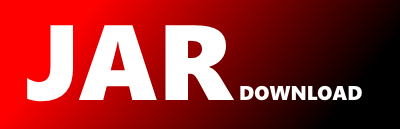
org.scalactic.anyvals.NegDouble.scala Maven / Gradle / Ivy
/*
* Copyright 2001-2016 Artima, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.scalactic.anyvals
import scala.collection.immutable.NumericRange
import scala.language.implicitConversions
import scala.util.{Try, Success, Failure}
import org.scalactic.{Validation, Pass, Fail}
import org.scalactic.{Or, Good, Bad}
/**
* An AnyVal
for negative Double
s.
*
*
*
*
*
*
* Because NegDouble
is an AnyVal
it
* will usually be as efficient as an Double
, being
* boxed only when a Double
would have been boxed.
*
*
*
* The NegDouble.apply
factory method is
* implemented in terms of a macro that checks literals for
* validity at compile time. Calling
* NegDouble.apply
with a literal
* Double
value will either produce a valid
* NegDouble
instance at run time or an error at
* compile time. Here's an example:
*
*
*
* scala> import anyvals._
* import anyvals._
*
* scala> NegDouble(-1.1)
* res1: org.scalactic.anyvals.NegDouble = NegDouble(-1.1)
*
* scala> NegDouble(1.1)
* <console>:14: error: NegDouble.apply can only be invoked on a negative (i < 0.0) floating point literal, like NegDouble(-1.1).
* NegDouble(1.1)
* ^
*
*
*
* NegDouble.apply
cannot be used if the value
* being passed is a variable (i.e., not a literal),
* because the macro cannot determine the validity of variables
* at compile time (just literals). If you try to pass a
* variable to NegDouble.apply
, you'll get a
* compiler error that suggests you use a different factor
* method, NegDouble.from
, instead:
*
*
*
* scala> val x = -1.1
* x: Double = -1.1
*
* scala> NegDouble(x)
* <console>:15: error: NegDouble.apply can only be invoked on a floating point literal, like NegDouble(-1.1). Please use NegDouble.from instead.
* NegDouble(x)
* ^
*
*
*
* The NegDouble.from
factory method will inspect
* the value at runtime and return an
* Option[NegDouble]
. If the value is valid,
* NegDouble.from
will return a
* Some[NegDouble]
, else it will return a
* None
. Here's an example:
*
*
*
* scala> NegDouble.from(x)
* res4: Option[org.scalactic.anyvals.NegDouble] = Some(NegDouble(-1.1))
*
* scala> val y = 1.1
* y: Double = 1.1
*
* scala> NegDouble.from(y)
* res5: Option[org.scalactic.anyvals.NegDouble] = None
*
*
*
* The NegDouble.apply
factory method is marked
* implicit, so that you can pass literal Double
s
* into methods that require NegDouble
, and get the
* same compile-time checking you get when calling
* NegDouble.apply
explicitly. Here's an example:
*
*
*
* scala> def invert(pos: NegDouble): Double = Double.MaxValue - pos
* invert: (pos: org.scalactic.anyvals.NegDouble)Double
*
* scala> invert(1.1)
* res6: Double = 1.7976931348623157E308
*
* scala> invert(Double.MaxValue)
* res8: Double = 0.0
*
* scala> invert(1.1)
* <console>:15: error: NegDouble.apply can only be invoked on a negative (i < 0.0) floating point literal, like NegDouble(-1.1).
* invert(1.1)
* ^
*
*
*
*
* This example also demonstrates that the
* NegDouble
companion object also defines implicit
* widening conversions when a similar conversion is provided in
* Scala. This makes it convenient to use a
* NegDouble
where a Double
is
* needed. An example is the subtraction in the body of the
* invert
method defined above,
* Double.MaxValue - pos
. Although
* Double.MaxValue
is a Double
, which
* has no -
method that takes a
* NegDouble
(the type of pos
), you
* can still subtract pos
, because the
* NegDouble
will be implicitly widened to
* Double
.
*
*
* @param value The Double
value underlying this NegDouble
.
*/
final class NegDouble private (val value: Double) extends AnyVal {
/**
* A string representation of this NegDouble
.
*/
override def toString: String = s"NegDouble(${value.toString()})"
/**
* Converts this NegDouble
to a Byte
.
