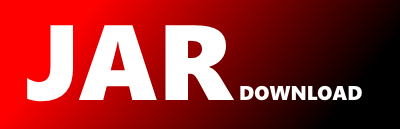
org.scalactic.Bool.scala Maven / Gradle / Ivy
/*
* Copyright 2001-2013 Artima, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.scalactic
/**
* A trait that represent a rich-featured boolean value, which includes the following members:
*
*
* - a boolean value
* - methods useful for failure messages construction
* - logical expression methods that makes
Bool
composable
*
*
* Bool
is used by code generated from BooleanMacro
(which AssertionsMacro
and RequirementsMacro
uses),
* it needs to be public so that the generated code can be compiled. It is expected that ScalaTest users would ever need to use Bool
directly.
*/
trait Bool {
private def makeString(raw: String, args: Array[Any]): String =
Resources.formatString(raw, args.map(Prettifier.default))
/**
* Construct and return failure message, by applying arguments returned from failureMessageArgs
to
* raw message returned from rawFailureMessage
*/
def failureMessage: String =
if (failureMessageArgs.isEmpty) rawFailureMessage else makeString(rawFailureMessage, failureMessageArgs.toArray)
/**
* Construct and return negated failure message, by applying arguments returned from negatedFailureMessageArgs
to
* raw message returned from rawNegatedFailureMessage
*/
def negatedFailureMessage: String =
if (negatedFailureMessageArgs.isEmpty) rawNegatedFailureMessage else makeString(rawNegatedFailureMessage, negatedFailureMessageArgs.toArray)
/**
* Construct and return mid sentence failure message, by applying arguments returned from midSentenceFailureMessageArgs
to
* raw message returned from rawMidSentenceFailureMessage
*/
def midSentenceFailureMessage: String =
if (midSentenceFailureMessageArgs.isEmpty) rawMidSentenceFailureMessage else makeString(rawMidSentenceFailureMessage, midSentenceFailureMessageArgs.toArray)
/**
* Construct and return mid sentence negated failure message, by applying arguments returned from midSentenceNegatedFailureMessageArgs
to
* raw message returned from rawMidSentenceNegatedFailureMessage
*/
def midSentenceNegatedFailureMessage: String =
if (midSentenceNegatedFailureMessageArgs.isEmpty) rawMidSentenceNegatedFailureMessage else makeString(rawMidSentenceNegatedFailureMessage, midSentenceNegatedFailureMessageArgs.toArray)
/**
* the Boolean
value of this Bool
*/
def value: Boolean
/**
* raw message to report a failure
*/
def rawFailureMessage: String
/**
* raw message with a meaning opposite to that of the failure message
*/
def rawNegatedFailureMessage: String
/**
* raw mid sentence message to report a failure
*/
def rawMidSentenceFailureMessage: String
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*/
def rawMidSentenceNegatedFailureMessage: String
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
.
*/
def failureMessageArgs: IndexedSeq[Any]
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
.
*/
def negatedFailureMessageArgs: IndexedSeq[Any]
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*/
def midSentenceFailureMessageArgs: IndexedSeq[Any]
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*/
def midSentenceNegatedFailureMessageArgs: IndexedSeq[Any]
/**
* Logical and
this Bool
with another Bool
*
* @param bool another Bool
* @return a Bool
that represents the result of logical and
*/
def &&(bool: Bool): Bool =
if (value)
new AndBool(this, bool)
else
this
/**
* Logical and
this Bool
with another Bool
*
* @param bool another Bool
* @return a Bool
that represents the result of logical and
*/
def &(bool: Bool): Bool = &&(bool)
/**
* Logical or
this Bool
with another Bool
*
* @param bool another Bool
* @return a Bool
that represents the result of logical or
*/
def ||(bool: => Bool): Bool = new OrBool(this, bool)
/**
* Logical or
this Bool
with another Bool
*
* @param bool another Bool
* @return a Bool
that represents the result of logical or
*/
def |(bool: => Bool): Bool = ||(bool)
/**
* Negate this Bool
*
* @return a Bool
that represents the result of negating the original Bool
*/
def unary_! : Bool = new NotBool(this)
}
/**
* Bool
companion object that provides factory methods to create different sub types of Bool
*
* Bool
is used by code generated from BooleanMacro
(which AssertionsMacro
and RequirementsMacro
uses),
* it needs to be public so that the generated code can be compiled. It is expected that ScalaTest users would ever need to use Bool
directly.
*/
object Bool {
/**
* Create a negated version of the given Bool
*
* @param bool the given Bool
* @return a negated version of the given Bool
*/
def notBool(bool: Bool): Bool = new NotBool(bool)
/**
* Create simple macro Bool
that is used by BooleanMacro
to wrap an unrecognized Boolean
expression.
