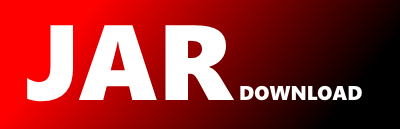
jcifs_1.3.3.docs.pipes.html Maven / Gradle / Ivy
Go to download
JCIFS is an Open Source client library that implements the CIFS/SMB networking protocol in 100% Java
Using JCIFS to Connect to Win32 Named Pipes
The Named Pipe client functions of the Windows SDK have been implemented with jCIFS (Named Pipes are implemented over SMB) allowing Java programs to directly communicate with Win32 Named Pipe server processes.
Note: Named Pipes are slow and less portable compared to sockets. There are few reasons why one might write a new application to use Win32 Named Pipes opposed to explicit network programming with Sockets.
There are several Win32 functions for sending and receiving data with Named Pipes all of which have been normalized into the Java XxxputStream interface however a different SmbNamedPipe constructor parameter is necessary depending on which style of messaging is to be used on the wire. These differences can be summarized by the type of Win32 functions that would be used had the client code been written for the Windows platform. The type of Named Pipe and it's attributes are specified when a server Named Pipe is created with a CreateNamedPipe Win32 call.
-
CallNamedPipe To use the CallNamedPipe style of messaging use the SmbFile.PIPE_TYPE_CALL flag.
-
TransactNamedPipe To use the TransactNamedPipe style of messaging use the SmbFile.PIPE_TYPE_TRANSACT flag.
-
CreateFile The traditional file I/O oriented Named Pipe messaging used by the CreateFile, WriteFile, and ReadFile Win32 functions is used when the SmbNamedPipe constructor is not passed either of the above mentioned flags.
See also:
-
Microsoft's Named Pipes SDK Documentation
-
JCIFS SmbNamedPipe API Documentation
-
Win32 Named Pipe Example Utilities
-
JCIFS SmbNamedPipe Example Programs
CallNamedPipe and TransactNamedPipe Style Named Pipes
There are several ways to obtain an InputStream and/or OutputStream with a Win32 Named Pipe server process. First, the open flags and mode of the server Named Pipe must be known. If for example, the following Win32 call was issued to create the server Named Pipe:
h = CreateNamedPipe("\\\\.\\PIPE\\foo",
PIPE_ACCESS_DUPLEX,
PIPE_TYPE_MESSAGE | PIPE_READMODE_MESSAGE | PIPE_WAIT,
10, bufferSize, bufferSize, NMPWAIT_WAIT_FOREVER, NULL);
This would create a Transact oriented Named Pipe. To connect to this type of Named Pipe, the corresponding jCIFS code could be used:
SmbNamedPipe pipe = new SmbNamedPipe( "smb://server/IPC$/PIPE/foo",
SmbNamedPipe.PIPE_TYPE_RDWR | SmbNamedPipe.PIPE_TYPE_CALL );
OutputStream out = pipe.getNamedPipeOutputStream();
InputStream in = pipe.getNamedPipeInputStream();
If a message is written to the OutputStream, it's response can then be read from the InputStream. Take care that the same SmbNamedPipe instance is used with getNamedPipeOutputStream and getNamedPipeInputStream or the streams will not be connected to the same Named Pipe server instance.
An alternative sample of jCIFS code that would provoke the TransactNamedPipe style of I/O would look like:
SmbNamedPipe pipe = new SmbNamedPipe( "smb://server/IPC$/foo",
SmbNamedPipe.PIPE_TYPE_RDWR | SmbNamedPipe.PIPE_TYPE_TRANSACT );
OutputStream out = pipe.getNamedPipeOutputStream();
InputStream in = pipe.getNamedPipeInputStream();
Again, writing to the OutputStream obtained from this pipe will result in data being available in it's InputStream. Also, note the lack of "/PIPE" in this SMB URL.
