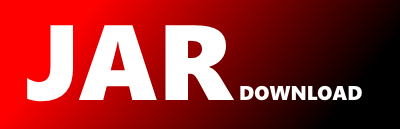
dotty.tools.dotc.transform.ReifiedReflect.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of scala3-compiler_3 Show documentation
Show all versions of scala3-compiler_3 Show documentation
scala3-compiler-bootstrapped
package dotty.tools.dotc
package transform
import core.*
import Decorators.*
import Flags.*
import Types.*
import Contexts.*
import Symbols.*
import NameKinds.*
import dotty.tools.dotc.ast.tpd
import tpd.*
import scala.collection.mutable
import dotty.tools.dotc.core.Annotations.*
import dotty.tools.dotc.core.Names.*
import dotty.tools.dotc.core.StdNames.*
import dotty.tools.dotc.quoted.*
import scala.annotation.constructorOnly
/** Helper methods to construct trees calling methods in `Quotes.reflect` based on the current `quotes` tree */
trait ReifiedReflect:
/** Stable reference to the instance of `scala.quoted.Quotes` */
def quotesTree: Tree
def self(using Context): Tree =
quotesTree.select(defn.Quotes_reflect)
/** Create type for `quotes.reflect.Term` */
def TermTpt(using Context) =
self.select(defn.Quotes_reflect_TermType)
/** Create type for `quotes.reflect.TypeTree` */
def TypeTreeTpt(using Context) =
self.select(defn.Quotes_reflect_TypeTreeType)
/** Create tree for `quotes.reflect.Apply(, List(*))` */
def Apply(fn: Tree, args: List[Tree])(using Context) =
val argTrees = tpd.mkList(args, TermTpt)
self.select(defn.Quotes_reflect_Apply)
.select(defn.Quotes_reflect_Apply_apply)
.appliedTo(fn, argTrees)
/** Create tree for `quotes.reflect.TypeApply(, List(*))` */
def TypeApply(fn: Tree, args: List[Tree])(using Context) =
val argTrees = tpd.mkList(args, TypeTreeTpt)
self.select(defn.Quotes_reflect_TypeApply)
.select(defn.Quotes_reflect_TypeApply_apply)
.appliedTo(fn, argTrees)
/** Create tree for `quotes.reflect.Assign(, )` */
def Assign(lhs: Tree, rhs: Tree)(using Context) =
self.select(defn.Quotes_reflect_Assign)
.select(defn.Quotes_reflect_Assign_apply)
.appliedTo(lhs, rhs)
/** Create tree for `quotes.reflect.Inferred()` */
def Inferred(typeTree: Tree)(using Context) =
self.select(defn.Quotes_reflect_Inferred)
.select(defn.Quotes_reflect_Inferred_apply)
.appliedTo(typeTree)
/** Create tree for `quotes.reflect.Literal()` */
def Literal(constant: Tree)(using Context) =
self.select(defn.Quotes_reflect_Literal)
.select(defn.Quotes_reflect_Literal_apply)
.appliedTo(constant)
/** Create tree for `quotes.reflect.TypeRepr.of(Type.of[](quotes))` */
def TypeReprOf(tpe: Type)(using Context) =
self.select(defn.Quotes_reflect_TypeRepr)
.select(defn.Quotes_reflect_TypeRepr_of)
.appliedToType(tpe)
.appliedTo(
tpd.Quote(TypeTree(tpe), Nil)
.select(nme.apply)
.appliedTo(quotesTree)
)
/** Create tree for `quotes.reflect.TypeRepr.typeConstructorOf()` */
def TypeRepr_typeConstructorOf(classTree: Tree)(using Context) =
self.select(defn.Quotes_reflect_TypeRepr)
.select(defn.Quotes_reflect_TypeRepr_typeConstructorOf)
.appliedTo(classTree)
/** Create tree for `quotes.reflect.asTerm()` */
def asTerm(expr: Tree)(using Context) =
self.select(defn.Quotes_reflect_asTerm)
.appliedTo(expr)
/** Create tree for `quotes.reflect.TypeReprMethods.asType()` */
def asType(tpe: Type)(typeRepr: Tree)(using Context) =
self.select(defn.Quotes_reflect_TypeReprMethods)
.select(defn.Quotes_reflect_TypeReprMethods_asType)
.appliedTo(typeRepr)
.asInstance(defn.QuotedTypeClass.typeRef.appliedTo(tpe))
/** Create tree for `quotes.reflect.TreeMethods.asExpr().asInstanceOf[]` */
def asExpr(tpe: Type)(term: Tree)(using Context) =
self.select(defn.Quotes_reflect_TreeMethods)
.select(defn.Quotes_reflect_TreeMethods_asExpr)
.appliedTo(term)
.asInstance(defn.QuotedExprClass.typeRef.appliedTo(tpe))
end ReifiedReflect
© 2015 - 2025 Weber Informatics LLC | Privacy Policy