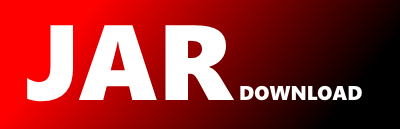
org.scalactic.NormalizingEquality.scala Maven / Gradle / Ivy
/*
* Copyright 2001-2013 Artima, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.scalactic
/**
* An Equality[A]
implementation that determines the equality of two objects by normalizing
* one or both objects, then comparing the results using an “after normalization” equality referenced from
* the afterNormalizationEquality
member. By default, the afterNormalizationEquality
is
* an instance of Equality.default[A]
.
*
*
*
* NormalizingEquality
is returned by the Explicitly
DSL's “after
being
”
* syntax, using for the afterNormalizationEquality
the implicit Equality
in scope for the type
* of Uniformity
passed to being
. Here's an example:
*
*
*
* scala> import org.scalactic._
* import org.scalactic._
*
* scala> import Explicitly._
* import Explicitly._
*
* scala> import StringNormalizations._
* import StringNormalizations._
*
* scala> after being lowerCased
* res0: org.scalactic.NormalizingEquality[String] = ComposedNormalizingEquality(Equality.default,lowerCased)
*
*/
trait NormalizingEquality[A] extends Equality[A] { thisNormEq =>
/**
* The Equality
with which to determine equality after normalizing the left-hand and, if appropriate,
* the right-hand values.
*
*
* In this trait's implementation, this val
is initialized with the result of invoking Equality.default[A]
.
* Thus default Equality
is the default afterNormalizationEquality
. This may be changed by overriding
* afterNormalizationEquality
in subclasses.
*
*/
val afterNormalizationEquality: Equality[A] = Equality.default[A]
/**
* Determines the equality of two objects by normalizing the left-hand value, a
, and, if appropriate, the right-hand
* value, b
, then passing them to areEqual
method of afterNormalizationEquality
.
*
*
* The left-hand value, a
, is normalized by passing it to the normalized
method of this
* NormalizingEquality
. The right-hand value, b
, is normalized, if appropriate, by passing it
* to the normalizedOrSame
method of this NormalizingEquality
.
*
*/
final def areEqual(a: A, b: Any): Boolean = {
afterNormalizationEquality.areEqual(normalized(a), normalizedOrSame(b))
}
/**
* Returns a normalized form of the passed object.
*
*
* If the passed object is already in normal form, this method may return the same instance passed.
*
*
* @tparam A the type of the object to normalize
* @param a the object to normalize
* @return the normalized form of the passed object
*/
def normalized(a: A): A
/**
* Indicates whether this NormalizingEquality
's normalized
method can “handle” the passed object, if cast to the
* appropriate type A
.
*
*
* If this method returns true for a particular passed object, it means that if the object is passed
* to normalizedOrSame
, that method will return the result of passing it to normalized
.
* It does not mean that the object will necessarily be modified when passed to normalizedOrSame
or normalized
.
* For more information and examples, see the documentation for normalizedCanHandle
in trait Uniformity
,
* which has the same contract.
*
*/
def normalizedCanHandle(b: Any): Boolean
/**
* Returns either the result of passing this object to normalized
, if appropriate, or the same object.
*
* @param b the object to normalize, if appropriate
* @return a normalized form of the passed object, if this Uniformity
was able to normalize it, else the same object passed
*/
def normalizedOrSame(b: Any): Any
/**
* Returns a new NormalizingEquality
that combines this and the passed Uniformity
.
*
*
* The normalized
and normalizedOrSame
methods
* of the NormalizingEquality
's returned by this method return a result
* obtained by forwarding the passed value first to this NormalizingEquality
's implementation of the method,
* then passing that result to the passed Uniformity
's implementation of the method, respectively.
* Essentially, the body of the composed normalized
method is:
*
*
*
* uniformityPassedToAnd.normalized(uniformityOnWhichAndWasInvoked.normalized(a))
*
*
*
* And the body of the composed normalizedOrSame
method is:
*
*
*
* uniformityPassedToAnd.normalizedOrSame(uniformityOnWhichAndWasInvoked.normalizedOrSame(a))
*
*
*
* The normalizeCanHandle
method of the NormalizingEquality
returned by this method returns a result
* obtained by anding the result of forwarding the passed value to this NormalizingEquality
's implementation of the method
* with the result of forwarding it to the passed Uniformity
's implementation.
* Essentially, the body of the composed normalizeCanHandle
method is:
*
*
*
* normEqOnWhichAndWasInvoked.normalizeCanHandle(a) && uniformityPassedToAnd.normalizeCanHandle(a)
*
*
* @param other a Uniformity
to 'and' with this one
* @return a NormalizingEquality
representing the composition of this and the passed Uniformity
*/
infix
final def and(other: Uniformity[A]): NormalizingEquality[A] =
new ComposedNormalizingEquality[A](afterNormalizationEquality, this.toUniformity and other)
// TODO: If someone passes a Normalization only to and, should I give them back a NormalizingEquivalence?
// If so, the same thing should be one if Equality.afterBeing gets just a Normalization.
/**
* Converts this NormalizingEquality
to a Uniformity
.
*
* @return a Uniformity
whose normalized
, normalizedCanHandle
, and normalizedOrSame
methods
* are implemented by the corresponding methods of this NormalizingEquality
.
*/
final def toUniformity: Uniformity[A] =
new Uniformity[A] {
def normalized(a: A): A = thisNormEq.normalized(a)
def normalizedCanHandle(b: Any): Boolean = thisNormEq.normalizedCanHandle(b)
def normalizedOrSame(b: Any): Any = thisNormEq.normalizedOrSame(b)
}
}