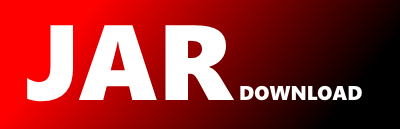
org.scalactic.anyvals.NegZFiniteFloat.scala Maven / Gradle / Ivy
/*
* Copyright 2001-2016 Artima, Inc.
*
* Licensed under the Apache License, Version 2.0 (the "License");
* you may not use this file except in compliance with the License.
* You may obtain a copy of the License at
*
* http://www.apache.org/licenses/LICENSE-2.0
*
* Unless required by applicable law or agreed to in writing, software
* distributed under the License is distributed on an "AS IS" BASIS,
* WITHOUT WARRANTIES OR CONDITIONS OF ANY KIND, either express or implied.
* See the License for the specific language governing permissions and
* limitations under the License.
*/
package org.scalactic.anyvals
import scala.collection.immutable.NumericRange
import scala.language.implicitConversions
import scala.util.{Try, Success, Failure}
import org.scalactic.{Validation, Pass, Fail}
import org.scalactic.{Or, Good, Bad}
/**
* An AnyVal
for finite non-positive Float
s.
*
*
*
*
*
*
* Because NegZFiniteFloat
is an AnyVal
it
* will usually be as efficient as an Float
, being
* boxed only when an Float
would have been boxed.
*
*
*
* The NegZFiniteFloat.apply
factory method is implemented
* in terms of a macro that checks literals for validity at
* compile time. Calling NegZFiniteFloat.apply
with a
* literal Float
value will either produce a valid
* NegZFiniteFloat
instance at run time or an error at
* compile time. Here's an example:
*
*
*
* scala> import anyvals._
* import anyvals._
*
* scala> NegZFiniteFloat(-1.1fF)
* res0: org.scalactic.anyvals.NegZFiniteFloat = NegZFiniteFloat(-1.1f)
*
* scala> NegZFiniteFloat(1.1fF)
* <console>:14: error: NegZFiniteFloat.apply can only be invoked on a finite non-positive (i <= 0.0f && i != Float.NegativeInfinity) floating point literal, like NegZFiniteFloat(-1.1fF).
* NegZFiniteFloat(-1.1fF)
* ^
*
*
*
* NegZFiniteFloat.apply
cannot be used if the value being
* passed is a variable (i.e., not a literal), because
* the macro cannot determine the validity of variables at
* compile time (just literals). If you try to pass a variable
* to NegZFiniteFloat.apply
, you'll get a compiler error
* that suggests you use a different factor method,
* NegZFiniteFloat.from
, instead:
*
*
*
* scala> val x = -1.1fF
* x: Float = -1.1f
*
* scala> NegZFiniteFloat(x)
* <console>:15: error: NegZFiniteFloat.apply can only be invoked on a floating point literal, like NegZFiniteFloat(-1.1fF). Please use NegZFiniteFloat.from instead.
* NegZFiniteFloat(x)
* ^
*
*
*
* The NegZFiniteFloat.from
factory method will inspect
* the value at runtime and return an
* Option[NegZFiniteFloat]
. If the value is valid,
* NegZFiniteFloat.from
will return a
* Some[NegZFiniteFloat]
, else it will return a
* None
. Here's an example:
*
*
*
* scala> NegZFiniteFloat.from(x)
* res3: Option[org.scalactic.anyvals.NegZFiniteFloat] = Some(NegZFiniteFloat(-1.1f))
*
* scala> val y = 1.1fF
* y: Float = 1.1f
*
* scala> NegZFiniteFloat.from(y)
* res4: Option[org.scalactic.anyvals.NegZFiniteFloat] = None
*
*
*
* The NegZFiniteFloat.apply
factory method is marked
* implicit, so that you can pass literal Float
s
* into methods that require NegZFiniteFloat
, and get the
* same compile-time checking you get when calling
* NegZFiniteFloat.apply
explicitly. Here's an example:
*
*
*
* scala> def invert(pos: NegZFiniteFloat): Float = Float.MaxValue - pos
* invert: (pos: org.scalactic.anyvals.NegZFiniteFloat)Float
*
* scala> invert(-1.1fF)
* res5: Float = 3.4028235E38
*
* scala> invert(Float.MaxValue)
* res6: Float = 0.0
*
* scala> invert(1.1fF)
* <console>:15: error: NegZFiniteFloat.apply can only be invoked on a finite non-positive (i <= 0.0f && i != Float.NegativeInfinity) floating point literal, like NegZFiniteFloat(-1.1fF).