File I/O Style Named Pipes
The other type of Named Pipe can be created on the server by not specifying a pipe mode of PIPE_TYPE_MESSAGE | PIPE_READMODE_MESSAGE with the CreateNamedPipe Win32 function. With this file I/O oriented Named Pipe, the corresponding jCIFS code could be used:
SmbNamedPipe pipe = new SmbNamedPipe( "smb://server/IPC$/foo",
SmbNamedPipe.PIPE_TYPE_RDWR );
InputStream in = pipe.getNamedPipeInputStream();
OutputStream out = pipe.getNamedPipeOutputStream();
Notice that for file I/O oriented Named Pipes the PIPE_TYPE_TRANSACT parameter is not specified and there is no PIPE in the URL. Incedentally, SmbFileInputStream or SmbFileOutputStream may be used to read or write to this type of Named Pipe as one might do with a regular file. However you cannot read and write to the same Named Pipe server instance without using SmbNamedPipe to associate the streams.
Finally, if the server process is not ready to process the request a "All pipe instances are busy" error may result in which case it will be necessary to sleep momentarily and try again.
Running the CallNamedPipe.java Example Program
The examples/CallNamedPipe.java program and examples/pipes/createnp.exe utility can be used to explore Named Pipes and the jCIFS Named Pipe client functionality. They, along with several other Named Pipe example programs and Win32 utility programs, were used to test the jCIFS implementation of Named Pipes. Users trying to write their own jCIFS Named Pipe clients might consult these programs to gain a better understanding of how jCIFS works with respect to Named Pipes.
To run the CallNamedPipe example, which issues a Transact style Named Pipe call, create a Transact oriented Named Pipe with the createnp.exe utility like:
C:\TEMP> createnp
dwOpenMode
0x00000003 PIPE_ACCESS_DUPLEX
0x00000001 PIPE_ACCESS_INBOUND
0x00000002 PIPE_ACCESS_OUTBOUND
0x80000000 FILE_FLAG_WRITE_THROUGH
0x40000000 FILE_FLAG_OVERLAPPED
0x00040000 WRITE_DAC
0x00080000 WRITE_OWNER
0x01000000 ACCESS_SYSTEM_SECURITY
dwPipeMode
0x00000000 PIPE_TYPE_BYTE
0x00000004 PIPE_TYPE_MESSAGE
0x00000000 PIPE_READMODE_BYTE
0x00000002 PIPE_READMODE_MESSAGE
0x00000000 PIPE_WAIT
0x00000001 PIPE_NOWAIT
defaults
inFile = <read from pipe input>
outFile = <write to pipe output>
dwOpenMode = PIPE_ACCESS_DUPLEX
dwPipeMode = PIPE_TYPE_BYTE | PIPE_READMODE_BYTE | PIPE_WAIT
bufferSize = 65535
createnp \\.\pipe\name /I inFile /O outFile /M mode /P pmode /B bufferSize
C:\TEMP> createnp \\.\pipe\foo /P 0x6
The 0x6 for the dwPipeMode parameter was determined by ORing together the hexadecimal values for PIPE_TYPE_MESSAGE (0x4) and PIPE_READMODE_MESSAGE (0x2). The Windows Calculator program in Scientific mode can help you with generating these values. See the CreateNamedPipe Win32 SDK Documentation for the exact meaning of these parameters.
Now compile and run the CallNamedPipe.java program like:
$ java -Djcifs.properties=my.prp CallNamedPipe smb://server/IPC$/PIPE/foo in out
This will read a buffer full of the file in which will be echoed by the createnp server process and written to the out file. The createnp program will exit and report success:
C:\TEMP> createnp \\.\pipe\foo /P 0x6
Success: operation performed successfully
Last updated Dec 22, 2008
jcifs-1.3.2
Copyright © 2004 The JCIFS Project
validate this page
© 2015 - 2025 Weber Informatics LLC | Privacy Policy