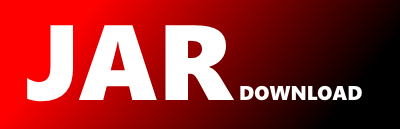
scalafx.scene.AccessibleRole.scala Maven / Gradle / Ivy
The newest version!
/*
* Copyright (c) 2011-2014, ScalaFX Project
* All rights reserved.
*
* Redistribution and use in source and binary forms, with or without
* modification, are permitted provided that the following conditions are met:
* * Redistributions of source code must retain the above copyright
* notice, this list of conditions and the following disclaimer.
* * Redistributions in binary form must reproduce the above copyright
* notice, this list of conditions and the following disclaimer in the
* documentation and/or other materials provided with the distribution.
* * Neither the name of the ScalaFX Project nor the
* names of its contributors may be used to endorse or promote products
* derived from this software without specific prior written permission.
*
* THIS SOFTWARE IS PROVIDED BY THE COPYRIGHT HOLDERS AND CONTRIBUTORS "AS IS" AND
* ANY EXPRESS OR IMPLIED WARRANTIES, INCLUDING, BUT NOT LIMITED TO, THE IMPLIED
* WARRANTIES OF MERCHANTABILITY AND FITNESS FOR A PARTICULAR PURPOSE ARE
* DISCLAIMED. IN NO EVENT SHALL THE SCALAFX PROJECT OR ITS CONTRIBUTORS BE LIABLE
* FOR ANY DIRECT, INDIRECT, INCIDENTAL, SPECIAL, EXEMPLARY, OR CONSEQUENTIAL
* DAMAGES (INCLUDING, BUT NOT LIMITED TO, PROCUREMENT OF SUBSTITUTE GOODS OR
* SERVICES; LOSS OF USE, DATA, OR PROFITS; OR BUSINESS INTERRUPTION) HOWEVER CAUSED
* AND ON ANY THEORY OF LIABILITY, WHETHER IN CONTRACT, STRICT LIABILITY, OR TORT
* (INCLUDING NEGLIGENCE OR OTHERWISE) ARISING IN ANY WAY OUT OF THE USE OF THIS
* SOFTWARE, EVEN IF ADVISED OF THE POSSIBILITY OF SUCH DAMAGE.
*/
package scalafx.scene
import javafx.{scene => jfxs}
import scalafx.delegate.{SFXEnumDelegate, SFXEnumDelegateCompanion}
/**
* This enum describes the accessible role for a `Node`.
*
* The role is used by assistive technologies such as screen readers
* to decide the set of actions and attributes for a node. For example,
* when the screen reader needs the current value of a slider, it
* will request it using the value attribute. When the screen reader
* changes the value of the slider, it will use an action to set
* the current value of the slider. The slider must respond
* appropriately to both these requests.
*
* Wraps [[http://docs.oracle.com/javase/8/javafx/api/javafx/scene/AccessibleRole.html]]
*/
object AccessibleRole extends SFXEnumDelegateCompanion[jfxs.AccessibleRole, AccessibleRole] {
/**
* Button role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val Button = new AccessibleRole(jfxs.AccessibleRole.BUTTON)
/**
* Check Box role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#SELECTED}
* - {@link AccessibleAttribute#INDETERMINATE}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val CheckBox = new AccessibleRole(jfxs.AccessibleRole.CHECK_BOX)
/**
* Check Menu Item role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#ACCELERATOR}
* - {@link AccessibleAttribute#MNEMONIC}
* - {@link AccessibleAttribute#DISABLED}
* - {@link AccessibleAttribute#SELECTED}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val CheckMenuItem = new AccessibleRole(jfxs.AccessibleRole.CHECK_MENU_ITEM)
/**
* Combo Box role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#EXPANDED}
* - {@link AccessibleAttribute#EDITABLE}
*
* Actions:
*
* - {@link AccessibleAction#EXPAND}
* - {@link AccessibleAction#COLLAPSE}
*
*/
val ComboBox = new AccessibleRole(jfxs.AccessibleRole.COMBO_BOX)
/**
* Context Menu role.