* invert(0.0F)
* ^
*
* scala> invert(1.1fF)
* <console>:15: error: NegZFiniteFloat.apply can only be invoked on a finite non-positive (i <= 0.0f && i != Float.NegativeInfinity) floating point literal, like NegZFiniteFloat(-1.1fF).
* invert(1.1fF)
* ^
*
*
*
*
* This example also demonstrates that the NegZFiniteFloat
* companion object also defines implicit widening conversions
* when no loss of precision will occur. This makes it convenient to use a
* NegZFiniteFloat
where a Float
or wider
* type is needed. An example is the subtraction in the body of
* the invert
method defined above,
* Float.MaxValue - pos
. Although
* Float.MaxValue
is a Float
, which
* has no -
method that takes a
* NegZFiniteFloat
(the type of pos
), you can
* still subtract pos
, because the
* NegZFiniteFloat
will be implicitly widened to
* Float
.
*
*
* @param value The Float
value underlying this NegZFiniteFloat
.
*/
final class NegZFiniteFloat private (val value: Float) extends AnyVal {
/**
* A string representation of this NegZFiniteFloat
.
*/
override def toString: String = s"NegZFiniteFloat(${value.toString()}f)"
/**
* Converts this NegZFiniteFloat
to a Byte
.
*/
def toByte: Byte = value.toByte
/**
* Converts this NegZFiniteFloat
to a Short
.
*/
def toShort: Short = value.toShort
/**
* Converts this NegZFiniteFloat
to a Char
.
*/
def toChar: Char = value.toChar
/**
* Converts this NegZFiniteFloat
to an Int
.
*/
def toInt: Int = value.toInt
/**
* Converts this NegZFiniteFloat
to a Long
.
*/
def toLong: Long = value.toLong
/**
* Converts this NegZFiniteFloat
to a Float
.
*/
def toFloat: Float = value.toFloat
/**
* Converts this NegZFiniteFloat
to a Double
.
*/
def toDouble: Double = value.toDouble
/** Returns this value, unmodified. */
def unary_+ : NegZFiniteFloat = this
/** Returns the negation of this value. */
def unary_- : PosZFiniteFloat = PosZFiniteFloat.ensuringValid(-value)
/**
* Converts this NegZFiniteFloat
's value to a string then concatenates the given string.
*/
def +(x: String): String = s"${value.toString()}${x.toString()}"
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Byte): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Short): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Char): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Int): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Long): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Float): Boolean = value < x
/** Returns `true` if this value is less than x, `false` otherwise. */
def <(x: Double): Boolean = value < x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Byte): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Short): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Char): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Int): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Long): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Float): Boolean = value <= x
/** Returns `true` if this value is less than or equal to x, `false` otherwise. */
def <=(x: Double): Boolean = value <= x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Byte): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Short): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Char): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Int): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Long): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Float): Boolean = value > x
/** Returns `true` if this value is greater than x, `false` otherwise. */
def >(x: Double): Boolean = value > x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Byte): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Short): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Char): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Int): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Long): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Float): Boolean = value >= x
/** Returns `true` if this value is greater than or equal to x, `false` otherwise. */
def >=(x: Double): Boolean = value >= x
/** Returns the sum of this value and `x`. */
def +(x: Byte): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Short): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Char): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Int): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Long): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Float): Float = value + x
/** Returns the sum of this value and `x`. */
def +(x: Double): Double = value + x
/** Returns the difference of this value and `x`. */
def -(x: Byte): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Short): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Char): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Int): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Long): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Float): Float = value - x
/** Returns the difference of this value and `x`. */
def -(x: Double): Double = value - x
/** Returns the product of this value and `x`. */
def *(x: Byte): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Short): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Char): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Int): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Long): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Float): Float = value * x
/** Returns the product of this value and `x`. */
def *(x: Double): Double = value * x
/** Returns the quotient of this value and `x`. */
def /(x: Byte): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Short): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Char): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Int): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Long): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Float): Float = value / x
/** Returns the quotient of this value and `x`. */
def /(x: Double): Double = value / x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Byte): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Short): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Char): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Int): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Long): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Float): Float = value % x
/** Returns the remainder of the division of this value by `x`. */
def %(x: Double): Double = value % x
// Stuff from RichFloat
/**
* Returns this
if this > that
or that
otherwise.