*
* @param expression the Boolean
expression
* @param expressionText the original expression text (source code)
* @return a simple macro Bool
*/
def simpleMacroBool(expression: Boolean, expressionText: String): Bool = new SimpleMacroBool(expression, expressionText)
/**
* Create binary macro Bool
that is used by BooleanMacro
to wrap a recognized Boolean
expression, which includes Boolean
expression that
* uses ==
, ===
, !=
, !==
, >
, >=
, <
, <=
, &&
,
* &
, ||
and |
.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param right the right-hand-side (RHS) of the Boolean
expression
* @param expression the Boolean
expression
* @return a binary macro Bool
*/
def binaryMacroBool(left: Any, operator: String, right: Any, expression: Boolean): Bool = new BinaryMacroBool(left, operator, right, expression)
/**
* Overloaded method that takes a Bool
in place of Boolean
expression to create a new binary macro Bool
.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param right the right-hand-side (RHS) of the Boolean
expression
* @param bool the Bool
that will provide the Boolean
expression value with bool.value
* @return a binary macro Bool
*/
def binaryMacroBool(left: Any, operator: String, right: Any, bool: Bool): Bool = new BinaryMacroBool(left, operator, right, bool)
/**
* Create unary macro Bool
that is used by BooleanMacro
to wrap a recognized Boolean
expression represented by a unary method call,
* which includes Boolean
expression that uses isEmpty
.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param expression the Boolean
expression
* @return a unary macro Bool
*/
def unaryMacroBool(left: Any, operator: String, expression: Boolean): Bool =
new UnaryMacroBool(left, operator, expression)
/**
* Overloaded method that takes a Bool
in place of Boolean
expression to create a new unary macro Bool
.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param bool the Bool
that will provide the Boolean
expression value with bool.value
* @return a binary macro Bool
*/
def unaryMacroBool(left: Any, operator: String, bool: Bool): Bool =
new UnaryMacroBool(left, operator, bool.value)
/**
* Create macro Bool
that is used by BooleanMacro
to wrap a recognized Boolean
expression
* represented by a isInstanceOf
method call,
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param className the class name passed to isInstanceOf
method call
* @param expression the Boolean
expression
* @return a Bool
instance that represents a isInstanceOf
method call
*/
def isInstanceOfMacroBool(left: Any, operator: String, className: String, expression: Boolean): Bool =
new IsInstanceOfMacroBool(left, operator, className, expression)
/**
* Overloaded method that takes a Bool
in place of Boolean
expression to create a new isInstanceOf
* macro Bool
.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param className the class name passed to isInstanceOf
method call
* @param bool the Bool
that will provide the Boolean
expression value with bool.value
* @return a Bool
instance that represents a isInstanceOf
method call
*/
def isInstanceOfMacroBool(left: Any, operator: String, className: String, bool: Bool): Bool =
new IsInstanceOfMacroBool(left, operator, className, bool.value)
/**
* Create macro Bool
that is used by BooleanMacro
to wrap a recognized Boolean
expression
* represented by length
and size
method call,
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param actual the actual value returned from length
or size
method call
* @param expected the expected value returned from length
or size
method call
* @return a Bool
instance that represents a length
or size
method call
*/
def lengthSizeMacroBool(left: Any, operator: String, actual: Any, expected: Any): Bool =
new LengthSizeMacroBool(left, operator, actual, expected)
/**
* Create exists macro Bool
that is used by BooleanMacro
to wrap a recognized Boolean
expression
* represented by exists
method call.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param right the right-hand-side (RHS) of the Boolean
expression
* @param expression the Boolean
expression
* @return a exists macro Bool
*/
def existsMacroBool(left: Any, right: Any, expression: Boolean): Bool =
new ExistsMacroBool(left, right, expression)
/**
* A helper method to check is the given Bool
is a simple macro Bool
and contains empty expression text.
*
* @param bool the Bool
to check
* @return true
if the given Bool
is a simple macro Bool
and contains empty expression text, false
otherwise.
*/
def isSimpleWithoutExpressionText(bool: Bool): Boolean =
bool match {
case s: org.scalactic.SimpleMacroBool if s.expressionText.isEmpty => true
case _ => false
}
}
private[scalactic] class SimpleBool(expression: Boolean) extends Bool {
/**
* the Boolean
value of this Bool
*/
val value: Boolean = expression
/**
* raw message to report a failure
*
* @return Localized string for "Expression was false"
*/
def rawFailureMessage: String = Resources.rawExpressionWasFalse
/**
* raw message with a meaning opposite to that of the failure message
*
* @return Localized string for "Expression was true"
*/
def rawNegatedFailureMessage: String = Resources.rawExpressionWasTrue
/**
* raw mid sentence message to report a failure
*
* @return Localized string for "Expression was false"
*/
def rawMidSentenceFailureMessage: String = Resources.rawExpressionWasFalse
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*
* @return Localized string for "Expression was false"
*/
def rawMidSentenceNegatedFailureMessage: String = Resources.rawExpressionWasTrue
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
.
