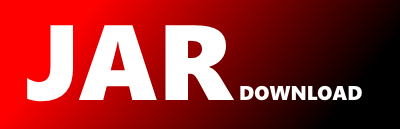
org.scalactic.TripleEquals.scala Maven / Gradle / Ivy
/*
* Copyright 2001-2013 Artima, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.scalactic
import TripleEqualsSupport._
/**
* Provides ===
and !==
operators that return Boolean
, delegate the equality determination
* to an Equality
type class, and require no relationship between the types of the two values compared.
*
*
* Recommended Usage:
* Trait TripleEquals
is useful (in both production and test code) when you need determine equality for a type of object differently than its
* equals
method: either you can't change the equals
method, or the equals
method is sensible generally, but
* you are in a special situation where you need something else. You can use the SuperSafe Community Edition compiler plugin to
* get a compile-time safety check of types being compared with ===
. In situations where you need a stricter type check, you can use
* TypeCheckedTripleEquals
.
*
*
*
* This trait will override or hide implicit methods defined by its sibling trait,
* TypeCheckedTripleEquals
,
* and can therefore be used to temporarily turn of type checking in a limited scope. Here's an example, in which TypeCheckedTripleEquals
will
* cause a compiler error:
*
*
*
* import org.scalactic._
* import TypeCheckedTripleEquals._
*
* object Example {
*
* def cmp(a: Int, b: Long): Int = {
* if (a === b) 0 // This line won't compile
* else if (a < b) -1
* else 1
* }
*
* def cmp(s: String, t: String): Int = {
* if (s === t) 0
* else if (s < t) -1
* else 1
* }
* }
*
*
* Because Int
and Long
are not in a subtype/supertype relationship, comparing 1
and 1L
in the context
* of TypeCheckedTripleEquals
will generate a compiler error:
*
*
*
* Example.scala:9: error: types Int and Long do not adhere to the equality constraint selected for
* the === and !== operators; they must either be in a subtype/supertype relationship;
* the missing implicit parameter is of type org.scalactic.Constraint[Int,Long]
* if (a === b) 0 // This line won't compile
* ^
* one error found
*
*
*
* You can “turn off” the type checking locally by importing the members of TripleEquals
in
* a limited scope:
*
*
*
* package org.scalactic.examples.tripleequals
*
* import org.scalactic._
* import TypeCheckedTripleEquals._
*
* object Example {
*
* def cmp(a: Int, b: Long): Int = {
* import TripleEquals._
* if (a === b) 0
* else if (a < b) -1
* else 1
* }
*
* def cmp(s: String, t: String): Int = {
* if (s === t) 0
* else if (s < t) -1
* else 1
* }
* }
*
*
*
* With the above change, the Example.scala
file compiles fine. Type checking is turned off only inside the first cmp
method that
* takes an Int
and a Long
. TypeCheckedTripleEquals
is still enforcing its type constraint, for example, for the s === t
* expression in the other overloaded cmp
method that takes strings.
*
*
*
* Because the methods in TripleEquals
(and its siblings)override all the methods defined in
* supertype TripleEqualsSupport
, you can achieve the same
* kind of nested tuning of equality constraints whether you mix in traits, import from companion objects, or use some combination of both.
*
*
*
* In short, you should be able to select a primary constraint level via either a mixin or import, then change that in nested scopes
* however you want, again either through a mixin or import, without getting any implicit conversion ambiguity. The innermost constraint level in scope
* will always be in force.
*
*
* @author Bill Venners
*/
trait TripleEquals extends TripleEqualsSupport {
import scala.language.implicitConversions
// Inherit the Scaladoc for these methods
implicit override def convertToEqualizer[T](left: T): Equalizer[T] = new Equalizer(left)
override def convertToCheckingEqualizer[T](left: T): CheckingEqualizer[T] = new CheckingEqualizer(left)
implicit override def unconstrainedEquality[A, B](implicit equalityOfA: Equality[A]): A CanEqual B = new EqualityConstraint[A, B](equalityOfA)
override def lowPriorityTypeCheckedConstraint[A, B](implicit equivalenceOfB: Equivalence[B], ev: A <:< B): A CanEqual B = new AToBEquivalenceConstraint[A, B](equivalenceOfB, ev)
override def convertEquivalenceToAToBConstraint[A, B](equivalenceOfB: Equivalence[B])(implicit ev: A <:< B): A CanEqual B = new AToBEquivalenceConstraint[A, B](equivalenceOfB, ev)
override def typeCheckedConstraint[A, B](implicit equivalenceOfA: Equivalence[A], ev: B <:< A): A CanEqual B = new BToAEquivalenceConstraint[A, B](equivalenceOfA, ev)
override def convertEquivalenceToBToAConstraint[A, B](equivalenceOfA: Equivalence[A])(implicit ev: B <:< A): A CanEqual B = new BToAEquivalenceConstraint[A, B](equivalenceOfA, ev)
@deprecated("The lowPriorityConversionCheckedConstraint method has been deprecated and will be removed in a future version of ScalaTest. It is no longer needed now that the deprecation period of ConversionCheckedTripleEquals has expired. It will not be replaced.", "3.1.0")
override def lowPriorityConversionCheckedConstraint[A, B](implicit equivalenceOfB: Equivalence[B], cnv: A => B): A CanEqual B = new AToBEquivalenceConstraint[A, B](equivalenceOfB, cnv)
@deprecated("The convertEquivalenceToAToBConversionConstraint method has been deprecated and will be removed in a future version of ScalaTest. It is no longer needed now that the deprecation period of ConversionCheckedTripleEquals has expired. It will not be replaced.", "3.1.0")
override def convertEquivalenceToAToBConversionConstraint[A, B](equivalenceOfB: Equivalence[B])(implicit ev: A => B): A CanEqual B = new AToBEquivalenceConstraint[A, B](equivalenceOfB, ev)
@deprecated("The conversionCheckedConstraint method has been deprecated and will be removed in a future version of ScalaTest. It is no longer needed now that the deprecation period of ConversionCheckedTripleEquals has expired. It will not be replaced.", "3.1.0")
override def conversionCheckedConstraint[A, B](implicit equivalenceOfA: Equivalence[A], cnv: B => A): A CanEqual B = new BToAEquivalenceConstraint[A, B](equivalenceOfA, cnv)
@deprecated("The convertEquivalenceToBToAConversionConstraint method has been deprecated and will be removed in a future version of ScalaTest. It is no longer needed now that the deprecation period of ConversionCheckedTripleEquals has expired. It will not be replaced.", "3.1.0")
override def convertEquivalenceToBToAConversionConstraint[A, B](equivalenceOfA: Equivalence[A])(implicit ev: B => A): A CanEqual B = new BToAEquivalenceConstraint[A, B](equivalenceOfA, ev)
}
/**
* Companion object to trait TripleEquals
that facilitates the importing of TripleEquals
members as
* an alternative to mixing it in. One use case is to import TripleEquals
members so you can use
* them in the Scala interpreter:
*
*
* $ scala -classpath scalatest.jar
* Welcome to Scala version 2.10.0
* Type in expressions to have them evaluated.
* Type :help for more information.
*
* scala> import org.scalactic._
* import org.scalactic._
*
* scala> import TripleEquals._
* import TripleEquals._
*
* scala> 1 + 1 === 2
* res0: Boolean = true
*
*/
object TripleEquals extends TripleEquals