*/
def max(that: NegZFiniteFloat): NegZFiniteFloat = if (math.max(value, that.value) == value) this else that
/**
* Returns this
if this < that
or that
otherwise.
*/
def min(that: NegZFiniteFloat): NegZFiniteFloat = if (math.min(value, that.value) == value) this else that
/**
* Indicates whether this `NegZFiniteFloat` has a value that is a whole number: it is finite and it has no fraction part.
*/
def isWhole = {
val longValue = value.toLong
longValue.toFloat == value || longValue == Long.MaxValue && value < Float.PositiveInfinity || longValue == Long.MinValue && value > Float.NegativeInfinity
}
/** Converts an angle measured in degrees to an approximately equivalent
* angle measured in radians.
*
* @return the measurement of the angle x in radians.
*/
def toRadians: Float = math.toRadians(value.toDouble).toFloat
/** Converts an angle measured in radians to an approximately equivalent
* angle measured in degrees.
* @return the measurement of the angle x in degrees.
*/
def toDegrees: Float = math.toDegrees(value.toDouble).toFloat
/**
* Applies the passed Float => Float
function to the underlying Float
* value, and if the result is positive, returns the result wrapped in a NegZFiniteFloat
,
* else throws AssertionError
.
*
*
* This method will inspect the result of applying the given function to this
* NegZFiniteFloat
's underlying Float
value and if the result
* is finite non-positive, it will return a NegZFiniteFloat
representing that value.
* Otherwise, the Float
value returned by the given function is
* not finite non-positive, so this method will throw AssertionError
.
*
*
*
* This method differs from a vanilla assert
or ensuring
* call in that you get something you didn't already have if the assertion
* succeeds: a type that promises an Float
is finite non-positive.
* With this method, you are asserting that you are convinced the result of
* the computation represented by applying the given function to this NegZFiniteFloat
's
* value will not produce invalid value.
* Instead of producing such invalid values, this method will throw AssertionError
.
*
*
* @param f the Float => Float
function to apply to this NegZFiniteFloat
's
* underlying Float
value.
* @return the result of applying this NegZFiniteFloat
's underlying Float
value to
* to the passed function, wrapped in a NegZFiniteFloat
if it is finite non-positive (else throws AssertionError
).
* @throws AssertionError if the result of applying this NegZFiniteFloat
's underlying Float
value to
* to the passed function is not finite non-positive.
*/
def ensuringValid(f: Float => Float): NegZFiniteFloat = {
val candidateResult: Float = f(value)
if (NegZFiniteFloatMacro.isValid(candidateResult)) new NegZFiniteFloat(candidateResult)
else throw new AssertionError(s"${candidateResult.toString()}, the result of applying the passed function to ${value.toString()}, was not a valid NegZFiniteFloat")
}
/**
* Rounds this `NegZFiniteFloat` value to the nearest whole number value that can be expressed as an `NegZInt`, returning the result as a `NegZInt`.
*/
def round: NegZInt = NegZInt.ensuringValid(math.round(value))
/**
* Returns the smallest (closest to 0) `NegZFiniteFloat` that is greater than or equal to this `NegZFiniteFloat`
* and represents a mathematical integer.
*/
def ceil: NegZFiniteFloat = NegZFiniteFloat.ensuringValid(math.ceil(value).toFloat)
/**
* Returns the greatest (closest to infinity) `NegZFiniteFloat` that is less than or equal to
* this `NegZFiniteFloat` and represents a mathematical integer.
*/
def floor: NegZFiniteFloat = NegZFiniteFloat.ensuringValid(math.floor(value).toFloat)
}
/**
* The companion object for NegZFiniteFloat
that offers
* factory methods that produce NegZFiniteFloat
s,
* implicit widening conversions from NegZFiniteFloat
to
* other numeric types, and maximum and minimum constant values
* for NegZFiniteFloat
.
*/
object NegZFiniteFloat {
/**
* The largest value representable as a finite non-positive Float
,
* which is NegZFiniteFloat(0.0f)
.