*
* @return empty Vector
*/
def failureMessageArgs: IndexedSeq[Any] = Vector.empty
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
.
*
* @return empty Vector
*/
def negatedFailureMessageArgs: IndexedSeq[Any] = Vector.empty
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*
* @return empty Vector
*/
def midSentenceFailureMessageArgs: IndexedSeq[Any] = Vector.empty
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*
* @return empty Vector
*/
def midSentenceNegatedFailureMessageArgs: IndexedSeq[Any] = Vector.empty
}
/**
* Bool that represents the result of logical AND
of two Bool
.
*
* @param bool1 the first Bool
* @param bool2 the second Bool
*/
private[scalactic] class AndBool(bool1: Bool, bool2: Bool) extends Bool {
/**
* the result of bool1.value
logical AND
bool2.value
*/
lazy val value: Boolean = bool1.value && bool2.value
/**
* raw message to report a failure
*
* @return Localized raw string for "{0}, but {1}"
*/
def rawFailureMessage: String = Resources.rawCommaBut
/**
* raw message with a meaning opposite to that of the failure message
*
* @return Localized raw string for "{0}, and {1}"
*/
def rawNegatedFailureMessage: String = Resources.rawCommaAnd
/**
* raw mid sentence message to report a failure
*
* @return Localized raw string for "{0}, but {1}"
*/
def rawMidSentenceFailureMessage: String = Resources.rawCommaBut
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*
* @return Localized raw string for "{0}, and {1}"
*/
def rawMidSentenceNegatedFailureMessage: String = Resources.rawCommaAnd
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
.
*
* @return Vector
that contains bool1.negatedFailureMessage
and bool2.midSentenceFailureMessage
*/
def failureMessageArgs = Vector(bool1.negatedFailureMessage, bool2.midSentenceFailureMessage)
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
.
*
* @return Vector
that contains bool1.negatedFailureMessage
and bool2.midSentenceNegatedFailureMessage
*/
def negatedFailureMessageArgs = Vector(bool1.negatedFailureMessage, bool2.midSentenceNegatedFailureMessage)
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*
* @return Vector
that contains bool1.midSentenceNegatedFailureMessage
and bool2.midSentenceFailureMessage
*/
def midSentenceFailureMessageArgs = Vector(bool1.midSentenceNegatedFailureMessage, bool2.midSentenceFailureMessage)
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*
* @return Vector
that contains bool1.midSentenceNegatedFailureMessage
and bool2.midSentenceNegatedFailureMessage
*/
def midSentenceNegatedFailureMessageArgs = Vector(bool1.midSentenceNegatedFailureMessage, bool2.midSentenceNegatedFailureMessage)
}
/**
* Bool that represents the result of logical OR
of two Bool
.
*
* @param bool1 the first Bool
* @param bool2 the second Bool
*/
private[scalactic] class OrBool(bool1: Bool, bool2: Bool) extends Bool {
/**
* the result of bool1.value
logical OR
bool2.value
*/
lazy val value: Boolean = bool1.value || bool2.value
/**
* raw message to report a failure
*
* @return Localized raw string for "{0}, and {1}"
*/
def rawFailureMessage: String = Resources.rawCommaAnd
/**
* raw message with a meaning opposite to that of the failure message
*
* @return Localized raw string for "{0}, and {1}"
*/
def rawNegatedFailureMessage: String = Resources.rawCommaAnd
/**
* raw mid sentence message to report a failure
*
* @return Localized raw string for "{0}, and {1}"
*/
def rawMidSentenceFailureMessage: String = Resources.rawCommaAnd
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*
* @return Localized raw string for "{0}, and {1}"
*/
def rawMidSentenceNegatedFailureMessage: String = Resources.rawCommaAnd
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
.
*
* @return Vector
that contains bool1.failureMessage
and bool2.midSentenceFailureMessage
*/
def failureMessageArgs = Vector(bool1.failureMessage, bool2.midSentenceFailureMessage)
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
.
