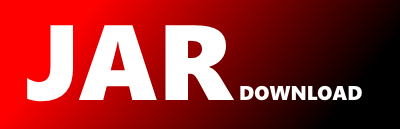
org.scalactic.anyvals.PosDouble.scala Maven / Gradle / Ivy
/*
* Copyright 2001-2014 Artima, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.scalactic.anyvals
import scala.language.implicitConversions
import scala.collection.immutable.NumericRange
/**
* An AnyVal
for positive Double
s.
*
* Note: a PosDouble
may not equal 0. If you want positive
* number or 0, use [[PosZDouble]].
*
*
* Because PosDouble
is an AnyVal
it
* will usually be as efficient as an Double
, being
* boxed only when a Double
would have been boxed.
*
*
*
* The PosDouble.apply
factory method is
* implemented in terms of a macro that checks literals for
* validity at compile time. Calling
* PosDouble.apply
with a literal
* Double
value will either produce a valid
* PosDouble
instance at run time or an error at
* compile time. Here's an example:
*
*
*
* scala> import anyvals._
* import anyvals._
*
* scala> PosDouble(1.0)
* res1: org.scalactic.anyvals.PosDouble = PosDouble(1.0)
*
* scala> PosDouble(0.0)
* <console>:14: error: PosDouble.apply can only be invoked on a positive (i > 0.0) floating point literal, like PosDouble(42.0).
* PosDouble(0.0)
* ^
*
*
*
* PosDouble.apply
cannot be used if the value
* being passed is a variable (i.e., not a literal),
* because the macro cannot determine the validity of variables
* at compile time (just literals). If you try to pass a
* variable to PosDouble.apply
, you'll get a
* compiler error that suggests you use a different factor
* method, PosDouble.from
, instead:
*
*
*
* scala> val x = 1.0
* x: Double = 1.0
*
* scala> PosDouble(x)
* <console>:15: error: PosDouble.apply can only be invoked on a floating point literal, like PosDouble(42.0). Please use PosDouble.from instead.
* PosDouble(x)
* ^
*
*
*
* The PosDouble.from
factory method will inspect
* the value at runtime and return an
* Option[PosDouble]
. If the value is valid,
* PosDouble.from
will return a
* Some[PosDouble]
, else it will return a
* None
. Here's an example:
*
*
*
* scala> PosDouble.from(x)
* res4: Option[org.scalactic.anyvals.PosDouble] = Some(PosDouble(1.0))
*
* scala> val y = 0.0
* y: Double = 0.0
*
* scala> PosDouble.from(y)
* res5: Option[org.scalactic.anyvals.PosDouble] = None
*
*
*
* The PosDouble.apply
factory method is marked
* implicit, so that you can pass literal Double
s
* into methods that require PosDouble
, and get the
* same compile-time checking you get when calling
* PosDouble.apply
explicitly. Here's an example:
*
*
*
* scala> def invert(pos: PosDouble): Double = Double.MaxValue - pos
* invert: (pos: org.scalactic.anyvals.PosDouble)Double
*
* scala> invert(1.1)
* res6: Double = 1.7976931348623157E308
*
* scala> invert(Double.MaxValue)
* res8: Double = 0.0
*
* scala> invert(0.0)
* <console>:15: error: PosDouble.apply can only be invoked on a positive (i > 0.0) floating point literal, like PosDouble(42.0).
* invert(0.0)
* ^
*
* scala> invert(-1.0)
* <console>:15: error: PosDouble.apply can only be invoked on a positive (i > 0.0) floating point literal, like PosDouble(42.0).
* invert(-1.0)
* ^
*
*
*
*
* This example also demonstrates that the
* PosDouble
companion object also defines implicit
* widening conversions when a similar conversion is provided in
* Scala. This makes it convenient to use a
* PosDouble
where a Double
is
* needed. An example is the subtraction in the body of the
* invert
method defined above,
* Double.MaxValue - pos
. Although
* Double.MaxValue
is a Double
, which
* has no -
method that takes a
* PosDouble
(the type of pos
), you
* can still subtract pos
, because the
* PosDouble
will be implicitly widened to
* Double
.
*
*
* @param value The Double
value underlying this PosDouble
.
*/
final class PosDouble private (val value: Double) extends AnyVal {
/**
* A string representation of this PosDouble
.