*/
def toByte: Byte = value.toByte
/**
* Converts this NegDouble
to a Short
.
*/
def toShort: Short = value.toShort
/**
* Converts this NegDouble
to a Char
.
*/
def toChar: Char = value.toChar
/**
* Converts this NegDouble
to an Int
.
*/
def toInt: Int = value.toInt
/**
* Converts this NegDouble
to a Long
.
*/
def toLong: Long = value.toLong
/**
* Converts this NegDouble
to a Float
.
*/
def toFloat: Float = value.toFloat
/**
* Converts this NegDouble
to a Double
.
*/
def toDouble: Double = value.toDouble
/** Returns this value, unmodified. */
def unary_+ : NegDouble = this
/** Returns the negation of this value. */
def unary_- : PosDouble = PosDouble.ensuringValid(-value)
/**
* Converts this NegDouble
's value to a string then concatenates the given string.
*/
def +(x: String): String = s"${value.toString()}${x.toString()}"
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Byte): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Short): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Char): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Int): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Long): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Float): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Double): Boolean = value < x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Byte): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Short): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Char): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Int): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Long): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Float): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Double): Boolean = value <= x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Byte): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Short): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Char): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Int): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Long): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Float): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Double): Boolean = value > x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Byte): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Short): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Char): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Int): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Long): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Float): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Double): Boolean = value >= x
/** Returns the sum of this value and `x`. */
def +(x: Byte): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Short): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Char): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Int): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Long): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Float): Double = value + x
/** Returns the sum of this value and `x`. */
def +(x: Double): Double = value + x
/** Returns the difference of this value and `x`. */
def -(x: Byte): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Short): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Char): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Int): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Long): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Float): Double = value - x
/** Returns the difference of this value and `x`. */
def -(x: Double): Double = value - x
/** Returns the product of this value and `x`. */
def *(x: Byte): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Short): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Char): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Int): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Long): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Float): Double = value * x
/** Returns the product of this value and `x`. */
def *(x: Double): Double = value * x
/** Returns the quotient of this value and `x`. */
def /(x: Byte): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Short): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Char): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Int): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Long): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Float): Double = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Double): Double = value / x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Byte): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Short): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Char): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Int): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Long): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Float): Double = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Double): Double = value % x
// TODO: Need Scaladoc
// Stuff from RichDouble
/**
* Returns this
if this > that
or that
otherwise.
*/
def max(that: NegDouble): NegDouble = if (math.max(value, that.value) == value) this else that
/**
* Returns this
if this < that
or that
otherwise.
*/
def min(that: NegDouble): NegDouble = if (math.min(value, that.value) == value) this else that
/**
* Indicates whether this `NegDouble` has a value that is a whole number: it is finite and it has no fraction part.
*/
def isWhole = {
val longValue = value.toLong
longValue.toDouble == value || longValue == Long.MaxValue && value < Double.PositiveInfinity || longValue == Long.MinValue && value > Double.NegativeInfinity
}
/** Converts an angle measured in degrees to an approximately equivalent
* angle measured in radians.
*
* @return the measurement of the angle x in radians.
*/
def toRadians: Double = math.toRadians(value)
/** Converts an angle measured in radians to an approximately equivalent
* angle measured in degrees.
* @return the measurement of the angle x in degrees.
*/
def toDegrees: Double = math.toDegrees(value)
/**
* Applies the passed Double => Double
function to the underlying Double
* value, and if the result is positive, returns the result wrapped in a NegDouble
,
* else throws AssertionError
.
*
*
* This method will inspect the result of applying the given function to this
* NegDouble
's underlying Double
value and if the result
* is greater than 0.0
, it will return a NegDouble
representing that value.
* Otherwise, the Double
value returned by the given function is
* 0.0
or negative, so this method will throw AssertionError
.
*
*
*
* This method differs from a vanilla assert
or ensuring
* call in that you get something you didn't already have if the assertion
* succeeds: a type that promises an Double
is positive.
* With this method, you are asserting that you are convinced the result of
* the computation represented by applying the given function to this NegDouble
's
* value will not produce zero, a negative number, including Double.NegativeInfinity
, or Double.NaN
.
* Instead of producing such invalid values, this method will throw AssertionError
.
*
*
* @param f the Double => Double
function to apply to this NegDouble
's
* underlying Double
value.