*
* @return Vector
that contains bool1.failureMessage
and bool2.midSentenceNegatedFailureMessage
*/
def negatedFailureMessageArgs = Vector(bool1.failureMessage, bool2.midSentenceNegatedFailureMessage)
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*
* @return Vector
that contains bool1.midSentenceFailureMessage
and bool2.midSentenceFailureMessage
*/
def midSentenceFailureMessageArgs = Vector(bool1.midSentenceFailureMessage, bool2.midSentenceFailureMessage)
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*
* @return Vector
that contains bool1.midSentenceFailureMessage
and bool2.midSentenceNegatedFailureMessage
*/
def midSentenceNegatedFailureMessageArgs = Vector(bool1.midSentenceFailureMessage, bool2.midSentenceNegatedFailureMessage)
}
private[scalactic] class NotBool(bool: Bool) extends Bool {
val value: Boolean = !bool.value
/**
* raw message to report a failure
*
* @return the passed in bool.rawNegatedFailureMessage
*/
def rawFailureMessage: String = bool.rawNegatedFailureMessage
/**
* raw message with a meaning opposite to that of the failure message
*
* @return the passed in bool.rawFailureMessage
*/
def rawNegatedFailureMessage: String = bool.rawFailureMessage
/**
* raw mid sentence message to report a failure
*
* @return the passed in bool.rawMidSentenceNegatedFailureMessage
*/
def rawMidSentenceFailureMessage: String = bool.rawMidSentenceNegatedFailureMessage
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*
* @return the passed in bool.rawMidSentenceFailureMessage
*/
def rawMidSentenceNegatedFailureMessage: String = bool.rawMidSentenceFailureMessage
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
.
*
* @return the passed in bool.negatedFailureMessageArgs
*/
def failureMessageArgs: IndexedSeq[Any] = bool.negatedFailureMessageArgs
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
.
*
* @return the passed in bool.failureMessageArgs
*/
def negatedFailureMessageArgs: IndexedSeq[Any] = bool.failureMessageArgs
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*
* @return the passed in bool.midSentenceNegatedFailureMessageArgs
*/
def midSentenceFailureMessageArgs: IndexedSeq[Any] = bool.midSentenceNegatedFailureMessageArgs
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*
* @return the passed in bool.midSentenceFailureMessageArgs
*/
def midSentenceNegatedFailureMessageArgs: IndexedSeq[Any] = bool.midSentenceFailureMessageArgs
}
/**
* Simple macro Bool
that is used by BooleanMacro
to wrap an unrecognized Boolean
expression.
*
* @param expression the Boolean
expression
* @param expressionText the original expression text (source code)
*/
private[scalactic] class SimpleMacroBool(expression: Boolean, val expressionText: String) extends Bool {
/**
* the Boolean
value of this Bool
, holding the passed in expression value.
*/
val value: Boolean = expression
/**
* raw message to report a failure
*
* @return Localized raw string of "Expression was false" if passed in expressionText
is empty, else "{0} was false"
*/
def rawFailureMessage: String = if (expressionText.isEmpty) Resources.rawExpressionWasFalse else Resources.rawWasFalse
/**
* raw message with a meaning opposite to that of the failure message
*
* @return Localized raw string of "Expression was true" if passed in expressionText
is empty, else "{0} was true"
*/
def rawNegatedFailureMessage: String = if (expressionText.isEmpty) Resources.rawExpressionWasTrue else Resources.rawWasTrue
/**
* raw mid sentence message to report a failure
*
* @return Localized raw string of "Expression was false" if passed in expressionText
is empty, else "{0} was false"
*/
def rawMidSentenceFailureMessage: String = if (expressionText.isEmpty) Resources.rawExpressionWasFalse else Resources.rawWasFalse
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*
* @return Localized raw string of "Expression was true" if passed in expressionText
is empty, else "{0} was true"
*/
def rawMidSentenceNegatedFailureMessage: String = if (expressionText.isEmpty) Resources.rawExpressionWasTrue else Resources.rawWasTrue
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
.
*
* @return empty Vector
if passed in expressionText
is empty, else Vector
that contains the unquoted expressionText
*/
def failureMessageArgs: IndexedSeq[Any] = if (expressionText.isEmpty) Vector.empty else Vector(UnquotedString(expressionText))
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
.
*
* @return empty Vector
if passed in expressionText
is empty, else Vector
that contains the unquoted expressionText
*/
def negatedFailureMessageArgs: IndexedSeq[Any] = if (expressionText.isEmpty) Vector.empty else Vector(UnquotedString(expressionText))
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*
* @return empty Vector
if passed in expressionText
is empty, else Vector
that contains the unquoted expressionText
*/
def midSentenceFailureMessageArgs: IndexedSeq[Any] = if (expressionText.isEmpty) Vector.empty else Vector(UnquotedString(expressionText))
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*
* @return empty Vector
if passed in expressionText
is empty, else Vector
that contains the unquoted expressionText
*/
def midSentenceNegatedFailureMessageArgs: IndexedSeq[Any] = if (expressionText.isEmpty) Vector.empty else Vector(UnquotedString(expressionText))
}
/**
* Binary macro Bool
that is used by BooleanMacro
to wrap a recognized Boolean
expression, which includes Boolean
expression that
* uses ==
, ===
, !=
, !==
, >
, >=
, <
, <=
, &&
,
* &
, ||
and |
.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param right the right-hand-side (RHS) of the Boolean
expression
* @param expression the Boolean
expression
*/
private[scalactic] class BinaryMacroBool(left: Any, operator: String, right: Any, expression: Boolean) extends Bool {
/**
* Overloaded constructor that takes a Bool
in place of Boolean
expression.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param right the right-hand-side (RHS) of the Boolean
expression
* @param bool the Bool
that will provide the Boolean
expression value with bool.value
*/
def this(left: Any, operator: String, right: Any, bool: Bool) =
this(left, operator, right, bool.value)
/**
* the Boolean
value of this Bool
.
