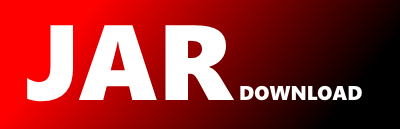
org.specs.xml.Xhtml.scala Maven / Gradle / Ivy
Go to download
Show more of this group Show more artifacts with this name
Show all versions of specs_2.8.0.Beta1-RC8
Show all versions of specs_2.8.0.Beta1-RC8
specs is a Behaviour-Driven-Design
framework
The newest version!
/**
* Copyright (c) 2007-2009 Eric Torreborre
*
* Permission is hereby granted, free of charge, to any person obtaining a copy of this software and associated
* documentation files (the "Software"), to deal in the Software without restriction, including without limitation
* the rights to use, copy, modify, merge, publish, distribute, sublicense, and/or sell copies of the Software,
* and to permit persons to whom the Software is furnished to do so, subject to the following conditions:
*
* The above copyright notice and this permission notice shall be included in all copies or substantial portions of
* the Software. Neither the name of specs nor the names of its contributors may be used to endorse or promote
* products derived from this software without specific prior written permission.
*
* THE SOFTWARE IS PROVIDED "AS IS", WITHOUT WARRANTY OF ANY KIND, EXPRESS OR IMPLIED, INCLUDING BUT NOT LIMITED
* TO THE WARRANTIES OF MERCHANTABILITY, FITNESS FOR A PARTICULAR PURPOSE AND NONINFRINGEMENT. IN NO EVENT SHALL
* THE AUTHORS OR COPYRIGHT HOLDERS BE LIABLE FOR ANY CLAIM, DAMAGES OR OTHER LIABILITY, WHETHER IN AN ACTION OF
* CONTRACT, TORT OR OTHERWISE, ARISING FROM, OUT OF OR IN CONNECTION WITH THE SOFTWARE OR THE USE OR OTHER
* DEALINGS IN THE SOFTWARE.
*/
package org.specs.xml
import scala.xml._
import scala.Math._
/**
* utility functions on Xhtml elements.
*
* - spanLastTd: adds a col span on the last td or th element of each row so that it spans the entire table
* - maxColSize: finds the maximum number of columns in a table
*/
trait Xhtml { outer =>
/** add a colspan on the last td or th of table rows, equal to the maximum number of columns. */
def spanLastTd(nodes: NodeSeq): NodeSeq = spanLastTd(nodes, maxColSize(nodes))
private def spanLastTd(nodes: NodeSeq, spanSize: Int): NodeSeq = {
nodes.toList match {
case List({ b } ) => {b} % nodes.toList.head.attributes
case List({ b } ) => {b} % nodes.toList.head.attributes
case List({ b } , Text(x)) => {b} % nodes.toList.head.attributes ++ Text(x)
/** don't set a colspan on the last cell of the biggest row */
case { b } :: otherThs if (nodes.toList.size < spanSize) => nodes.toList.head ++ spanLastTd(otherThs, spanSize)
case { b } :: otherTds if (nodes.toList.size < spanSize) => nodes.toList.head ++ spanLastTd(otherTds, spanSize)
case List({ x @ _*}
) => {spanLastTd(x, spanSize)}
% nodes.toList.head.attributes
case { y @ _*} :: otherRows => {spanLastTd(y, spanSize)} ++ spanLastTd(otherRows, spanSize)
case Text(x) :: other => Text(x) ++ spanLastTd(other, spanSize)
case other => other
}
}
/** @return the maximum number of columns in a table. */
def maxColSize(nodes: NodeSeq): Int = maxColSize(nodes, 0)
/** @return the maximum number of columns in a table, given a previously computed maximum */
private def maxColSize(nodes: NodeSeq, maximum: Int): Int = {
nodes.toList match {
case List({ b @ _* } ) => maximum + 1
case List({ b @ _* } , Text(x)) => maximum + 1
case { b @ _* } :: others => maxColSize(others, maximum + 1)
case List({ b @ _* } ) => maximum + 1
case List({ b @ _* } , Text(x)) => maximum + 1
case { b @ _* } :: others => maxColSize(others, maximum + 1)
case List({ x @ _*}
) => maxColSize(x, maximum)
case { y @ _*} :: otherRows => max(maxColSize(y, maximum), maxColSize(otherRows, maximum))
case Text(x) :: other => maxColSize(other, maximum)
case other => maximum
}
}
/** @return an unsorted html list from a list of objects */
def itemize[T](list: T*)= {
list.map(""+_).mkString("\n", "
\n", "\n")
}
/** include a NodeSeq in a collapsible section with a title */
def collapsible(title: String, content: NodeSeq): String = collapsible(title, content.toString)
/** include a String in a collapsible section with a title */
def collapsible(title: String, content: String): String = collapsible(System.nanoTime, title, content)
/** create a collapsible section with a given id for a NodeSeq */
private[specs] def collapsible(id: Long, title: String, content: NodeSeq): String = collapsible(id, title, 5, content.toString)
/** create a collapsible section with a given id for a String */
private[specs] def collapsible(id: Long, title: String, content: String): String = collapsible(id, title, 5, content)
/** create a collapsible section with a given id for a String and a specific header type */
private[specs] def collapsible(id: Long, title: String, titleNumber: Int, content: String): String = {
"\n"+
"
"+title+" \n"+
" \n"+
""
}
/**
* implicit to allow any piece of xml to be transformed to a collapsible section
*/
implicit def toXhtmlCollapsible(content: => { def toXhtml: NodeSeq }) = new Collapsible(content.toXhtml.toString)
/**
* implicit to allow any object with a toString method to be transformed to a collapsible section
*/
implicit def toCollapsible(content: =>Any) = new Collapsible(content.toString)
/**
* implicit class holding the "collapsible" method
*/
class Collapsible(content: =>String) {
def collapsible(title: String) = outer.collapsible(title, content)
}
}
object Xhtml extends Xhtml
© 2015 - 2025 Weber Informatics LLC | Privacy Policy