*/
final val MaxValue: NegZFiniteFloat = NegZFiniteFloat.ensuringValid(0.0f)
/**
* The smallest value representable as a finite non-positive
* Float
, which is NegZFiniteFloat(-3.4028235E38)
.
*/
final val MinValue: NegZFiniteFloat = NegZFiniteFloat.ensuringValid(Float.MinValue) // Can't use the macro here
/**
* A factory method that produces an Option[NegZFiniteFloat]
given a
* Float
value.
*
*
* This method will inspect the passed Float
value and if
* it is a finite non-positive Float
, it will return a NegZFiniteFloat
* representing that value wrapped in a Some
. Otherwise, the passed Float
* value is not finite non-positive, so this method will return None
.
*
*
*
* This factory method differs from the apply
* factory method in that apply
is implemented
* via a macro that inspects Float
literals at
* compile time, whereas from
inspects
* Float
values at run time.
*
*
* @param value the Float
to inspect, and if finite non-positive, return
* wrapped in a Some[NegZFiniteFloat]
.
* @return the specified Float
value wrapped in a
* Some[NegZFiniteFloat]
, if it is finite non-positive, else
* None
.
*/
def from(value: Float): Option[NegZFiniteFloat] =
if (NegZFiniteFloatMacro.isValid(value)) Some(new NegZFiniteFloat(value)) else None
/**
* A factory/assertion method that produces a NegZFiniteFloat
given a
* valid Float
value, or throws AssertionError
,
* if given an invalid Float
value.
*
* Note: you should use this method only when you are convinced that it will
* always succeed, i.e., never throw an exception. It is good practice to
* add a comment near the invocation of this method indicating ''why'' you think
* it will always succeed to document your reasoning. If you are not sure an
* `ensuringValid` call will always succeed, you should use one of the other
* factory or validation methods provided on this object instead: `isValid`,
* `tryingValid`, `passOrElse`, `goodOrElse`, or `rightOrElse`.
*
*
* This method will inspect the passed Float
value and if
* it is a finite non-positive Float
, it will return a NegZFiniteFloat
representing that value.
* Otherwise, the passed Float
value is not finite non-positive, so
* this method will throw AssertionError
.
*
*
*
* This factory method differs from the apply
* factory method in that apply
is implemented
* via a macro that inspects Float
literals at
* compile time, whereas from
inspects
* Float
values at run time.
* It differs from a vanilla assert
or ensuring
* call in that you get something you didn't already have if the assertion
* succeeds: a type that promises a Float
is positive.
*
*
* @param value the Float
to inspect, and if finite non-positive, return
* wrapped in a NegZFiniteFloat
.
* @return the specified Float
value wrapped in a
* NegZFiniteFloat
, if it is finite non-positive, else
* throws AssertionError
.
* @throws AssertionError if the passed value is not finite non-positive
*/
def ensuringValid(value: Float): NegZFiniteFloat =
if (NegZFiniteFloatMacro.isValid(value)) new NegZFiniteFloat(value) else {
throw new AssertionError(s"${value.toString()} was not a valid NegZFiniteFloat")
}
/**
* A factory/validation method that produces a NegZFiniteFloat
, wrapped
* in a Success
, given a valid Float
value, or if the
* given Float
is invalid, an AssertionError
, wrapped
* in a Failure
.
*
*
* This method will inspect the passed Float
value and if
* it is a finite non-positive Float
, it will return a NegZFiniteFloat
* representing that value, wrapped in a Success
.
* Otherwise, the passed Float
value is not finite non-positive, so this
* method will return an AssertionError
, wrapped in a Failure
.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Float
literals at compile time, whereas this method inspects
* Float
values at run time.
*
*
* @param value the Float
to inspect, and if finite non-positive, return
* wrapped in a Success(NegZFiniteFloat)
.
* @return the specified Float
value wrapped
* in a Success(NegZFiniteFloat)
, if it is finite non-positive, else a Failure(AssertionError)
.
*/
def tryingValid(value: Float): Try[NegZFiniteFloat] =
if (NegZFiniteFloatMacro.isValid(value))
Success(new NegZFiniteFloat(value))
else
Failure(new AssertionError(s"${value.toString()} was not a valid NegZFiniteFloat"))
/**
* A validation method that produces a Pass
* given a valid Float
value, or
* an error value of type E
produced by passing the
* given invalid Int
value
* to the given function f
, wrapped in a Fail
.