*/
val value: Boolean = expression
private def getObjectsForFailureMessage =
left match {
case aEqualizer: org.scalactic.TripleEqualsSupport#Equalizer[_] =>
Prettifier.getObjectsForFailureMessage(aEqualizer.leftSide, right)
case aEqualizer: org.scalactic.TripleEqualsSupport#CheckingEqualizer[_] =>
Prettifier.getObjectsForFailureMessage(aEqualizer.leftSide, right)
case _ => Prettifier.getObjectsForFailureMessage(left, right)
}
/**
* raw message to report a failure, this method implementation will return the friendly raw message based on the passed
* in operator
.
*
* @return Localized friendly raw message based on the passed in operator
*/
def rawFailureMessage: String = {
operator match {
case "==" => Resources.rawDidNotEqual
case "===" => Resources.rawDidNotEqual
case "!=" => Resources.rawEqualed
case "!==" => Resources.rawEqualed
case ">" => Resources.rawWasNotGreaterThan
case ">=" => Resources.rawWasNotGreaterThanOrEqualTo
case "<" => Resources.rawWasNotLessThan
case "<=" => Resources.rawWasNotLessThanOrEqualTo
case "startsWith" => Resources.rawDidNotStartWith
case "endsWith" => Resources.rawDidNotEndWith
case "contains" =>
left match {
case leftMap: scala.collection.GenMap[_, _] => Resources.rawDidNotContainKey
case _ => Resources.rawDidNotContain
}
case "eq" => Resources.rawWasNotTheSameInstanceAs
case "ne" => Resources.rawWasTheSameInstanceAs
case "&&" | "&" =>
(left, right) match {
case (leftBool: Bool, rightBool: Bool) =>
if (leftBool.value)
Resources.rawCommaBut
else
leftBool.rawFailureMessage
case (leftBool: Bool, rightAny: Any) =>
if (leftBool.value)
Resources.rawCommaBut
else
leftBool.rawFailureMessage
case _ =>
Resources.rawCommaBut
}
case "||" | "|" => Resources.rawCommaAnd
case _ => Resources.rawExpressionWasFalse
}
}
/**
* raw message with a meaning opposite to that of the failure message, this method implementation will return the
* friendly raw message based on the passed in operator
.
*
* @return Localized negated friendly raw message based on the passed in operator
*/
def rawNegatedFailureMessage: String =
operator match {
case "==" => Resources.rawEqualed
case "===" => Resources.rawEqualed
case "!=" => Resources.rawDidNotEqual
case "!==" => Resources.rawDidNotEqual
case ">" => Resources.rawWasGreaterThan
case ">=" => Resources.rawWasGreaterThanOrEqualTo
case "<" => Resources.rawWasLessThan
case "<=" => Resources.rawWasLessThanOrEqualTo
case "startsWith" => Resources.rawStartedWith
case "endsWith" => Resources.rawEndedWith
case "contains" =>
left match {
case leftMap: scala.collection.GenMap[_, _] => Resources.rawContainedKey
case _ => Resources.rawContained
}
case "eq" => Resources.rawWasTheSameInstanceAs
case "ne" => Resources.rawWasNotTheSameInstanceAs
case "&&" | "&" => Resources.rawCommaAnd
case "||" | "|" => Resources.rawCommaAnd
case _ => Resources.rawExpressionWasTrue
}
/**
* raw mid sentence message to report a failure
*
* @return the same result as rawFailureMessage
*/
def rawMidSentenceFailureMessage: String = rawFailureMessage
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*
* @return the same result as rawNegatedFailureMessage
*/
def rawMidSentenceNegatedFailureMessage: String = rawNegatedFailureMessage
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
. Based
* on the passed in operator, this implementation will return the arguments needed by rawFailureMessage
* to construct the final friendly failure message.