*/
override def toString: String = s"PosDouble($value)"
/**
* Converts this PosDouble
to a Byte
.
*/
def toByte: Byte = value.toByte
/**
* Converts this PosDouble
to a Short
.
*/
def toShort: Short = value.toShort
/**
* Converts this PosDouble
to a Char
.
*/
def toChar: Char = value.toChar
/**
* Converts this PosDouble
to an Int
.
*/
def toInt: Int = value.toInt
/**
* Converts this PosDouble
to a Long
.
*/
def toLong: Long = value.toLong
/**
* Converts this PosDouble
to a Float
.
*/
def toFloat: Float = value.toFloat
/**
* Converts this PosDouble
to a Double
.
*/
def toDouble: Double = value.toDouble
/** Returns this value, unmodified. */
def unary_+ : PosDouble = this
/** Returns the negation of this value. */
def unary_- : Double = -value
/**
* Converts this PosDouble
's value to a string then concatenates the given string.
*/
def +(x: String): String = value + x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Byte): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Short): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Char): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Int): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Long): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Float): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Double): Boolean = value < x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Byte): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Short): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Char): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Int): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Long): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Float): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Double): Boolean = value <= x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Byte): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Short): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Char): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Int): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Long): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Float): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Double): Boolean = value > x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Byte): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Short): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Char): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Int): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Long): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Float): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Double): Boolean = value >= x
/** Returns the sum of this value and `x`. */
def +(x: Byte): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Short): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Char): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Int): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Long): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Float): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Double): Double = value + x
/** Returns the difference of this value and `x`. */
def -(x: Byte): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Short): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Char): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Int): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Long): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Float): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Double): Double = value - x
/** Returns the product of this value and `x`. */
def *(x: Byte): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Short): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Char): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Int): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Long): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Float): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Double): Double = value * x
/** Returns the quotient of this value and `x`. */
def /(x: Byte): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Short): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Char): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Int): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Long): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Float): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Double): Double = value / x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Byte): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Short): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Char): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Int): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Long): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Float): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Double): Double = value % x
// Stuff from RichDouble
def isPosInfinity: Boolean = Double.PositiveInfinity == value
/**
* Returns this
if this > that
or that
otherwise.
*/
def max(that: PosDouble): PosDouble = if (math.max(value, that.value) == value) this else that
/**
* Returns this
if this < that
or that
otherwise.
*/
def min(that: PosDouble): PosDouble = if (math.min(value, that.value) == value) this else that
def isWhole = {
val longValue = value.toLong
longValue.toDouble == value || longValue == Long.MaxValue && value < Double.PositiveInfinity || longValue == Long.MinValue && value > Double.NegativeInfinity
}
def round: PosZLong = PosZLong.from(math.round(value)).get // Also could be zero.
def ceil: PosDouble = PosDouble.from(math.ceil(value)).get // I think this one is safe, but try NaN
def floor: PosZDouble = PosZDouble.from(math.floor(value)).get // Could be zero.
/** Converts an angle measured in degrees to an approximately equivalent
* angle measured in radians.
*
* @return the measurement of the angle x in radians.
*/
def toRadians: Double = math.toRadians(value)
/** Converts an angle measured in radians to an approximately equivalent
* angle measured in degrees.
* @return the measurement of the angle x in degrees.
*/
def toDegrees: Double = math.toDegrees(value)
// adapted from RichInt:
/**
* Create a Range
from this PosDouble
value
* until the specified end
(exclusive) with step value 1.
*
* @param end The final bound of the range to make.
* @return A [[scala.collection.immutable.Range.Partial[Double, NumericRange[Double]]]] from `this` up to but
* not including `end`.
*/
def until(end: Double): Range.Partial[Double, NumericRange[Double]] =
value.until(end)
/**
* Create a Range
from this PosDouble
value
* until the specified end
(exclusive) with the specified step
value.
*
* @param end The final bound of the range to make.
* @param end The final bound of the range to make.
* @param step The number to increase by for each step of the range.
* @return A [[scala.collection.immutable.NumericRange.Exclusive[Double]]] from `this` up to but
* not including `end`.
*/
def until(end: Double, step: Double): NumericRange.Exclusive[Double] =
value.until(end, step)
/**
* Create an inclusive Range
from this PosDouble
value
* to the specified end
with step value 1.