*
* Attributes:
*
* - {@link AccessibleAttribute#PARENT_MENU}
* - {@link AccessibleAttribute#VISIBLE}
*
* Actions:
*
*
*/
val ContextMenu = new AccessibleRole(jfxs.AccessibleRole.CONTEXT_MENU)
/**
* Date Picker role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#DATE}
*
* Actions:
*
*
*/
val DatePicker = new AccessibleRole(jfxs.AccessibleRole.DATE_PICKER)
/**
* Decrement Button role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val DecrementButton = new AccessibleRole(jfxs.AccessibleRole.DECREMENT_BUTTON)
/**
* Hyperlink role.
*
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#VISITED}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val Hyperlink = new AccessibleRole(jfxs.AccessibleRole.HYPERLINK)
/**
* Increment Button role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val IncrementButton = new AccessibleRole(jfxs.AccessibleRole.INCREMENT_BUTTON)
/**
* Image View role.
*
* Attributes:
*
*
* Actions:
*
*
*
* It is strongly recommended that a text description of the image be provided
* for each {@link ImageView}. This can be done by setting either
* {@link Node#accessibleTextProperty()} for the {@link ImageView}
* or by using {@link AccessibleAttribute#LABELED_BY}.
*
*/
val ImageView = new AccessibleRole(jfxs.AccessibleRole.IMAGE_VIEW)
/**
* List View role.
*
* Attributes:
*
* - {@link AccessibleAttribute#ITEM_AT_INDEX}
* - {@link AccessibleAttribute#ITEM_COUNT}
* - {@link AccessibleAttribute#SELECTED_ITEMS}
* - {@link AccessibleAttribute#MULTIPLE_SELECTION}
* - {@link AccessibleAttribute#VERTICAL_SCROLLBAR}
* - {@link AccessibleAttribute#HORIZONTAL_SCROLLBAR}
* - {@link AccessibleAttribute#FOCUS_ITEM}
*
* Actions:
*
* - {@link AccessibleAction#SHOW_ITEM}
* - {@link AccessibleAction#SET_SELECTED_ITEMS}
*
*/
val ListView = new AccessibleRole(jfxs.AccessibleRole.LIST_VIEW)
/**
* List Item role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#INDEX}
* - {@link AccessibleAttribute#SELECTED}
*
* Actions:
*
* - {@link AccessibleAction#REQUEST_FOCUS}
*
*/
val ListItem = new AccessibleRole(jfxs.AccessibleRole.LIST_ITEM)
/**
* Menu role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#ACCELERATOR}
* - {@link AccessibleAttribute#MNEMONIC}
* - {@link AccessibleAttribute#DISABLED}
* - {@link AccessibleAttribute#SUBMENU}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val Menu = new AccessibleRole(jfxs.AccessibleRole.MENU)
/**
* Menu Bar role.
*
* Attributes:
*
*
* Actions:
*
*
*/
val MenuBar = new AccessibleRole(jfxs.AccessibleRole.MENU_BAR)
/**
* Menu Button role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val MenuButton = new AccessibleRole(jfxs.AccessibleRole.MENU_BUTTON)
/**
* Menu Item role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#ACCELERATOR}
* - {@link AccessibleAttribute#MNEMONIC}
* - {@link AccessibleAttribute#DISABLED}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val MenuItem = new AccessibleRole(jfxs.AccessibleRole.MENU_ITEM)
/**
* Node role.