* @return the result of applying this NegDouble
's underlying Double
value to
* to the passed function, wrapped in a NegDouble
if it is positive (else throws AssertionError
).
* @throws AssertionError if the result of applying this NegDouble
's underlying Double
value to
* to the passed function is not positive.
*/
def ensuringValid(f: Double => Double): NegDouble = {
val candidateResult: Double = f(value)
if (NegDoubleMacro.isValid(candidateResult)) new NegDouble(candidateResult)
else throw new AssertionError(s"${candidateResult.toString()}, the result of applying the passed function to ${value.toString()}, was not a valid NegDouble")
}
/**
* Rounds this `NegDouble` value to the nearest whole number value that can be expressed as an `NegZLong`, returning the result as a `NegZLong`.
*/
def round: NegZLong = NegZLong.ensuringValid(math.round(value))
/**
* Returns the smallest (closest to 0) `NegZDouble` that is greater than or equal to this `NegZDouble`
* and represents a mathematical integer.
*/
def ceil: NegZDouble = NegZDouble.ensuringValid(math.ceil(value).toDouble)
/**
* Returns the greatest (closest to infinity) `NegDouble` that is less than or equal to
* this `NegDouble` and represents a mathematical integer.
*/
def floor: NegDouble = NegDouble.ensuringValid(math.floor(value).toDouble)
/**
* Returns the NegDouble
sum of this NegDouble
's value and the given NegZDouble
value.
*
*
* This method will always succeed (not throw an exception) because
* adding a negative Double and non-positive Double and another
* negative Double will always result in another negative Double
* value (though the result may be infinity).
*
*/
def plus(x: NegZDouble): NegDouble = NegDouble.ensuringValid(value + x.value)
/**
* True if this NegDouble
value represents negative infinity, else false.
*/
def isNegInfinity: Boolean = Double.NegativeInfinity == value
/**
* True if this NegDouble
value is any finite value (i.e., it is neither positive nor negative infinity), else false.
*/
def isFinite: Boolean = !value.isInfinite
}
/**
* The companion object for NegDouble
that offers
* factory methods that produce NegDouble
s,
* implicit widening conversions from NegDouble
to
* other numeric types, and maximum and minimum constant values
* for NegDouble
.
*/
object NegDouble {
/**
* The largest value representable as a negative Double
,
* which is NegDouble(-4.9E-324)
.
*/
final val MaxValue: NegDouble = NegDouble.ensuringValid(-Double.MinPositiveValue)
/**
* The smallest value representable as a negative
* Double
, which is NegDouble(-1.7976931348623157E308)
.
*/
final val MinValue: NegDouble = NegDouble.ensuringValid(Double.MinValue) // Can't use the macro here
/**
* A factory method that produces an Option[NegDouble]
given a
* Double
value.
*
*
* This method will inspect the passed Double
value and if
* it is a negative Double
, it will return a NegDouble
* representing that value, wrapped in a Some
. Otherwise, the passed Double
* value is not negative, so this method will return None
.
*
*
*
* This factory method differs from the apply
* factory method in that apply
is implemented
* via a macro that inspects Double
literals at
* compile time, whereas from
inspects
* Double
values at run time.
*
*
* @param value the Double
to inspect, and if negative, return
* wrapped in a Some[NegDouble]
.
* @return the specified Double
value wrapped in a
* Some[NegDouble]
, if it is NegDouble, else
* None
.
*/
def from(value: Double): Option[NegDouble] =
if (NegDoubleMacro.isValid(value)) Some(new NegDouble(value)) else None
/**
* A factory/assertion method that produces a NegDouble
given a
* valid Double
value, or throws AssertionError
,
* if given an invalid Double
value.
*
*
* This method will inspect the passed Double
value and if
* it is a negative Double
, it will return a NegDouble
* representing that value. Otherwise, the passed Double
value is not negative,
* so this method will throw AssertionError
.
*
*
*
* This factory method differs from the apply
* factory method in that apply
is implemented
* via a macro that inspects Double
literals at
* compile time, whereas from
inspects
* Double
values at run time.
* It differs from a vanilla assert
or ensuring
* call in that you get something you didn't already have if the assertion
* succeeds: a type that promises a Double
is negative.
*
*
* @param value the Double
to inspect, and if negative, return
* wrapped in a NegDouble
.