*
*
* This method will inspect the passed Float
value and if
* it is a finite non-positive Float
, it will return a Pass
.
* Otherwise, the passed Float
value is finite non-positive, so this
* method will return a result of type E
obtained by passing
* the invalid Float
value to the given function f
,
* wrapped in a `Fail`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Float
literals at compile time, whereas this method inspects
* Float
values at run time.
*
*
* @param value the `Float` to validate that it is finite non-positive.
* @return a `Pass` if the specified `Float` value is finite non-positive,
* else a `Fail` containing an error value produced by passing the
* specified `Float` to the given function `f`.
*/
def passOrElse[E](value: Float)(f: Float => E): Validation[E] =
if (NegZFiniteFloatMacro.isValid(value)) Pass else Fail(f(value))
/**
* A factory/validation method that produces a NegZFiniteFloat
, wrapped
* in a Good
, given a valid Float
value, or if the
* given Float
is invalid, an error value of type B
* produced by passing the given invalid Float
value
* to the given function f
, wrapped in a Bad
.
*
*
* This method will inspect the passed Float
value and if
* it is a finite non-positive Float
, it will return a NegZFiniteFloat
* representing that value, wrapped in a Good
.
* Otherwise, the passed Float
value is not finite non-positive, so this
* method will return a result of type B
obtained by passing
* the invalid Float
value to the given function f
,
* wrapped in a `Bad`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Float
literals at compile time, whereas this method inspects
* Float
values at run time.
*
*
* @param value the Float
to inspect, and if finite non-positive, return
* wrapped in a Good(NegZFiniteFloat)
.
* @return the specified Float
value wrapped
* in a Good(NegZFiniteFloat)
, if it is finite non-positive, else a Bad(f(value))
.
*/
def goodOrElse[B](value: Float)(f: Float => B): NegZFiniteFloat Or B =
if (NegZFiniteFloatMacro.isValid(value)) Good(NegZFiniteFloat.ensuringValid(value)) else Bad(f(value))
/**
* A factory/validation method that produces a NegZFiniteFloat
, wrapped
* in a Right
, given a valid Int
value, or if the
* given Int
is invalid, an error value of type L
* produced by passing the given invalid Int
value
* to the given function f
, wrapped in a Left
.
*
*
* This method will inspect the passed Int
value and if
* it is a finite non-positive Int
, it will return a NegZFiniteFloat
* representing that value, wrapped in a Right
.
* Otherwise, the passed Int
value is not finite non-positive, so this
* method will return a result of type L
obtained by passing
* the invalid Int
value to the given function f
,
* wrapped in a `Left`.
*
*
*
* This factory method differs from the apply
factory method
* in that apply
is implemented via a macro that inspects
* Int
literals at compile time, whereas this method inspects
* Int
values at run time.
*
*
* @param value the Int
to inspect, and if finite non-positive, return
* wrapped in a Right(NegZFiniteFloat)
.
* @return the specified Int
value wrapped
* in a Right(NegZFiniteFloat)
, if it is finite non-positive, else a Left(f(value))
.
*/
def rightOrElse[L](value: Float)(f: Float => L): Either[L, NegZFiniteFloat] =
if (NegZFiniteFloatMacro.isValid(value)) Right(NegZFiniteFloat.ensuringValid(value)) else Left(f(value))
/**
* A predicate method that returns true if a given
* Float
value is finite non-positive.
*
* @param value the Float
to inspect, and if finite non-positive, return true.
* @return true if the specified Float
is finite non-positive, else false.
*/
def isValid(value: Float): Boolean = NegZFiniteFloatMacro.isValid(value)
/**
* A factory method that produces a NegZFiniteFloat
given a
* Float
value and a default NegZFiniteFloat
.
*
*
* This method will inspect the passed Float
value and if
* it is a finite non-positive Float
, it will return a NegZFiniteFloat
representing that value.
* Otherwise, the passed Float
value is not finite non-positive, so this
* method will return the passed default
value.
*
*
*
* This factory method differs from the apply
* factory method in that apply
is implemented
* via a macro that inspects Float
literals at
* compile time, whereas from
inspects
* Float
values at run time.
*
*
* @param value the Float
to inspect, and if finite non-positive, return.