*
* Attributes:
*
* - {@link AccessibleAttribute#ROLE}
* - {@link AccessibleAttribute#PARENT}
* - {@link AccessibleAttribute#SCENE}
* - {@link AccessibleAttribute#BOUNDS}
* - {@link AccessibleAttribute#DISABLED}
* - {@link AccessibleAttribute#FOCUSED}
* - {@link AccessibleAttribute#VISIBLE}
*
* Actions:
*
* - {@link AccessibleAction#REQUEST_FOCUS}
*
* Optional Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#LABELED_BY}
* - {@link AccessibleAttribute#ROLE_DESCRIPTION}
* - {@link AccessibleAttribute#HELP}
*
* Optional Actions:
*
* - {@link AccessibleAction#SHOW_MENU}
*
*/
val Node = new AccessibleRole(jfxs.AccessibleRole.NODE)
/**
* Page role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#SELECTED}
*
* Actions:
*
* - {@link AccessibleAction#REQUEST_FOCUS}
*
*/
val PageItem = new AccessibleRole(jfxs.AccessibleRole.PAGE_ITEM)
/**
* Pagination role.
*
* Attributes:
*
* - {@link AccessibleAttribute#ITEM_AT_INDEX}
* - {@link AccessibleAttribute#ITEM_COUNT}
* - {@link AccessibleAttribute#FOCUS_ITEM}
*
* Actions:
*
*
*/
val Pagination = new AccessibleRole(jfxs.AccessibleRole.PAGINATION)
/**
* Parent role.
*
* Attributes:
*
* - {@link AccessibleAttribute#CHILDREN}
*
* Actions:
*
*
*/
val Parent = new AccessibleRole(jfxs.AccessibleRole.PARENT)
/**
* Password Field role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT} - must return null or empty string
*
* Actions:
*
*
*/
val PasswordField = new AccessibleRole(jfxs.AccessibleRole.PASSWORD_FIELD)
/**
* Progress Indicator role.
*
* Attributes:
*
* - {@link AccessibleAttribute#VALUE}
* - {@link AccessibleAttribute#MIN_VALUE}
* - {@link AccessibleAttribute#MAX_VALUE}
* - {@link AccessibleAttribute#INDETERMINATE}
*
* Actions:
*
*
*/
val ProgressIndicator = new AccessibleRole(jfxs.AccessibleRole.PROGRESS_INDICATOR)
/**
* Radio Button role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#SELECTED}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val RadioButton = new AccessibleRole(jfxs.AccessibleRole.RADIO_BUTTON)
/**
* Radio Menu Item role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#ACCELERATOR}
* - {@link AccessibleAttribute#MNEMONIC}
* - {@link AccessibleAttribute#DISABLED}
* - {@link AccessibleAttribute#SELECTED}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val RadioMenuItem = new AccessibleRole(jfxs.AccessibleRole.RADIO_MENU_ITEM)
/**
* Slider role.
*
* Attributes:
*
* - {@link AccessibleAttribute#VALUE}
* - {@link AccessibleAttribute#MIN_VALUE}
* - {@link AccessibleAttribute#MAX_VALUE}
* - {@link AccessibleAttribute#ORIENTATION}
*
* Actions:
*
* - {@link AccessibleAction#INCREMENT}
* - {@link AccessibleAction#DECREMENT}
* - {@link AccessibleAction#SET_VALUE}
*
*/
val Slider = new AccessibleRole(jfxs.AccessibleRole.SLIDER)
/**
* Spinner role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
*
* Actions:
*
* - {@link AccessibleAction#INCREMENT}
* - {@link AccessibleAction#DECREMENT}
*
*/
val Spinner = new AccessibleRole(jfxs.AccessibleRole.SPINNER)
/**