*
* @param end The final bound of the range to make.
* @return A [[scala.collection.immutable.Range.Partial[Double, NumericRange[Double]]]] from `'''this'''` up to
* and including `end`.
*/
def to(end: Double): Range.Partial[Double, NumericRange[Double]] =
value.to(end)
/**
* Create an inclusive Range
from this PosDouble
value
* to the specified end
with the specified step
value.
*
* @param end The final bound of the range to make.
* @param step The number to increase by for each step of the range.
* @return A [[scala.collection.immutable.NumericRange.Inclusive[Double]]] from `'''this'''` up to
* and including `end`.
*/
def to(end: Double, step: Double): NumericRange.Inclusive[Double] =
value.to(end, step)
}
/**
* The companion object for PosDouble
that offers
* factory methods that produce PosDouble
s,
* implicit widening conversions from PosDouble
to
* other numeric types, and maximum and minimum constant values
* for PosDouble
.
*/
object PosDouble {
/**
* The largest value representable as a positive Double
,
* which is PosDouble(1.7976931348623157E308)
.
*/
final val MaxValue: PosDouble = PosDouble.from(Double.MaxValue).get
/**
* The smallest value representable as a positive
* Double
, which is PosDouble(4.9E-324)
.
*/
final val MinValue: PosDouble = PosDouble.from(Double.MinPositiveValue).get // Can't use the macro here
/**
* A factory method that produces an Option[PosDouble]
given a
* Double
value.
*
*
* This method will inspect the passed Double
value and if
* it is a positive Double
, i.e., a value greater
* than 0.0, it will return a PosDouble
representing that value,
* wrapped in a Some
. Otherwise, the passed Double
* value is 0.0 or negative, so this method will return None
.
*
*
*
* This factory method differs from the apply
* factory method in that apply
is implemented
* via a macro that inspects Double
literals at
* compile time, whereas from
inspects
* Double
values at run time.
*
*
* @param value the Double
to inspect, and if positive, return
* wrapped in a Some[PosDouble]
.
* @return the specified Double
value wrapped in a
* Some[PosDouble]
, if it is positive, else
* None
.
*/
def from(value: Double): Option[PosDouble] =
if (value > 0.0) Some(new PosDouble(value)) else None
import language.experimental.macros
import scala.language.implicitConversions
/**
* A factory method, implemented via a macro, that produces a
* PosDouble
if passed a valid Double
* literal, otherwise a compile time error.
*
*
* The macro that implements this method will inspect the
* specified Double
expression at compile time. If
* the expression is a positive Double
literal,
* i.e., with a value greater than 0.0, it will return
* a PosDouble
representing that value. Otherwise,
* the passed Double
expression is either a literal
* that is 0.0 or negative, or is not a literal, so this method
* will give a compiler error.
*
*
*
* This factory method differs from the from
* factory method in that this method is implemented via a
* macro that inspects Double
literals at compile
* time, whereas from
inspects Double
* values at run time.
*
*
* @param value the Double
literal expression to
* inspect at compile time, and if positive, to return
* wrapped in a PosDouble
at run time.
* @return the specified, valid Double
literal
* value wrapped in a PosDouble
. (If the
* specified expression is not a valid Double
* literal, the invocation of this method will not
* compile.)
*/
implicit def apply(value: Double): PosDouble = macro PosDoubleMacro.apply
/**
* Implicit widening conversion from PosDouble
to
* Double
.
*
* @param pos the PosDouble
to widen
* @return the Double
value underlying the specified
* PosDouble
*/
implicit def widenToDouble(pos: PosDouble): Double = pos.value
/**
* Implicit widening conversion from PosDouble
to
* PosZDouble
.
*
* @param pos the PosDouble
to widen
* @return the Double
value underlying the specified
* PosDouble
wrapped in a PosZDouble
.
*/
implicit def widenToPosZDouble(pos: PosDouble): PosZDouble = PosZDouble.from(pos.value).get
/**
* Implicit Ordering instance.
*/
implicit val posDoubleOrd: Ordering[PosDouble] =
new Ordering[PosDouble] {
def compare(x: PosDouble, y: PosDouble): Int = x.toDouble.compare(y)
}
}