* @param default the NegZFiniteFloat
to return if the passed
* Float
value is not finite non-positive.
* @return the specified Float
value wrapped in a
* NegZFiniteFloat
, if it is finite non-positive, else the
* default
NegZFiniteFloat
value.
*/
def fromOrElse(value: Float, default: => NegZFiniteFloat): NegZFiniteFloat =
if (NegZFiniteFloatMacro.isValid(value)) new NegZFiniteFloat(value) else default
import language.experimental.macros
import scala.language.implicitConversions
/**
* A factory method, implemented via a macro, that produces a
* NegZFiniteFloat
if passed a valid Float
* literal, otherwise a compile time error.
*
*
* The macro that implements this method will inspect the
* specified Float
expression at compile time. If
* the expression is a finite non-positive Float
literal,
* it will return a NegZFiniteFloat
representing that value.
* Otherwise, the passed Float
expression is either a literal
* that is not finite non-positive, or is not a literal, so this method
* will give a compiler error.
*
*
*
* This factory method differs from the from
* factory method in that this method is implemented via a
* macro that inspects Float
literals at compile
* time, whereas from
inspects Float
* values at run time.
*
*
* @param value the Float
literal expression to
* inspect at compile time, and if finite non-positive, to return
* wrapped in a NegZFiniteFloat
at run time.
* @return the specified, valid Float
literal
* value wrapped in a NegZFiniteFloat
. (If the
* specified expression is not a valid Float
* literal, the invocation of this method will not
* compile.)
*/
inline implicit def apply(value: => Float): NegZFiniteFloat = ${ NegZFiniteFloatMacro('{value}) }
/**
* Implicit widening conversion from NegZFiniteFloat
to
* Float
.
*
* @param pos the NegZFiniteFloat
to widen
* @return the Float
value underlying the
* specified NegZFiniteFloat
*/
implicit def widenToFloat(pos: NegZFiniteFloat): Float = pos.value
/**
* Implicit widening conversion from NegZFiniteFloat
to
* Double
.
*
* @param pos the NegZFiniteFloat
to widen
* @return the Float
value underlying the
* specified NegZFiniteFloat
, widened to
* Double
.
*/
implicit def widenToDouble(pos: NegZFiniteFloat): Double = pos.value
/**
* Implicit widening conversion from NegZFiniteFloat
to NegZFloat
.
*
* @param pos the NegZFiniteFloat
to widen
* @return the Float
value underlying the specified NegZFiniteFloat
,
* widened to Float
and wrapped in a NegZFloat
.
*/
implicit def widenToNegZFloat(pos: NegZFiniteFloat): NegZFloat = NegZFloat.ensuringValid(pos.value)
/**
* Implicit widening conversion from NegZFiniteFloat
to NegZDouble
.
*
* @param pos the NegZFiniteFloat
to widen
* @return the Float
value underlying the specified NegZFiniteFloat
,
* widened to Double
and wrapped in a NegZDouble
.
*/
implicit def widenToNegZDouble(pos: NegZFiniteFloat): NegZDouble = NegZDouble.ensuringValid(pos.value)
/**
* Implicit widening conversion from NegZFiniteFloat
to NegZFiniteDouble
.
*
* @param pos the NegZFiniteFloat
to widen
* @return the Float
value underlying the specified NegZFiniteFloat
,
* widened to Double
and wrapped in a NegZFiniteDouble
.
*/
implicit def widenToNegZFiniteDouble(pos: NegZFiniteFloat): NegZFiniteDouble = NegZFiniteDouble.ensuringValid(pos.value)
/**
* Implicit widening conversion from NegZFiniteFloat
to FiniteDouble
.
*
* @param pos the NegZFiniteFloat
to widen
* @return the Float
value underlying the specified NegZFiniteFloat
,
* widened to Double
and wrapped in a FiniteDouble
.
*/
implicit def widenToFiniteDouble(pos: NegZFiniteFloat): FiniteDouble = FiniteDouble.ensuringValid(pos.value)
/**
* Implicit Ordering instance.
*/
implicit val ordering: Ordering[NegZFiniteFloat] =
new Ordering[NegZFiniteFloat] {
def compare(x: NegZFiniteFloat, y: NegZFiniteFloat): Int = x.toFloat.compare(y)
}
}