*
* @return Vector that contains arguments needed by rawFailureMessage
to construct the final friendly failure message
*/
def failureMessageArgs: IndexedSeq[Any] =
operator match {
case "==" | "===" | "!=" | "!==" | ">" | ">=" | "<" | "<=" =>
val (leftee, rightee) = getObjectsForFailureMessage
Vector(leftee, rightee)
case "startsWith" | "endsWith" | "contains" | "eq" | "ne" =>
Vector(left, right)
case "&&" | "&" =>
(left, right) match {
case (leftBool: Bool, rightBool: Bool) =>
if (leftBool.value)
Vector(UnquotedString(leftBool.negatedFailureMessage), UnquotedString(rightBool.midSentenceFailureMessage))
else
leftBool.failureMessageArgs
case (leftBool: Bool, rightAny: Any) =>
if (leftBool.value)
Vector(UnquotedString(leftBool.negatedFailureMessage), rightAny)
else
leftBool.failureMessageArgs
case (leftAny: Any, rightBool: Bool) =>
Vector(leftAny, UnquotedString(if (rightBool.value) rightBool.midSentenceNegatedFailureMessage else rightBool.midSentenceFailureMessage))
case _ =>
Vector(left, right)
}
case "||" | "|" =>
(left, right) match {
case (leftBool: Bool, rightBool: Bool) =>
Vector(UnquotedString(leftBool.failureMessage), UnquotedString(rightBool.midSentenceFailureMessage))
case (leftBool: Bool, rightAny: Any) =>
Vector(UnquotedString(leftBool.failureMessage), rightAny)
case (leftAny: Any, rightBool: Bool) =>
Vector(leftAny, UnquotedString(rightBool.midSentenceFailureMessage))
case _ =>
Vector(left, right)
}
case _ => Vector.empty
}
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
. Based
* on the passed in operator, this implementation will return the arguments needed by rawNegatedFailureMessage
to construct
* the final negated friendly failure message.
*
* @return Vector that contains arguments needed by rawNegatedFailureMessage
to construct the final negated friendly failure message
*/
def negatedFailureMessageArgs: IndexedSeq[Any] =
operator match {
case "==" | "===" | "!=" | "!==" | ">" | ">=" | "<" | "<=" =>
val (leftee, rightee) = getObjectsForFailureMessage
Vector(leftee, rightee)
case "startsWith" | "endsWith" | "contains" | "eq" | "ne" =>
Vector(left, right)
case "&&" | "&" =>
(left, right) match {
case (leftBool: Bool, rightBool: Bool) =>
Vector(
UnquotedString(if (leftBool.value) leftBool.negatedFailureMessage else leftBool.failureMessage),
UnquotedString(if (rightBool.value) rightBool.midSentenceNegatedFailureMessage else rightBool.midSentenceFailureMessage)
)
case (leftBool: Bool, rightAny: Any) =>
Vector(UnquotedString(if (leftBool.value) leftBool.negatedFailureMessage else leftBool.failureMessage), rightAny)
case (leftAny: Any, rightBool: Bool) =>
Vector(leftAny, UnquotedString(if (rightBool.value) rightBool.midSentenceNegatedFailureMessage else rightBool.negatedFailureMessage))
case _ =>
Vector(left, right)
}
case "||" | "|" =>
(left, right) match {
case (leftBool: Bool, rightBool: Bool) =>
Vector(
UnquotedString(if (leftBool.value) leftBool.negatedFailureMessage else leftBool.failureMessage),
UnquotedString(if (rightBool.value) rightBool.midSentenceNegatedFailureMessage else rightBool.midSentenceFailureMessage)
)
case (leftBool: Bool, rightAny: Any) =>
Vector(UnquotedString(if (leftBool.value) leftBool.negatedFailureMessage else leftBool.failureMessage), rightAny)
case (leftAny: Any, rightBool: Bool) =>
Vector(leftAny, UnquotedString(if (rightBool.value) rightBool.midSentenceNegatedFailureMessage else rightBool.midSentenceFailureMessage))
case _ =>
Vector(left, right)
}
case _ => Vector.empty
}
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*
* @return the same result as failureMessageArgs
*/
def midSentenceFailureMessageArgs: IndexedSeq[Any] = failureMessageArgs
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*
* @return the same result as negatedFailureMessageArgs
*/
def midSentenceNegatedFailureMessageArgs: IndexedSeq[Any] = negatedFailureMessageArgs
}
/**
* Unary macro Bool
that is used by BooleanMacro
to wrap a recognized Boolean
expression represents a unary method call, which includes
* Boolean
expression that uses isEmpty
.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param expression the Boolean
expression
*/
private[scalactic] class UnaryMacroBool(left: Any, operator: String, expression: Boolean) extends Bool {
/**
* the Boolean
value of this Bool
.
*/
val value: Boolean = expression
/**
* raw message to report a failure, this method implementation will return the friendly raw message based on the passed
* in operator
.
*
* @return Localized friendly raw message based on the passed in operator
*/
def rawFailureMessage: String = {
operator match {
case "isEmpty" => Resources.rawWasNotEmpty
case "nonEmpty" => Resources.rawWasEmpty
case _ => Resources.rawExpressionWasFalse
}
}
/**
* raw message with a meaning opposite to that of the failure message, this method implementation will return the
* friendly raw message based on the passed in operator
.