* @return the specified Double
value wrapped in a
* NegDouble
, if it is negative, else
* throws AssertionError
.
* @throws AssertionError if the passed value is not negative
*/
def ensuringValid(value: Double): NegDouble =
if (NegDoubleMacro.isValid(value)) new NegDouble(value) else {
throw new AssertionError(s"${value.toString()} was not a valid NegDouble")
}
/**
* A factory/validation method that produces a NegDouble
, wrapped
* in a Success
, given a valid Float
value, or if the
* given Float
is invalid, an AssertionError
, wrapped
* in a Failure
.
*
* Note: you should use this method only when you are convinced that it will
* always succeed, i.e., never throw an exception. It is good practice to
* add a comment near the invocation of this method indicating ''why'' you think
* it will always succeed to document your reasoning. If you are not sure an
* `ensuringValid` call will always succeed, you should use one of the other
* factory or validation methods provided on this object instead: `isValid`,
* `tryingValid`, `passOrElse`, `goodOrElse`, or `rightOrElse`.
*
*
* This method will inspect the passed Float
value and if
* it is a negative Float
, it will return a NegDouble
* representing that value, wrapped in a Success
.
* Otherwise, the passed Float
value is not negative, so this
* method will return an AssertionError
, wrapped in a Failure
.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Float
literals at compile time, whereas this method inspects
* Float
values at run time.
*
*
* @param value the Float
to inspect, and if negative, return
* wrapped in a Success(NegDouble)
.
* @return the specified Float
value wrapped
* in a Success(NegDouble)
, if it is negative, else a Failure(AssertionError)
.
*/
def tryingValid(value: Double): Try[NegDouble] =
if (NegDoubleMacro.isValid(value))
Success(new NegDouble(value))
else
Failure(new AssertionError(s"${value.toString()} was not a valid NegDouble"))
/**
* A validation method that produces a Pass
* given a valid Double
value, or
* an error value of type E
produced by passing the
* given invalid Int
value
* to the given function f
, wrapped in a Fail
.
*
*
* This method will inspect the passed Double
value and if
* it is a negative Double
, it will return a Pass
.
* Otherwise, the passed Double
value is negative, so this
* method will return a result of type E
obtained by passing
* the invalid Double
value to the given function f
,
* wrapped in a `Fail`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Double
literals at compile time, whereas this method inspects
* Double
values at run time.
*
*
* @param value the `Int` to validate that it is negative.
* @return a `Pass` if the specified `Int` value is negative,
* else a `Fail` containing an error value produced by passing the
* specified `Double` to the given function `f`.
*/
def passOrElse[E](value: Double)(f: Double => E): Validation[E] =
if (NegDoubleMacro.isValid(value)) Pass else Fail(f(value))
/**
* A factory/validation method that produces a NegDouble
, wrapped
* in a Good
, given a valid Double
value, or if the
* given Double
is invalid, an error value of type B
* produced by passing the given invalid Double
value
* to the given function f
, wrapped in a Bad
.
*
*
* This method will inspect the passed Double
value and if
* it is a negative Double
, it will return a NegDouble
* representing that value, wrapped in a Good
.
* Otherwise, the passed Double
value is not negative, so this
* method will return a result of type B
obtained by passing
* the invalid Double
value to the given function f
,
* wrapped in a `Bad`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Double
literals at compile time, whereas this method inspects
* Double
values at run time.
*
*
* @param value the Double
to inspect, and if negative, return
* wrapped in a Good(NegDouble)
.
* @return the specified Double
value wrapped
* in a Good(NegDouble)
, if it is negative, else a Bad(f(value))
.
*/
def goodOrElse[B](value: Double)(f: Double => B): NegDouble Or B =
if (NegDoubleMacro.isValid(value)) Good(NegDouble.ensuringValid(value)) else Bad(f(value))
/**
* A factory/validation method that produces a NegDouble
, wrapped
* in a Right
, given a valid Double
value, or if the
* given Double
is invalid, an error value of type L
* produced by passing the given invalid Double
value
* to the given function f
, wrapped in a Left
.
*
*
* This method will inspect the passed Double
value and if
* it is a negative Double
, it will return a NegDouble
* representing that value, wrapped in a Right
.
