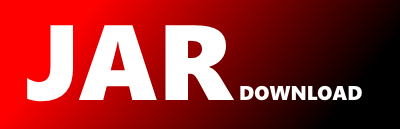
ncsa.hdf.object.fits.FitsFile Maven / Gradle / Ivy
/*****************************************************************************
* Copyright by The HDF Group. *
* Copyright by the Board of Trustees of the University of Illinois. *
* All rights reserved. *
* *
* This file is part of the HDF Java Products distribution. *
* The full copyright notice, including terms governing use, modification, *
* and redistribution, is contained in the files COPYING and Copyright.html. *
* COPYING can be found at the root of the source code distribution tree. *
* Or, see http://hdfgroup.org/products/hdf-java/doc/Copyright.html. *
* If you do not have access to either file, you may request a copy from *
* [email protected]. *
****************************************************************************/
package ncsa.hdf.object.fits;
import java.io.DataInput;
import java.io.IOException;
import java.io.InputStream;
import java.io.RandomAccessFile;
import javax.swing.tree.DefaultMutableTreeNode;
import javax.swing.tree.MutableTreeNode;
import javax.swing.tree.TreeNode;
import ncsa.hdf.object.Dataset;
import ncsa.hdf.object.Datatype;
import ncsa.hdf.object.FileFormat;
import ncsa.hdf.object.Group;
import ncsa.hdf.object.HObject;
import nom.tam.fits.AsciiTableHDU;
import nom.tam.fits.BasicHDU;
import nom.tam.fits.BinaryTableHDU;
import nom.tam.fits.Fits;
import nom.tam.fits.ImageHDU;
import nom.tam.fits.RandomGroupsHDU;
import nom.tam.fits.TableHDU;
/**
* This class provides file level APIs. File access APIs include retrieving the
* file hierarchy, opening and closing file, and writing file content to disk.
*
* @version 2.4 9/4/2007
* @author Peter X. Cao
*/
public class FitsFile extends FileFormat
{
private static final long serialVersionUID = -1965689032980605791L;
/**
* The root node of the file hierearchy.
*/
private MutableTreeNode rootNode;
/** the fits file */
private Fits fitsFile;
private static boolean isFileOpen;
/**
* Constructs an empty FitsFile with read-only access.
*/
public FitsFile() {
this("");
}
/**
* Constructs an FitsFile object of given file name with read-only access.
*/
public FitsFile(String pathname) {
super(pathname);
isReadOnly = true;
isFileOpen = false;
this.fid = -1;
try { fitsFile = new Fits(fullFileName); }
catch (Exception ex) {}
}
/**
* Checks if the given file format is a Fits file.
*
* @param fileformat the fileformat to be checked.
* @return true if the given file is an Fits file; otherwise returns false.
*/
@Override
public boolean isThisType(FileFormat fileformat) {
return (fileformat instanceof FitsFile);
}
/**
* Checks if a given file is a Fits file.
*
* @param filename the file to be checked.
* @return true if the given file is an Fits file; otherwise returns false.
*/
@Override
public boolean isThisType(String filename)
{
boolean is_fits = false;
RandomAccessFile raf = null;
try { raf = new RandomAccessFile(filename, "r"); }
catch (Exception ex) { raf = null; }
if (raf == null) {
try { raf.close();} catch (Exception ex) {}
return false;
}
byte[] header = new byte[80];
try { raf.read(header); }
catch (Exception ex) { header = null; }
if (header != null)
{
String front = new String(header, 0, 9);
if (!front.startsWith("SIMPLE =")) {
try { raf.close();} catch (Exception ex) {}
return false;
}
String back = new String(header, 9, 70);
back = back.trim();
if ((back.length() < 1) || (back.charAt(0) != 'T')) {
try { raf.close();} catch (Exception ex) {}
return false;
}
is_fits = true;;
}
try { raf.close();} catch (Exception ex) {}
return is_fits;
}
/**
* Creates a FitsFile instance with specified file name and READ access.
*
* @param pathname the full path name of the file.
* Regardless of specified access, the FitsFile implementation uses
* READ.
*
* @see ncsa.hdf.object.FileFormat@createInstance(java.lang.String, int)
*/
@Override
public FileFormat createInstance(String filename, int access)
throws Exception {
return new FitsFile(filename);
}
// Implementing FileFormat
@Override
public int open() throws Exception {
if (!isFileOpen) {
isFileOpen = true;
rootNode = loadTree();
}
return 0;
}
private MutableTreeNode loadTree() {
long[] oid = {0};
FitsGroup rootGroup = new FitsGroup(
this,
"/",
null, // root node does not have a parent path
null, // root node does not have a parent node
oid);
DefaultMutableTreeNode root = new DefaultMutableTreeNode(rootGroup) {
private static final long serialVersionUID = 5556789624491863365L;
@Override
public boolean isLeaf() { return false; }
};
if (fitsFile == null) {
return root;
}
BasicHDU[] hdus = null;
try { hdus = fitsFile.read(); }
catch (Exception ex) {}
if (hdus == null) {
return root;
}
int n = hdus.length;
int nImageHDU = 0;
int nTableHDU = 0;
String hduName = null;
BasicHDU hdu = null;
for (int i=0; i
* @param obj the object which the attribute is to be attached to.
* @param attr the atribute to attach.
* @param attrExisted The indicator if the given attribute exists.
* @return true if successful and false otherwise.
*/
@Override
public void writeAttribute(HObject obj, ncsa.hdf.object.Attribute attr,
boolean attrExisted) throws Exception {
// not supported
throw new UnsupportedOperationException("Unsupported operation.");
}
/**
* Returns the version of the library.
*/
@Override
public String getLibversion()
{
String ver = "Fits Java (version 2.4)";
return ver;
}
// implementing FileFormat
@Override
public HObject get(String path) throws Exception
{
throw new UnsupportedOperationException("get() is not supported");
}
}