*
* @return Localized negated friendly raw message based on the passed in operator
*/
def rawNegatedFailureMessage: String =
operator match {
case "isEmpty" => Resources.rawWasEmpty
case "nonEmpty" => Resources.rawWasNotEmpty
case _ => Resources.rawExpressionWasTrue
}
/**
* raw mid sentence message to report a failure
*
* @return the same result as rawFailureMessage
*/
def rawMidSentenceFailureMessage: String = rawFailureMessage
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*
* @return the same result as rawNegatedFailureMessage
*/
def rawMidSentenceNegatedFailureMessage: String = rawNegatedFailureMessage
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
. Based
* on the passed in operator, this implementation will return the arguments needed by rawFailureMessage
* to construct the final friendly failure message.
*
* @return Vector that contains arguments needed by rawFailureMessage
to construct the final friendly failure message
*/
def failureMessageArgs: IndexedSeq[Any] =
operator match {
case "isEmpty" | "nonEmpty" =>
Vector(left)
case _ => Vector.empty
}
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
. Based
* on the passed in operator, this implementation will return the arguments needed by rawNegatedFailureMessage
to construct
* the final negated friendly failure message.
*
* @return Vector that contains arguments needed by rawNegatedFailureMessage
to construct the final negated friendly failure message
*/
def negatedFailureMessageArgs: IndexedSeq[Any] =
operator match {
case "isEmpty" | "nonEmpty" =>
Vector(left)
case _ => Vector.empty
}
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*
* @return the same result as failureMessageArgs
*/
def midSentenceFailureMessageArgs: IndexedSeq[Any] = failureMessageArgs
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*
* @return the same result as negatedFailureMessageArgs
*/
def midSentenceNegatedFailureMessageArgs: IndexedSeq[Any] = negatedFailureMessageArgs
}
/**
* Macro Bool
that is used by BooleanMacro
to wrap a recognized Boolean
expression
* that represents a isInstanceOf
method call.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param className the class name passed to isInstanceOf
method call
* @param expression the Boolean
expression
*/
private[scalactic] class IsInstanceOfMacroBool(left: Any, operator: String, className: String, expression: Boolean) extends Bool {
/**
* the Boolean
value of this Bool
.
*/
val value: Boolean = expression
/**
* raw message to report a failure, this method implementation will return the friendly raw message based on the passed
* in operator
.
*
* @return Localized friendly raw message based on the passed in operator
*/
def rawFailureMessage: String = {
operator match {
case "isInstanceOf" => Resources.rawWasNotInstanceOf
case _ => Resources.rawExpressionWasFalse
}
}
/**
* raw message with a meaning opposite to that of the failure message, this method implementation will return the
* friendly raw message based on the passed in operator
.
*
* @return Localized negated friendly raw message based on the passed in operator
*/
def rawNegatedFailureMessage: String =
operator match {
case "isInstanceOf" => Resources.rawWasInstanceOf
case _ => Resources.rawExpressionWasTrue
}
/**
* raw mid sentence message to report a failure
*
* @return the same result as rawFailureMessage
*/
def rawMidSentenceFailureMessage: String = rawFailureMessage
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*
* @return the same result as rawNegatedFailureMessage
*/
def rawMidSentenceNegatedFailureMessage: String = rawNegatedFailureMessage
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
. Based
* on the passed in operator, this implementation will return the arguments needed by rawFailureMessage
* to construct the final friendly failure message.
*
* @return Vector that contains arguments needed by rawFailureMessage
to construct the final friendly failure message
*/
def failureMessageArgs: IndexedSeq[Any] =
operator match {
case "isInstanceOf" =>
Vector(left, UnquotedString(className))
case _ => Vector.empty
}
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
. Based
* on the passed in operator, this implementation will return the arguments needed by rawNegatedFailureMessage
to construct
* the final negated friendly failure message.
*
* @return Vector that contains arguments needed by rawNegatedFailureMessage
to construct the final negated friendly failure message
*/
def negatedFailureMessageArgs: IndexedSeq[Any] =
operator match {
case "isInstanceOf" =>
Vector(left, UnquotedString(className))
case _ => Vector.empty
}
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*
* @return the same result as failureMessageArgs
*/
def midSentenceFailureMessageArgs: IndexedSeq[Any] = failureMessageArgs
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*
* @return the same result as negatedFailureMessageArgs
*/
def midSentenceNegatedFailureMessageArgs: IndexedSeq[Any] = negatedFailureMessageArgs
}
/**
* Macro Bool
that is used by BooleanMacro
to wrap a recognized Boolean
expression
* that represents a length
or size
method call.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param operator the operator (method name) of the Boolean
expression
* @param actual the actual length or size of left
* @param expected the expected length or size of left
*/
private[scalactic] class LengthSizeMacroBool(left: Any, operator: String, actual: Any, expected: Any) extends Bool {
/**
* the Boolean
value of this Bool
.
*/
val value: Boolean = actual == expected
/**
* raw message to report a failure, this method implementation will return the friendly raw message based on the passed
* in operator
.