* Otherwise, the passed Double
value is not negative, so this
* method will return a result of type L
obtained by passing
* the invalid Double
value to the given function f
,
* wrapped in a `Left`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Double
literals at compile time, whereas this method inspects
* Double
values at run time.
*
*
* @param value the Double
to inspect, and if negative, return
* wrapped in a Right(NegDouble)
.
* @return the specified Double
value wrapped
* in a Right(NegDouble)
, if it is negative, else a Left(f(value))
.
*/
def rightOrElse[L](value: Double)(f: Double => L): Either[L, NegDouble] =
if (NegDoubleMacro.isValid(value)) Right(NegDouble.ensuringValid(value)) else Left(f(value))
/**
* A predicate method that returns true if a given
* Double
value is negative.
*
* @param value the Double
to inspect, and if negative, return true.
* @return true if the specified Double
is positive, else false.
*/
def isValid(value: Double): Boolean = NegDoubleMacro.isValid(value)
/**
* A factory method that produces a NegDouble
given a
* Double
value and a default NegDouble
.
*
*
* This method will inspect the passed Double
value and if
* it is a negative Double
, it will return a NegDouble
* representing that value. Otherwise, the passed Double
value is negative,
* so this method will return the passed default
value.
*
*
*
* This factory method differs from the apply
* factory method in that apply
is implemented
* via a macro that inspects Double
literals at
* compile time, whereas from
inspects
* Double
values at run time.
*
*
* @param value the Double
to inspect, and if negative, return.
* @param default the NegDouble
to return if the passed
* Double
value is not negative.
* @return the specified Double
value wrapped in a
* NegDouble
, if it is negative, else the
* default
NegDouble
value.
*/
def fromOrElse(value: Double, default: => NegDouble): NegDouble =
if (NegDoubleMacro.isValid(value)) new NegDouble(value) else default
import language.experimental.macros
import scala.language.implicitConversions
/**
* A factory method, implemented via a macro, that produces a
* NegDouble
if passed a valid Double
* literal, otherwise a compile time error.
*
*
* The macro that implements this method will inspect the
* specified Double
expression at compile time. If
* the expression is a negative Double
literal,
* it will return a NegDouble
representing that value.
* Otherwise, the passed Double
expression is either a literal
* that is not negative, or is not a literal, so this method
* will give a compiler error.
*
*
*
* This factory method differs from the from
* factory method in that this method is implemented via a
* macro that inspects Double
literals at compile
* time, whereas from
inspects Double
* values at run time.
*
*
* @param value the Double
literal expression to
* inspect at compile time, and if negative, to return
* wrapped in a NegDouble
at run time.
* @return the specified, valid Double
literal
* value wrapped in a NegDouble
. (If the
* specified expression is not a valid Double
* literal, the invocation of this method will not
* compile.)
*/
inline implicit def apply(value: => Double): NegDouble = ${ NegDoubleMacro('{value}) }
/**
* Implicit widening conversion from NegDouble
to
* Double
.
*
* @param pos the NegDouble
to widen
* @return the Double
value underlying the specified
* NegDouble
*/
implicit def widenToDouble(pos: NegDouble): Double = pos.value
/**
* Implicit widening conversion from NegDouble
to NegZDouble
.
*
* @param pos the NegDouble
to widen
* @return the Double
value underlying the specified NegDouble
,
* widened to Double
and wrapped in a NegZDouble
.
*/
implicit def widenToNegZDouble(pos: NegDouble): NegZDouble = NegZDouble.ensuringValid(pos.value)
/**
* Implicit widening conversion from NegDouble
to NonZeroDouble
.
*
* @param pos the NegDouble
to widen
* @return the Double
value underlying the specified NegDouble
,
* widened to Double
and wrapped in a NonZeroDouble
.
*/
implicit def widenToNonZeroDouble(pos: NegDouble): NonZeroDouble = NonZeroDouble.ensuringValid(pos.value)
/**
* Implicit Ordering instance.
*/
implicit val ordering: Ordering[NegDouble] =
new Ordering[NegDouble] {
def compare(x: NegDouble, y: NegDouble): Int = x.toDouble.compare(y.toDouble)
}
/**
* The negative infinity value, which is NegDouble.ensuringValid(Double.NegativeInfinity)
.
*/
final val NegativeInfinity: NegDouble = NegDouble.ensuringValid(Double.NegativeInfinity) // Can't use the macro here
}