* Text role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#FONT}
*
* Actions:
*
* - {@link AccessibleAction#SET_TEXT}
*
*/
val Text = new AccessibleRole(jfxs.AccessibleRole.TEXT)
/**
* Text Area role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#FONT}
* - {@link AccessibleAttribute#EDITABLE}
* - {@link AccessibleAttribute#SELECTION_START}
* - {@link AccessibleAttribute#SELECTION_END}
* - {@link AccessibleAttribute#CARET_OFFSET}
* - {@link AccessibleAttribute#OFFSET_AT_POINT}
* - {@link AccessibleAttribute#LINE_START}
* - {@link AccessibleAttribute#LINE_END}
* - {@link AccessibleAttribute#LINE_FOR_OFFSET}
* - {@link AccessibleAttribute#BOUNDS_FOR_RANGE}
*
* Actions:
*
* - {@link AccessibleAction#SET_TEXT}
* - {@link AccessibleAction#SET_TEXT_SELECTION}
*
*/
val TextArea = new AccessibleRole(jfxs.AccessibleRole.TEXT_AREA)
/**
* Text Field role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#FONT}
* - {@link AccessibleAttribute#EDITABLE}
* - {@link AccessibleAttribute#SELECTION_START}
* - {@link AccessibleAttribute#SELECTION_END}
* - {@link AccessibleAttribute#CARET_OFFSET}
* - {@link AccessibleAttribute#OFFSET_AT_POINT}
* - {@link AccessibleAttribute#BOUNDS_FOR_RANGE}
*
* Actions:
*
* - {@link AccessibleAction#SET_TEXT}
* - {@link AccessibleAction#SET_TEXT_SELECTION}
*
*/
val TextField = new AccessibleRole(jfxs.AccessibleRole.TEXT_FIELD)
/**
* Toggle Button role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#SELECTED}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
*
*/
val ToggleButton = new AccessibleRole(jfxs.AccessibleRole.TOGGLE_BUTTON)
/**
* Tooltip role.
*
* Attributes:
*
*
* Actions:
*
*
*/
val Tooltip = new AccessibleRole(jfxs.AccessibleRole.TOOLTIP)
/**
* Scroll Bar role.
*
* Attributes:
*
* - {@link AccessibleAttribute#VALUE}
* - {@link AccessibleAttribute#MAX_VALUE}
* - {@link AccessibleAttribute#MIN_VALUE}
* - {@link AccessibleAttribute#ORIENTATION}
*
* Actions:
*
* - {@link AccessibleAction#INCREMENT}
* - {@link AccessibleAction#DECREMENT}
* - {@link AccessibleAction#BLOCK_INCREMENT}
* - {@link AccessibleAction#BLOCK_DECREMENT}
* - {@link AccessibleAction#SET_VALUE}
*
*/
val ScrollBar = new AccessibleRole(jfxs.AccessibleRole.SCROLL_BAR)
/**
* Scroll Pane role.
*
* Attributes:
*
* - {@link AccessibleAttribute#CONTENTS}
* - {@link AccessibleAttribute#HORIZONTAL_SCROLLBAR}
* - {@link AccessibleAttribute#VERTICAL_SCROLLBAR}
*
* Actions:
*
*
*/
val ScrollPane = new AccessibleRole(jfxs.AccessibleRole.SCROLL_PANE)
/**
* Split Menu Button role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#EXPANDED}
*
* Actions:
*
* - {@link AccessibleAction#FIRE}
* - {@link AccessibleAction#EXPAND}
* - {@link AccessibleAction#COLLAPSE}
*
*/
val SplitMenuButton = new AccessibleRole(jfxs.AccessibleRole.SPLIT_MENU_BUTTON)
/**
* Tab Item role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#SELECTED}
*
* Actions:
*
* - {@link AccessibleAction#REQUEST_FOCUS}
*
*/
val TabItem = new AccessibleRole(jfxs.AccessibleRole.TAB_ITEM)
/**
* Tab Pane role.
