name + " = " + req.getParameter(name));
}
out.println(prex);
}
e = req.getParameterNames();
if (e.hasMoreElements())
{
out.println(h1 + "Servlet parameters (Multiple Value style):" + h1x);
out.println(pre);
while (e.hasMoreElements())
{
String name = (String) e.nextElement();
String vals[] = (String[]) req.getParameterValues(name);
if (vals != null)
{
out.print(b + " " + name + " = " + bx);
out.println(vals[0]);
for (int i = 1; i < vals.length; i++)
out.println(" " + vals[i]);
}
out.println(p);
}
out.println(prex);
}
e = req.getAttributeNames();
if (e.hasMoreElements())
{
out.println(h1 + "Request attributes:" + h1x);
out.println(pre);
while (e.hasMoreElements())
{
String name = (String) e.nextElement();
out.println(" " + name + ": " + req.getAttribute(name));
}
out.println(prex);
}
if (ostream != null)
{
out.flush();
return ostream.toString();
}
return "";
}
/**
* Returns a hex representation of a byte.
*
* @param b
* The byte to convert to hex.
* @return The 2-digit hex value of the supplied byte.
*/
protected static final String toHex(byte b)
{
char ret[] = new char[2];
ret[0] = hexDigit((b >>> 4) & (byte) 0x0F);
ret[1] = hexDigit((b >>> 0) & (byte) 0x0F);
return new String(ret);
}
/**
** Encode filename (accomodating UTF-8 characters) for specific browser download/access
** Sadly, Mozilla uses a different encoding scheme than everyone else
** Sadly, Safari has a known bug where doesn't correctly translate encoding for user
**
** This method require inclusion of the javamail mail package.
** @deprecated It is now possible to specify encoded filenames for the browser
** see @link{Web#buildContentDisposition}
**/
public static String encodeFileName(HttpServletRequest req, String fileName )
{
String agent = req.getHeader("USER-AGENT");
try
{
if ( agent != null && agent.indexOf("MSIE")>=0 )
fileName = java.net.URLEncoder.encode(fileName, "UTF8");
else if ( agent != null && agent.indexOf("Mozilla")>=0 && agent.indexOf("Safari") == -1 )
fileName = javax.mail.internet.MimeUtility.encodeText(fileName, "UTF8", "B");
else
fileName = java.net.URLEncoder.encode(fileName, "UTF8");
}
catch (java.io.UnsupportedEncodingException e)
{
log.error(e.getMessage(), e);
}
return fileName;
}
/**
* This attempts to build the value of the content disposition header. It provides a ISO-8859-1 representation
* and a full UTF-8 version. This allows browser that understand the full version to use that and
* for mainly IE 8 the old limited one.
* @param filename The filename to encode
* @param isDownload Whether the file is a download, will use "attachment" if true and "inline" if false.
* @return The value of the content disposition header specifying it's inline content.
*/
public static String buildContentDisposition(String filename, boolean isDownload) {
try {
// This will replace all non US-ASCII characters with '?'
// Although this behaviour is unspecified doing it manually is overkill (too much work).
// Make sure we escape double quotes.
String iso8859Filename = new String(filename.getBytes("ISO-8859-1"), "ISO-8859-1")
.replace("\\", "\\\\")
.replace("\"", "\\\"");
String utf8Filename = URLEncoder.encode(filename, "UTF-8").replace("+", "%20");
return new StringBuilder()
.append(isDownload ? "attachment; " : "inline; ")
.append("filename=\"").append(iso8859Filename).append("\"; ")
// For sensible browser give them a full UTF-8 encoded string.
.append("filename*=UTF-8''").append(utf8Filename)
.toString();
} catch (UnsupportedEncodingException shouldNeverHappen) {
throw new RuntimeException(shouldNeverHappen);
}
}
private static String internalEscapeHtml(String value, boolean escapeNewlines) {
// FIXME this method needs to be removed entirely and is only here as a reference of how this used to work
if (value == null) return "";
try {
StringBuilder buf = new StringBuilder();
final int len = value.length();
for (int i = 0; i < len; i++)
{
char c = value.charAt(i);
switch (c)
{
case '<':
{
if (buf == null) buf = new StringBuilder(value.substring(0, i));
buf.append("<");
}
break;
case '>':
{
if (buf == null) buf = new StringBuilder(value.substring(0, i));
buf.append(">");
}
break;
case '&':
{
if (buf == null) buf = new StringBuilder(value.substring(0, i));
buf.append("&");
}
break;
case '"':
{
if (buf == null) buf = new StringBuilder(value.substring(0, i));
buf.append(""");
}
break;
case '\n':
{
if (escapeNewlines)
{
if (buf == null) buf = new StringBuilder(value.substring(0, i));
buf.append("
\n");
}
else
{
if (buf != null) buf.append(c);
}
}
break;
default:
{
if (c < 128)
{
if (buf != null) buf.append(c);
}
else
{
// escape higher Unicode characters using an
// HTML numeric character entity reference like "㴸"
if (buf == null) buf = new StringBuilder(value.substring(0, i));
buf.append("");
buf.append(Integer.toString((int) c));
buf.append(";");
}
}
break;
}
} // for
return (buf == null) ? value : buf.toString();
}
catch (Exception e)
{
return value;
}
}
/**
** Make sure any HTML is 'clean' (no javascript, invalid image tags)
**/
public static String cleanHtml( String htmlStr )
{
//KNL-610 if a null String is passed return to avoid NPE -DH
if (htmlStr == null)
{
return null;
}
// handle embedded images
htmlStr = htmlStr.replaceAll("
-1 && badEnd == -1)
badHtml = htmlStr.substring( badStart );
else
badHtml = htmlStr.substring( badStart, badEnd+END_JAVASCRIPT.length() );
// use replace( CharSequence, CharSequence) -- no regexp
htmlStr = htmlStr.replace( new StringBuilder(badHtml), new StringBuilder() );
}
return htmlStr;
}
protected static void displayStringChars(PrintWriter out, String str)
{
if (str == null)
{
out.print("null");
}
else
for (int i = 0; i < str.length(); i++)
{
int c = (int) str.charAt(i);
out.print(Integer.toHexString(c) + " ");
}
out.println();
}
}