*
* @return Localized friendly raw message based on the passed in operator
*/
def rawFailureMessage: String = {
operator match {
case "length" => Resources.rawHadLengthInsteadOfExpectedLength
case "size" => Resources.rawHadSizeInsteadOfExpectedSize
case _ => Resources.rawExpressionWasFalse
}
}
/**
* raw message with a meaning opposite to that of the failure message, this method implementation will return the
* friendly raw message based on the passed in operator
.
*
* @return Localized negated friendly raw message based on the passed in operator
*/
def rawNegatedFailureMessage: String =
operator match {
case "length" => Resources.rawHadLength
case "size" => Resources.rawHadSize
case _ => Resources.rawExpressionWasTrue
}
/**
* raw mid sentence message to report a failure
*
* @return the same result as rawFailureMessage
*/
def rawMidSentenceFailureMessage: String = rawFailureMessage
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*
* @return the same result as rawNegatedFailureMessage
*/
def rawMidSentenceNegatedFailureMessage: String = rawNegatedFailureMessage
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
. Based
* on the passed in operator, this implementation will return the arguments needed by rawFailureMessage
* to construct the final friendly failure message.
*
* @return Vector that contains arguments needed by rawFailureMessage
to construct the final friendly failure message
*/
def failureMessageArgs: IndexedSeq[Any] =
operator match {
case "length" | "size" =>
Vector(left, actual, expected)
case _ => Vector.empty
}
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
. Based
* on the passed in operator, this implementation will return the arguments needed by rawNegatedFailureMessage
to construct
* the final negated friendly failure message.
*
* @return Vector that contains arguments needed by rawNegatedFailureMessage
to construct the final negated friendly failure message
*/
def negatedFailureMessageArgs: IndexedSeq[Any] =
operator match {
case "length" | "size" =>
Vector(left, actual)
case _ => Vector.empty
}
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*
* @return the same result as failureMessageArgs
*/
def midSentenceFailureMessageArgs: IndexedSeq[Any] = failureMessageArgs
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*
* @return the same result as negatedFailureMessageArgs
*/
def midSentenceNegatedFailureMessageArgs: IndexedSeq[Any] = negatedFailureMessageArgs
}
/**
* Macro Bool
that is used by BooleanMacro
to wrap a recognized Boolean
expression
* that represents a length
or size
method call.
*
* @param left the left-hand-side (LHS) of the Boolean
expression
* @param right the right-hand-side (RHS) of the Boolean
expression
* @param expression the Boolean
expression
*/
private[scalactic] class ExistsMacroBool(left: Any, right: Any, expression: Boolean) extends Bool {
/**
* the Boolean
value of this Bool
.
*/
val value: Boolean = expression
/**
* raw message to report a failure, this method implementation will return localized "x did not contain y" message.
*
* @return Localized friendly raw message based on the passed in operator
*/
def rawFailureMessage: String =
Resources.rawDidNotContain
/**
* raw message with a meaning opposite to that of the failure message, this method implementation will return localized
* "x contained y" message.
*
* @return Localized negated friendly raw message based on the passed in operator
*/
def rawNegatedFailureMessage: String =
Resources.rawContained
/**
* raw mid sentence message to report a failure
*
* @return the same result as rawFailureMessage
*/
def rawMidSentenceFailureMessage: String = rawFailureMessage
/**
* raw mid sentence message with a meaning opposite to that of the failure message
*
* @return the same result as rawNegatedFailureMessage
*/
def rawMidSentenceNegatedFailureMessage: String = rawNegatedFailureMessage
/**
* Arguments to construct final failure message with raw message returned from rawFailureMessage
. This
* implementation will return a Vector
that contains the passed in left
and right
.
*
* @return Vector that contains arguments needed by rawFailureMessage
to construct the final friendly failure message
*/
def failureMessageArgs: IndexedSeq[Any] =
Vector(left, right)
/**
* Arguments to construct final negated failure message with raw message returned from rawNegatedFailureMessage
.
* This implementation will return a Vector
that contains the passed in left
and right
.
*
* @return Vector that contains arguments needed by rawNegatedFailureMessage
to construct the final negated friendly failure message
*/
def negatedFailureMessageArgs: IndexedSeq[Any] =
Vector(left, right)
/**
* Arguments to construct final mid sentence failure message with raw message returned from rawMidSentenceFailureMessage
.
*
* @return the same result as failureMessageArgs
*/
def midSentenceFailureMessageArgs: IndexedSeq[Any] = failureMessageArgs
/**
* Arguments to construct final negated mid sentence failure message with raw message returned from rawMidSentenceNegatedFailureMessage
.
*
* @return the same result as negatedFailureMessageArgs
*/
def midSentenceNegatedFailureMessageArgs: IndexedSeq[Any] = negatedFailureMessageArgs
}
© 2015 - 2025 Weber Informatics LLC | Privacy Policy