*
* Attributes:
*
* - {@link AccessibleAttribute#ITEM_AT_INDEX}
* - {@link AccessibleAttribute#ITEM_COUNT}
* - {@link AccessibleAttribute#FOCUS_ITEM}
*
* Actions:
*
*
*/
val TabPane = new AccessibleRole(jfxs.AccessibleRole.TAB_PANE)
/**
* Table Cell role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#ROW_INDEX}
* - {@link AccessibleAttribute#COLUMN_INDEX}
* - {@link AccessibleAttribute#SELECTED}
*
* Actions:
*
* - {@link AccessibleAction#REQUEST_FOCUS}
*
*/
val TableCell = new AccessibleRole(jfxs.AccessibleRole.TABLE_CELL)
/**
* Table Column role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#INDEX}
*
* Actions:
*
*
*/
val TableColumn = new AccessibleRole(jfxs.AccessibleRole.TABLE_COLUMN)
/**
* Table Row role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#INDEX}
*
* Actions:
*
*
*/
val TableRow = new AccessibleRole(jfxs.AccessibleRole.TABLE_ROW)
/**
* Table View role.
*
* Attributes:
*
* - {@link AccessibleAttribute#ROW_COUNT}
* - {@link AccessibleAttribute#ROW_AT_INDEX}
* - {@link AccessibleAttribute#COLUMN_COUNT}
* - {@link AccessibleAttribute#COLUMN_AT_INDEX}
* - {@link AccessibleAttribute#SELECTED_ITEMS}
* - {@link AccessibleAttribute#CELL_AT_ROW_COLUMN}
* - {@link AccessibleAttribute#HEADER}
* - {@link AccessibleAttribute#MULTIPLE_SELECTION}
* - {@link AccessibleAttribute#VERTICAL_SCROLLBAR}
* - {@link AccessibleAttribute#HORIZONTAL_SCROLLBAR}
* - {@link AccessibleAttribute#FOCUS_ITEM}
*
* Actions:
*
* - {@link AccessibleAction#SHOW_ITEM}
* - {@link AccessibleAction#SET_SELECTED_ITEMS}
*
*/
val TableView = new AccessibleRole(jfxs.AccessibleRole.TABLE_VIEW)
/**
* Thumb role.
*
* Attributes:
*
* - {@link AccessibleAttribute#VALUE}
*
* Actions:
*
*
*/
val Thumb = new AccessibleRole(jfxs.AccessibleRole.THUMB)
/**
* Titled Pane role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#EXPANDED}
*
* Actions:
*
* - {@link AccessibleAction#EXPAND}
* - {@link AccessibleAction#COLLAPSE}
*
*/
val TitledPane = new AccessibleRole(jfxs.AccessibleRole.TITLED_PANE)
/**
* Tool Bar role.
*
* Attributes:
*
* - {@link AccessibleAttribute#OVERFLOW_BUTTON}
*
* Actions:
*
*
*/
val ToolBar = new AccessibleRole(jfxs.AccessibleRole.TOOL_BAR)
/**
* Tree Item role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#INDEX}
* - {@link AccessibleAttribute#SELECTED}
* - {@link AccessibleAttribute#EXPANDED}
* - {@link AccessibleAttribute#LEAF}
* - {@link AccessibleAttribute#DISCLOSURE_LEVEL}
* - {@link AccessibleAttribute#TREE_ITEM_COUNT}
* - {@link AccessibleAttribute#TREE_ITEM_AT_INDEX}
* - {@link AccessibleAttribute#TREE_ITEM_PARENT}
*
* Actions:
*
* - {@link AccessibleAction#EXPAND}
* - {@link AccessibleAction#COLLAPSE}
* - {@link AccessibleAction#REQUEST_FOCUS}
*
*/
val TreeItem = new AccessibleRole(jfxs.AccessibleRole.TREE_ITEM)
/**
* Tree Table Cell role.
*
* Attributes:
*
* - {@link AccessibleAttribute#TEXT}
* - {@link AccessibleAttribute#SELECTED}
* - {@link AccessibleAttribute#ROW_INDEX}
* - {@link AccessibleAttribute#COLUMN_INDEX}
*
* Actions:
*
* - {@link AccessibleAction#REQUEST_FOCUS}
*
*/
val TreeTableCell = new AccessibleRole(jfxs.AccessibleRole.TREE_TABLE_CELL)
/**
* Tree Table Row role.
*
* Attributes:
*
* - {@link AccessibleAttribute#INDEX}
* - {@link AccessibleAttribute#EXPANDED}
* - {@link AccessibleAttribute#LEAF}
* - {@link AccessibleAttribute#DISCLOSURE_LEVEL}
* - {@link AccessibleAttribute#TREE_ITEM_COUNT}
* - {@link AccessibleAttribute#TREE_ITEM_AT_INDEX}
* - {@link AccessibleAttribute#TREE_ITEM_PARENT}
*
* Actions:
*
* - {@link AccessibleAction#EXPAND}
* - {@link AccessibleAction#COLLAPSE}
*
*/
val TreeTableRow = new AccessibleRole(jfxs.AccessibleRole.TREE_TABLE_ROW)
/**
* Tree Table View role.
*
* Attributes:
*
* - {@link AccessibleAttribute#ROW_COUNT}
* - {@link AccessibleAttribute#ROW_AT_INDEX}
* - {@link AccessibleAttribute#COLUMN_COUNT}
* - {@link AccessibleAttribute#COLUMN_AT_INDEX}
* - {@link AccessibleAttribute#SELECTED_ITEMS}
* - {@link AccessibleAttribute#CELL_AT_ROW_COLUMN}
* - {@link AccessibleAttribute#HEADER}
* - {@link AccessibleAttribute#MULTIPLE_SELECTION}
* - {@link AccessibleAttribute#VERTICAL_SCROLLBAR}
* - {@link AccessibleAttribute#HORIZONTAL_SCROLLBAR}
* - {@link AccessibleAttribute#FOCUS_ITEM}
*
* Actions:
*
* - {@link AccessibleAction#SHOW_ITEM}
* - {@link AccessibleAction#SET_SELECTED_ITEMS}
*
*/
val TreeTableView = new AccessibleRole(jfxs.AccessibleRole.TREE_TABLE_VIEW)
/**
* Tree View role.
*
* Attributes:
*
* - {@link AccessibleAttribute#ROW_COUNT}
* - {@link AccessibleAttribute#ROW_AT_INDEX}
* - {@link AccessibleAttribute#SELECTED_ITEMS}
* - {@link AccessibleAttribute#MULTIPLE_SELECTION}
* - {@link AccessibleAttribute#VERTICAL_SCROLLBAR}
* - {@link AccessibleAttribute#HORIZONTAL_SCROLLBAR}
* - {@link AccessibleAttribute#FOCUS_ITEM}
*
* Actions:
*
* - {@link AccessibleAction#SHOW_ITEM}
* - {@link AccessibleAction#SET_SELECTED_ITEMS}
*
*/
val TreeView = new AccessibleRole(jfxs.AccessibleRole.TREE_VIEW)
protected override def unsortedValues: Array[AccessibleRole] = Array(Button, CheckBox, CheckMenuItem, ComboBox,
ContextMenu, DatePicker, DecrementButton, Hyperlink, IncrementButton, ImageView, ListView, ListItem, Menu,
MenuBar, MenuButton, MenuItem, Node, PageItem, Pagination, Parent, PasswordField, ProgressIndicator, RadioButton,
RadioMenuItem, Slider, Spinner, Text, TextArea, TextField, ToggleButton, Tooltip, ScrollBar, ScrollPane,
SplitMenuButton, TabItem, TabPane, TableCell, TableColumn, TableRow, TableView, Thumb, TitledPane,
ToolBar, TreeItem, TreeTableCell, TreeTableRow, TreeTableView, TreeView
)
}
sealed case class AccessibleRole(override val delegate: jfxs.AccessibleRole) extends SFXEnumDelegate[jfxs.AccessibleRole]
© 2015 - 2024 Weber Informatics LLC | Privacy Policy