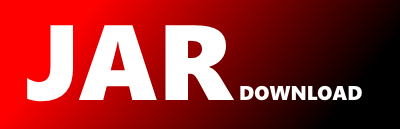
sbt.testing.Runner.scala Maven / Gradle / Ivy
package sbt.testing
/** Represents one run of a suite of tests.
*
* The run represented by a Runner
has a lifecycle. The run
* begins when the Runner
is instantiated by the framework and
* returned to the client during a Framework.runner
invocation.
* The run continues until the client invokes done
on the
* Runner
. Before invoking done
, the client can
* invoke the tasks
method as many times at it wants, but once
* done
has been invoked, the Runner
enters "spent"
* mode. Any subsequent invocations of tasks
will be met with an
* IllegalStateException
.
*
* In Scala.js, the client may request multiple instances of
* Runner
, where one of these instances is considered the master.
* The slaves receive a communication channel to the master. Once the master's
* done
method is invoked, nothing may be invoked on the slaves
* or the master. Slaves can be de-comissioned before the master terminates.
*/
trait Runner {
/** Returns an array of tasks that when executed will run tests and suites
* determined by the passed TaskDef
s.
*
*
* Each returned task, when executed, will run tests and suites determined by
* the test class name, fingerprints, "explicitly specified" field, and
* selectors of one of the passed TaskDef
s.
*
*
*
* This tasks
method may be called with TaskDef
s
* containing the same value for testClassName
but different
* fingerprints. For example, if both a class and its companion object were
* test classes, the tasks
method could be passed an array
* containing TaskDef
s with the same name but with a different
* value for fingerprint.isModule
.
*
*
*
* A test framework may "reject" a requested task by returning no
* Task
for that TaskDef
.
*
*
* @param taskDefs the TaskDef
s for requested tasks
* @return an array of Task
s
* @throws java.lang.IllegalStateException if invoked after done
* has been invoked.
*/
def tasks(taskDefs: Array[TaskDef]): Array[Task]
/** Indicates the client is done with this Runner
instance.
*
* After invoking the done
method on a Runner
* instance, the client should no longer invoke the task
methods
* on that instance. (If the client does invoke task
after
* done
, it will be rewarded with an
* IllegalStateException
.)
*
* Similarly, after returning from done
, the test framework
* should no longer write any messages to the Logger
, nor fire
* any more events to the EventHandler
, passed to
* Framework.runner
. If the test framework has not completed
* writing log messages or firing events when the client invokes
* done
, the framework should not return from done
* until it is finished sending messages and events, and may block the thread
* that invoked done
until it is actually done.
*
* In short, by invoking done
, the client indicates it is done
* invoking the task
methods for this run. By returning from
* done
, the test framework indicates it is done writing log
* messages and firing events for this run.
*
* If the client invokes done
more than once on the same
* Runner
instance, the test framework should on subsequent
* invocations should throw IllegalStateException
.
*
* The test framework may send a summary (i.e., a message giving
* total tests succeeded, failed, and so on) to the user via a log message.
* If so, it should return the summary from done
. If not, it
* should return an empty string. The client may use the return value of
* done
to decide whether to display its own summary message.
*
* The test framework may return a multi-lines string (i.e., a
* message giving total tests succeeded, failed and so on) to the client.
*
* In Scala.js, the client must not call this method before all execute
* methods of all Tasks have called their completion continuation. Otherwise,
* the Framework should throw an IllegalStateException (since it cannot
* block).
*
* Further, if this is the master, the client must not call this method
* before, all done methods of all slaves have returned (otherwise,
* IllegalStateException). If this is a slave, the returned string is ignored.
*
* @return a possibly multi-line summary string, or the empty string if no
* summary is provided
*/
def done(): String
/**
* Remote args that will be passed to Runner
in a sub-process as
* remoteArgs.
*
* @return an array of strings that will be passed to Runner
in
* a sub-process as remoteArgs
.
*/
def remoteArgs(): Array[String]
/** Returns the arguments that were used to create this Runner
.
*
* @return an array of argument that is used to create this Runner.
*/
def args: Array[String]
/** Scala.js specific: Invoked on the master Runner
, if a slave
* sends a message (through the channel provided by the client).
*
* The master may send a message back to the sending slave by returning the
* message in a Some.
*
* Invoked on a slave Runner
, if the master responds to a
* message (sent by the slave via the supplied closure in
* slaveRunner
). The return value of the call is ignored in
* this case.
*/
def receiveMessage(msg: String): Option[String]
/** Scala.js specific: Serialize a task created by tasks
or
* returned from execute
.
*
* The resulting string will be passed to the deserializeTask
* method of another runner. After calling this method, the passed task is
* invalid and should dissociate from this runner.
*/
def serializeTask(task: Task, serializer: TaskDef => String): String
/** Scala.js specific: Deserialize a task that has been serialized by
* serializeTask
of another or this Runner
.
*
* The resulting task must associate with this runner.
*/
def deserializeTask(task: String, deserializer: String => TaskDef): Task
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy